Use a list of values to select rows from a Pandas dataframe
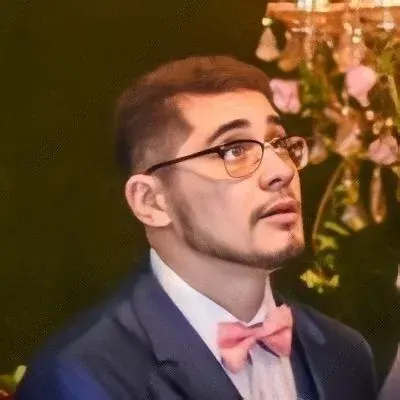
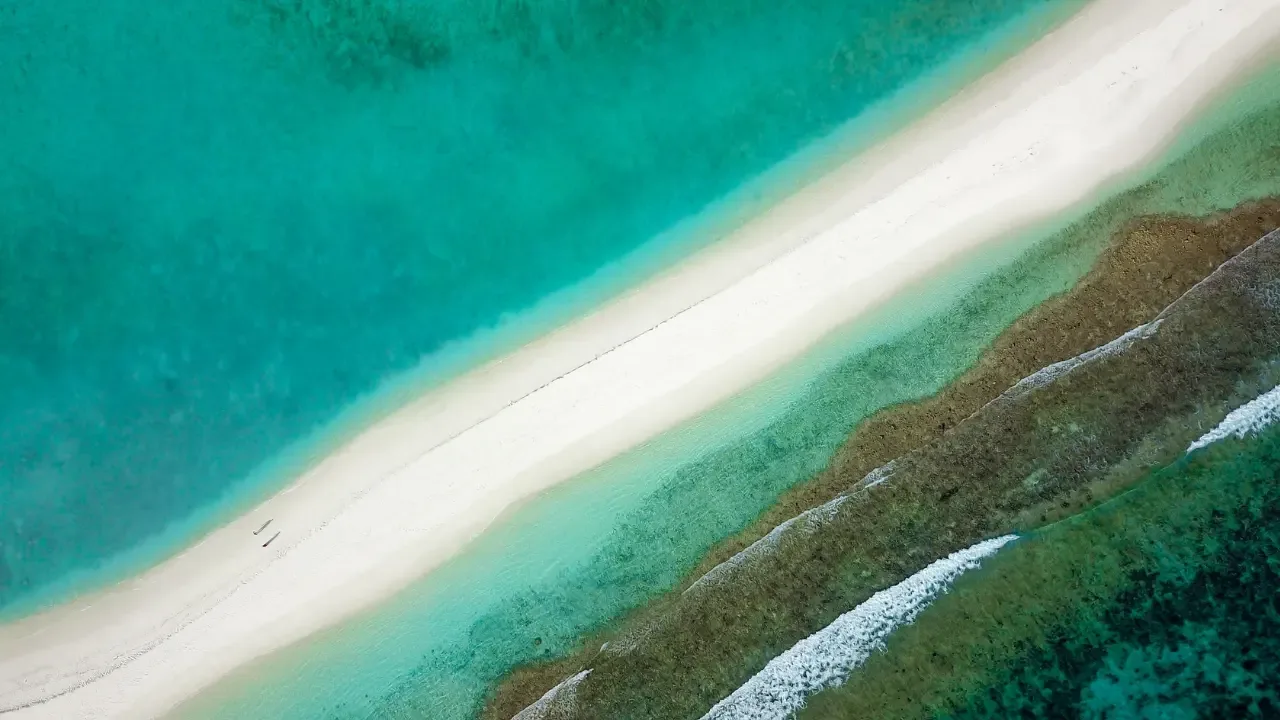
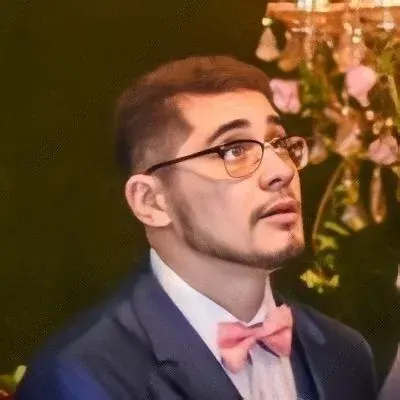
Using a List of Values to Select Rows from a Pandas Dataframe
So, you've got a Pandas dataframe, and you want to filter out specific rows based on a list of values. This can be a bit tricky if you're not familiar with the proper syntax. Don't worry, though β I'm here to help you out!
The Problem
Let's start with the problem at hand. Suppose you have the following Pandas dataframe:
df = DataFrame({'A': [5, 6, 3, 4], 'B': [1, 2, 3, 5]})
You want to subset the dataframe based on a list of values. In this example, let's say you want to select rows where the value in column 'A' is either 3 or 6.
The Solution
To achieve this, you might be tempted to use the in
operator in the following way:
list_of_values = [3, 6]
y = df[df['A'] in list_of_values]
However, this will result in a TypeError
because the in
operator cannot be used directly with a Pandas series.
The correct way to filter the dataframe based on a list of values is to use the isin()
method of the Pandas series.
list_of_values = [3, 6]
y = df[df['A'].isin(list_of_values)]
And voilΓ ! You will obtain the desired result:
A B
1 6 2
2 3 3
Explanation
Let's break down the solution to make sure you understand what's happening.
The expression
df['A']
returns a Pandas series representing the values in column 'A' of the dataframe.The
isin()
method of the series checks if each value in the series is contained in the list of values you provided. It returns a boolean series withTrue
for values that match andFalse
otherwise.By using this boolean series as an index for the dataframe
df
, you select only the rows where the corresponding value in column 'A' satisfies your condition.
Recap and Call-to-Action
To recap, when you want to filter a Pandas dataframe based on a list of values, make sure to use the isin()
method instead of the in
operator. This will save you from encountering any errors and help you select the desired rows.
Now that you know this handy trick, why not try it out on your own dataframes? If you have any questions or other Pandas-related topics you'd like to explore, leave a comment below and let's continue the conversation!
π Happy coding with Pandas! πΌπ»