"Unicode Error "unicodeescape" codec can"t decode bytes... Cannot open text files in Python 3
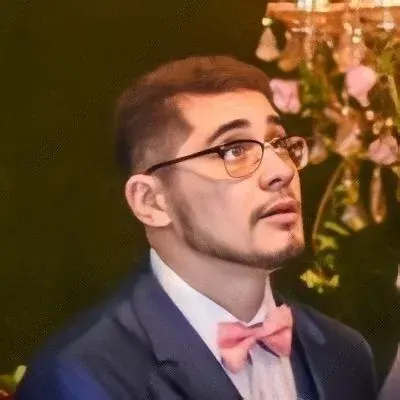
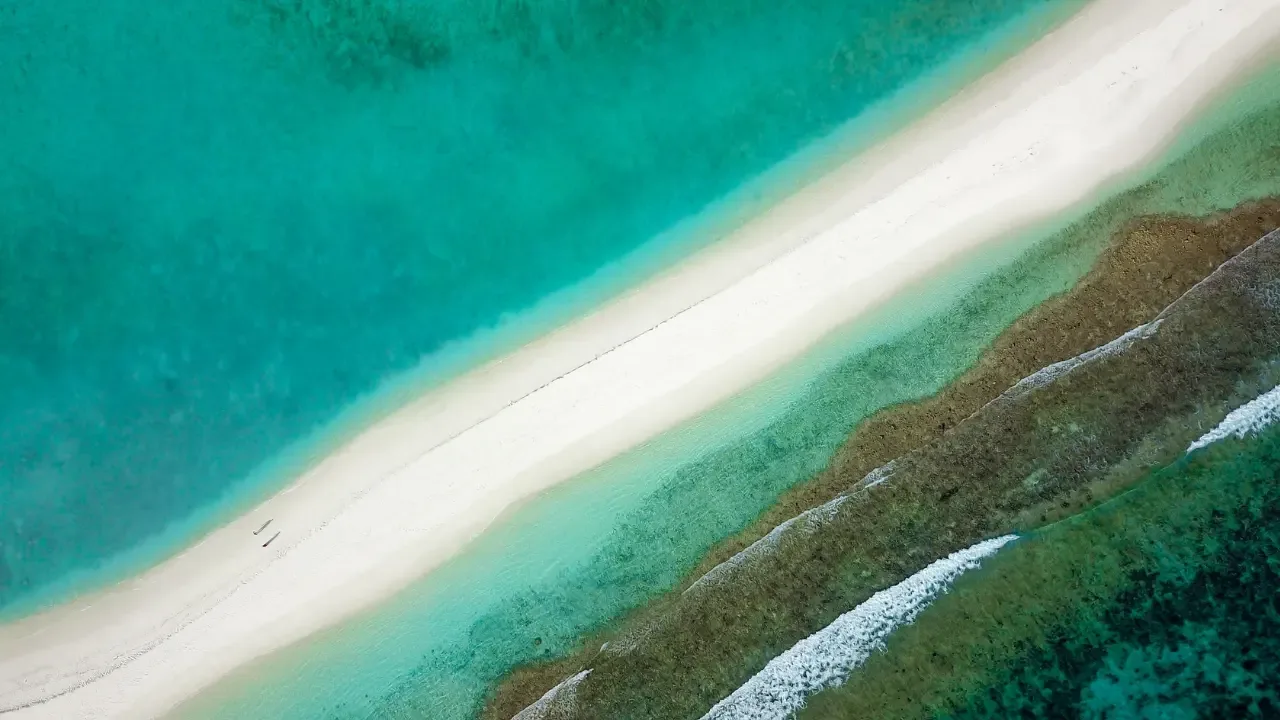
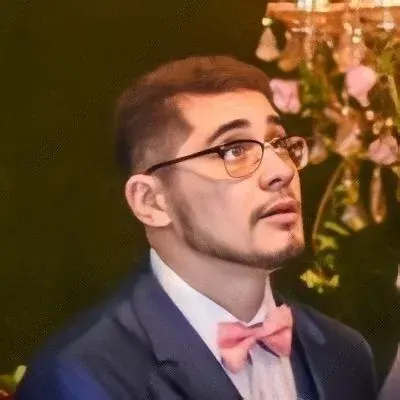
Unicode Error "unicodeescape" codec can't decode bytes... Cannot open text files in Python 3
Are you facing a Unicode error when trying to open a text file in Python 3? 😕
Don't worry, you're not alone! Many developers have encountered this frustrating issue. But fear not, because I'm here to help you understand the problem and provide you with easy solutions. 💪
Understanding the Problem
The error message you're seeing is related to Unicode decoding. Python 3 treats strings as Unicode by default. So when you try to open a text file with non-ASCII characters (such as Russian characters in your case) using the codecs.open()
function, you might encounter the "unicodeescape" codec error.
This error occurs because the file path you provided contains escape sequences that Python tries to decode. In Python, escape sequences start with a backslash and are used to represent special characters. However, in this case, the file path itself is being treated as an escape sequence.
Common Issues
Let's analyze the examples you provided to better understand the common issues and errors that can arise:
g = codecs.open("C:\Users\Eric\Desktop\beeline.txt", "r", encoding="utf-8")
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-4: truncated \UXXXXXXXX escape
In this example, the backslashes in the file path are being interpreted as escape sequences, resulting in a SyntaxError
. Python expects a valid Unicode escape sequence (starting with \U
followed by 8 hexadecimal digits). Since the file path does not follow this format, the error occurs.
g = codecs.open("C:\Users\Eric\Desktop\Site.txt", "r", encoding="utf-8")
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-4: truncated \UXXXXXXXX escape
Here, we encounter the same error as before. The backslashes in the file path are causing the issue.
g = codecs.open("C:\Python31\Notes.txt", "r", encoding="utf-8")
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 11-12: malformed \N character escape
In this case, the backslash followed by the letter 'N' in the file path is treated as a Unicode character. However, it is not a valid Unicode escape sequence, leading to a SyntaxError
.
Easy Solutions
Now that we understand the problem, let's explore some easy solutions.
Solution 1: Use Raw String
One way to fix the issue is by using a raw string. In Python, a raw string is prefixed with r
and treats backslashes as literal characters. By using a raw string, you can ensure that the file path is interpreted correctly.
g = codecs.open(r"C:\Users\Eric\Desktop\beeline.txt", "r", encoding="utf-8")
Simply add the r
prefix before the string containing the file path, and the error should be resolved.
Solution 2: Double Backslashes
Another solution is to double the backslashes in the file path. This ensures that the backslashes are treated as literal characters and not escape sequences.
g = codecs.open("C:\\Users\\Eric\\Desktop\\beeline.txt", "r", encoding="utf-8")
By using two backslashes instead of one, Python will correctly interpret the file path and open the text file without any Unicode errors.
Solution 3: Use Forward Slashes
Alternatively, you can use forward slashes instead of backslashes in the file path. Forward slashes are treated as regular characters in Python and are not used as escape sequences.
g = codecs.open("C:/Users/Eric/Desktop/beeline.txt", "r", encoding="utf-8")
By making this simple change, you can successfully open the text file without encountering any Unicode errors.
Conclusion
Unicode errors when opening text files in Python 3 can be frustrating, especially when working with non-ASCII characters. However, by understanding the problem and applying the easy solutions I've provided, you can overcome these errors and continue working with text files seamlessly. 😀
Remember, when dealing with file paths in Python, use raw strings, double backslashes, or forward slashes to avoid the "unicodeescape" codec error.
If you found this guide helpful or have any other questions, feel free to leave a comment below. Happy coding! 🚀