Update a dataframe in pandas while iterating row by row
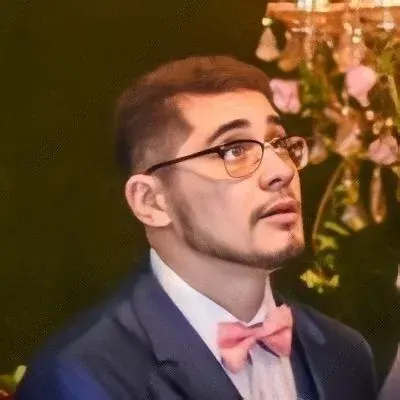
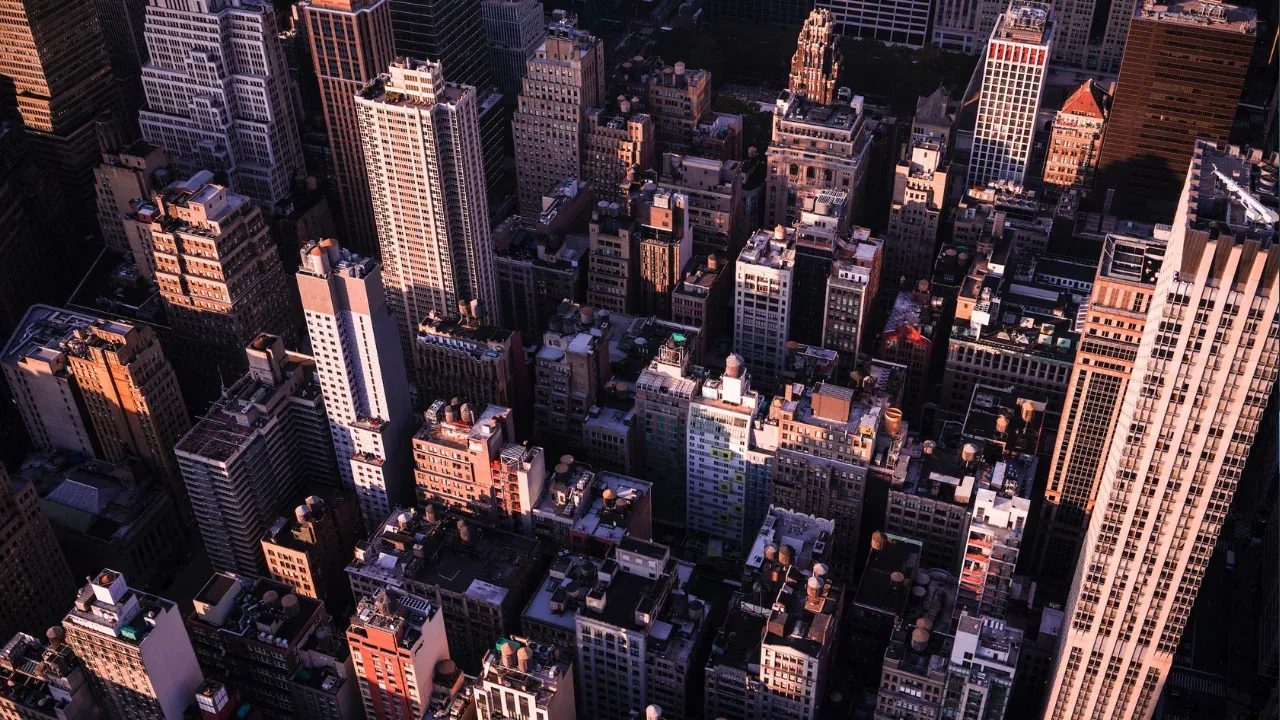
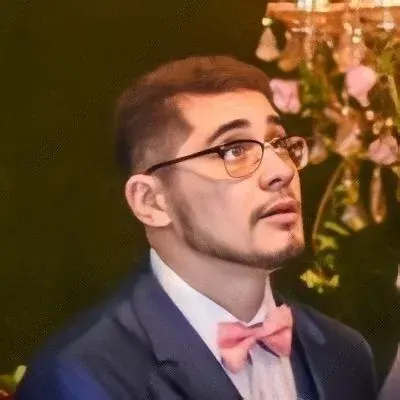
Updating a DataFrame in Pandas while Iterating Row by Row 🐼
So, you have a Pandas DataFrame and you want to update specific values in your DataFrame as you iterate row by row. You've tried a few approaches, but nothing seems to be working. Don't worry, I've got you covered! In this blog post, I'll show you how to update a DataFrame in Pandas while iterating row by row with easy-to-understand solutions. Let's dive in! 💻📊
The Problem 🤔
Let's first understand the problem you're facing. You have a large DataFrame that looks something like this:
date exer exp ifor mat
1092 2014-03-17 American M 528.205 2014-04-19
1093 2014-03-17 American M 528.205 2014-04-19
1094 2014-03-17 American M 528.205 2014-04-19
1095 2014-03-17 American M 528.205 2014-04-19
1096 2014-03-17 American M 528.205 2014-05-17
You want to iterate through each row and update the value of the ifor
column based on certain conditions and a lookup in another DataFrame. However, your current attempts to update the values are not yielding the desired results. 😫
The Solution 💡
To update a DataFrame while iterating row by row, you need to use the .loc
accessor to assign new values to specific locations in the DataFrame. Here's how you can achieve this:
for i, row in df.iterrows():
if <something>:
df.loc[i, 'ifor'] = x
else:
df.loc[i, 'ifor'] = y
In the above code snippet, we are iterating through each row using the iterrows()
function. For each row, we check the condition using <something>
. If the condition is met, we update the value in the ifor
column for that specific row using df.loc[i, 'ifor'] = x
. Otherwise, we update it with y
.
Example Usage 🚀
Let's see an example to better understand how this solution works. Suppose we have a DataFrame df
with the following initial values:
import pandas as pd
data = {
'date': ['2014-03-17', '2014-03-17', '2014-03-17', '2014-03-17', '2014-03-17'],
'exer': ['American', 'American', 'American', 'American', 'American'],
'exp': ['M', 'M', 'M', 'M', 'M'],
'ifor': [528.205, 528.205, 528.205, 528.205, 528.205],
'mat': ['2014-04-19', '2014-04-19', '2014-04-19', '2014-04-19', '2014-05-17']
}
df = pd.DataFrame(data)
Now, let's update the ifor
column based on a condition:
for i, row in df.iterrows():
if row['exer'] == 'American':
df.loc[i, 'ifor'] = 1000
else:
df.loc[i, 'ifor'] = 500
After executing the code above, the updated DataFrame df
will look like this:
date exer exp ifor mat
1092 2014-03-17 American M 1000.0 2014-04-19
1093 2014-03-17 American M 1000.0 2014-04-19
1094 2014-03-17 American M 1000.0 2014-04-19
1095 2014-03-17 American M 1000.0 2014-04-19
1096 2014-03-17 American M 1000.0 2014-05-17
As you can see, the values in the ifor
column have been successfully updated based on the condition.
Wrapping Up 📝
Updating a DataFrame in Pandas while iterating row by row can be achieved using the .loc
accessor to assign new values to specific locations in the DataFrame. Remember to use the .loc[i, 'column_name']
syntax to update a specific cell's value. I hope this guide has provided you with easy-to-understand solutions to solve your problem.
Now it's your turn to try it out! Feel free to experiment with your own conditions and logic to update the DataFrame dynamically. Happy coding! 💻✨