Use of *args and **kwargs
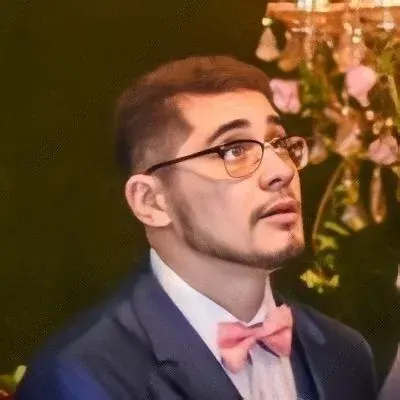
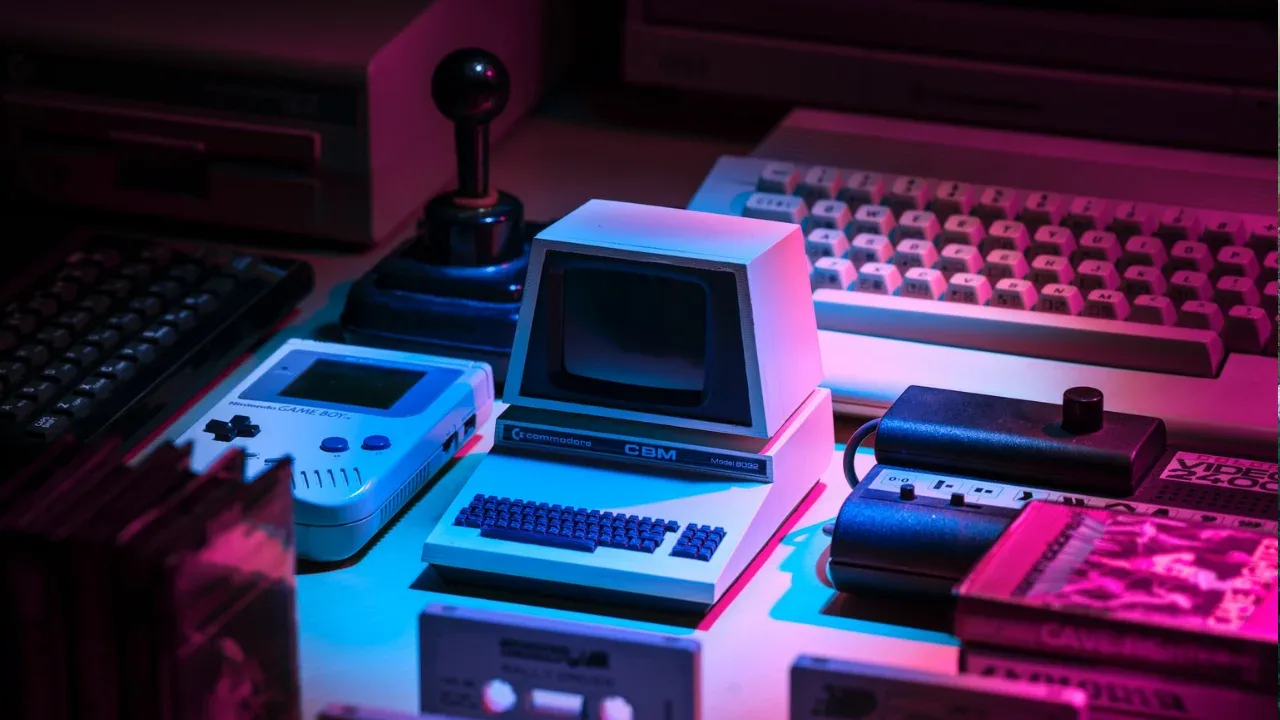
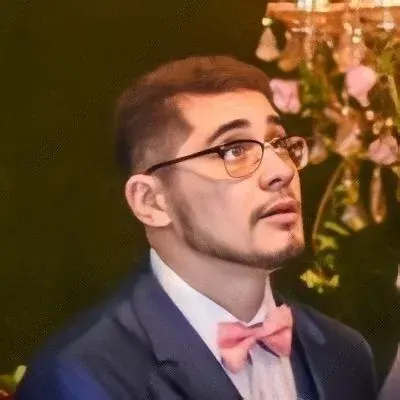
Demystifying *args and **kwargs in Python 🐍
If you've been scratching your head 🤔 trying to understand what *args and **kwargs are all about, you're not alone! Many programmers, especially beginners, struggle with grasping the concept of these magical constructs in Python. But fear not! In this blog post, we'll demystify *args and **kwargs once and for all. Let's dive in! 💻
Understanding *args 🧐
Okay, let's start with *args. Think of args as your go-to tool when you want to pass a variable number of arguments to a function. The "args" part is just a naming convention, so feel free to use any other name that makes sense to you. But make sure to keep that single asterisk () in front of it!
Here's the deal: When you use *args in a function definition, it allows you to pass any number of positional arguments. These arguments get stored as a tuple 📦 inside the function, so you can iterate over them or perform any other operations you like.
def calculate_total(*args):
total = 0
for num in args:
total += num
return total
total_sum = calculate_total(1, 2, 3, 4, 5)
print(total_sum) # Output: 15
In the example above, we define a function called calculate_total
that takes in any number of arguments using the *args parameter. Inside the function, we can treat *args as a regular tuple and perform calculations accordingly. In this case, we simply add up all the values and return the total.
Decoding **kwargs 😎
Now, let's move on to **kwargs. This one might seem a bit trickier at first, but don't worry, we'll break it down for you! Just like *args, kwargs is just a conventional name, and you can use any other name with the double asterisks ().
**kwargs allows you to pass a variable number of keyword arguments to a function. These arguments are then stored as a dictionary inside the function, with the keys representing the argument names and the values representing the corresponding values.
def greet(**kwargs):
for key, value in kwargs.items():
print(f"Hello {value}! Your {key} is awesome!")
greet(name="Alice", hobby="cooking", age=25)
Output:
Hello Alice! Your name is awesome!
Hello cooking! Your hobby is awesome!
Hello 25! Your age is awesome!
In this example, we define a function called greet
that accepts any number of keyword arguments using **kwargs. Inside the function, we iterate over the dictionary of arguments using the items()
method and print a customized message for each provided key-value pair.
When to use *args and **kwargs 🤷♂️
Now that we've covered the basics of *args and **kwargs, you might be wondering when to use them in your own code.
Here's a simple rule of thumb:
Use *args when you want to pass a variable number of positional arguments to a function.
Use **kwargs when you want to pass a variable number of keyword arguments to a function.
By using these constructs, you can make your code more flexible and reusable. Imagine needing to write a function that performs calculations on a list of numbers. With *args, you can pass any number of arguments without worrying about creating a fixed number of parameters. And with **kwargs, you can pass any number of named arguments without modifying your function's signature.
Wrapping it up 🎁
Congratulations! You've now unlocked the secrets of *args and **kwargs in Python! Armed with this knowledge, you'll be able to handle variable argument lists with ease and take your code to the next level.
So go ahead, give them a try in your own projects and let your creativity flourish! Just remember to use *args for positional arguments and **kwargs for keyword arguments, and you'll be all set. 🔥
Leave a comment below and let us know about your experiences with *args and **kwargs. Have you encountered any challenges or found innovative ways to use them? We'd love to hear from you! Happy coding! 💻💪