UnicodeEncodeError: "ascii" codec can"t encode character u"\xa0" in position 20: ordinal not in range(128)
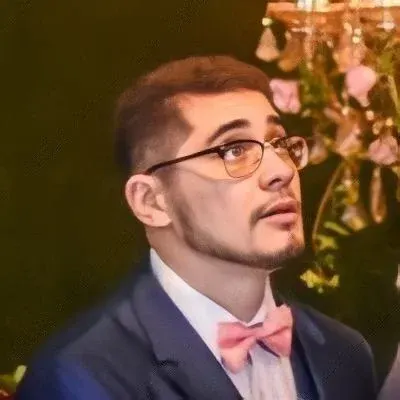
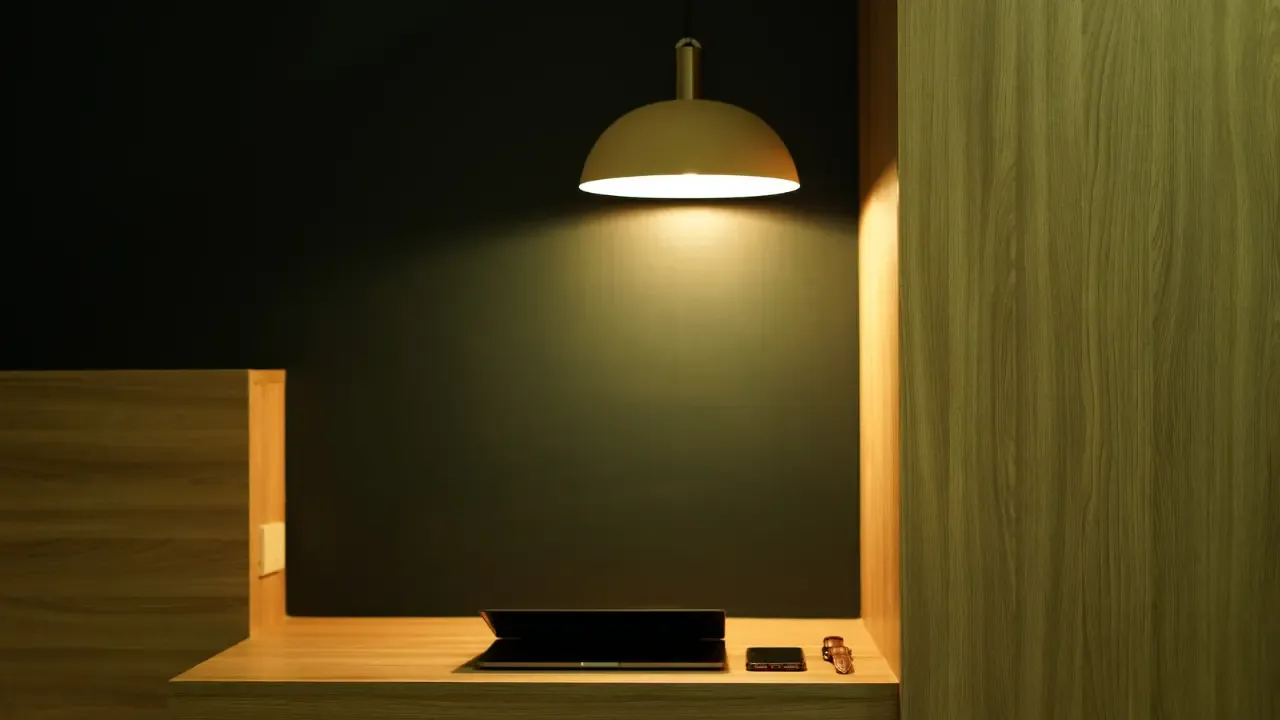
How to Fix the UnicodeEncodeError: 'ascii' codec can't encode character
Have you ever encountered the dreaded UnicodeEncodeError
when dealing with unicode characters in your Python code? It can be quite frustrating, especially when the error seems to appear sporadically and is hard to reproduce.
In this blog post, we will address the common issue of UnicodeEncodeError
and provide you with easy solutions to fix it consistently. Great news: you don't need to be an expert in Unicode encoding to solve this problem! 😊
Understanding the Problem
The UnicodeEncodeError
occurs when you try to encode a Unicode string using the ASCII codec, but the specific character you're trying to encode is not in the range of ASCII characters (i.e., characters with ordinal values less than 128).
In the context of your question, you mentioned that you are using BeautifulSoup to fetch text from different web pages. The error occurs in the following code snippet:
agent_telno = agent.find('div', 'agent_contact_number')
agent_telno = '' if agent_telno is None else agent_telno.contents[0]
p.agent_info = str(agent_contact + ' ' + agent_telno).strip()
The issue arises when trying to concatenate the agent_contact
and agent_telno
variables, and then convert them to a string. If either of these variables contains a character that is not ASCII-compatible, the UnicodeEncodeError
will be raised.
Solutions to Fix the Problem
Now that we understand the problem, let's explore a few solutions to tackle the UnicodeEncodeError
consistently:
1. Use Unicode strings throughout your code
By using Unicode strings (prefixed with u
) instead of regular strings, Python will automatically handle the encoding for you. Update the code snippet to:
agent_telno = agent.find('div', 'agent_contact_number')
agent_telno = '' if agent_telno is None else agent_telno.contents[0]
p.agent_info = u' '.join([agent_contact, agent_telno]).strip()
This change ensures that the agent_info
variable is always a Unicode string.
2. Specify the encoding explicitly
If you know the encoding of the text you're working with, explicitly decode it using the specified encoding. For example, if the encoding is UTF-8, modify the code as follows:
agent_telno = agent.find('div', 'agent_contact_number')
agent_telno = '' if agent_telno is None else agent_telno.contents[0]
p.agent_info = (agent_contact + ' ' + agent_telno).encode('utf-8').strip()
By encoding the string with the appropriate encoding, you can avoid the UnicodeEncodeError
.
3. Ignore or replace non-ASCII characters
Depending on your specific use case, you might decide to ignore or replace the non-ASCII characters altogether. You can achieve this by using the errors
parameter in the encode
method. Here's an example:
agent_telno = agent.find('div', 'agent_contact_number')
agent_telno = '' if agent_telno is None else agent_telno.contents[0]
p.agent_info = (agent_contact + ' ' + agent_telno).encode('ascii', 'ignore').strip()
In this case, the ignore
option tells Python to ignore any non-ASCII characters instead of raising an error.
Consistently Fix the Problem
With these solutions in place, you should be able to consistently fix the UnicodeEncodeError
when dealing with unicode characters in your code. 🎉
Remember, the solution you choose depends on your specific use case and the requirements of your project. Ensure that you understand the implications of each approach before implementing it in your code.
If you have any other ideas or solutions for this problem, please share them with us in the comments! Let's help each other overcome this challenge together.
Keep coding and embracing the beauty of Unicode! ✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
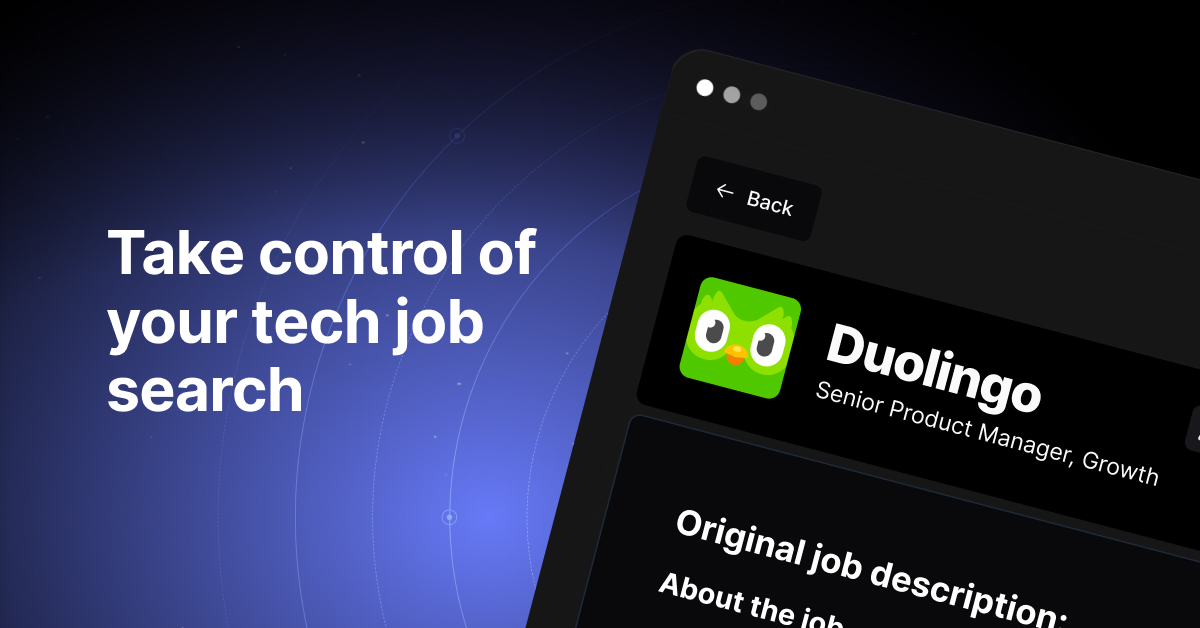