Understanding Python super() with __init__() methods
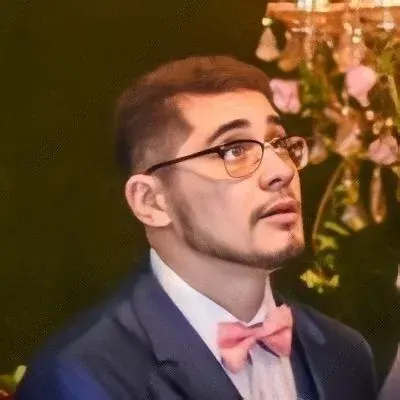
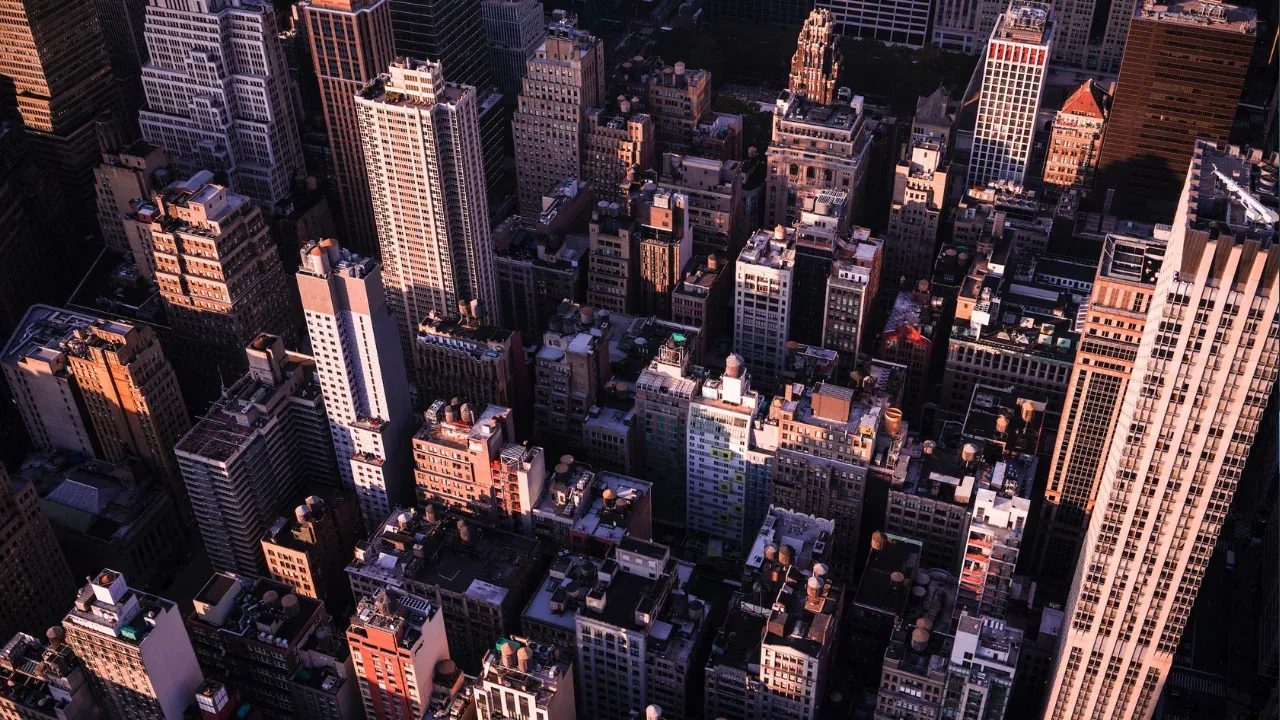
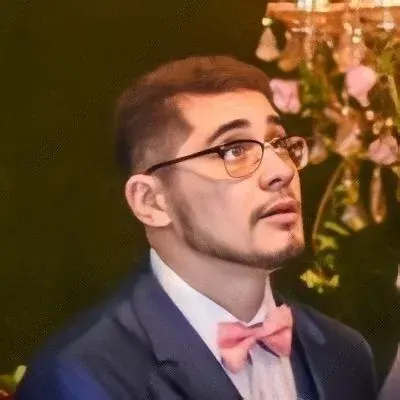
Understanding Python super()
with __init__()
methods 🐍👨💻
Do you often find yourself confused when it comes to using super()
in Python's __init__()
methods? 🤔
Well, fear not! In this blog post, we'll dive deep into the world of super()
and demystify its usage for you. By the end, you'll be confidently wielding the power of super()
like a Python pro! 💪💻
Why is super()
used? 🤷♀️
In Python, super()
is used to call the __init__()
method of the superclass (or parent class) in a subclass. This allows the subclass to inherit and utilize the initialization logic defined in the superclass. 📚🔀
Is there a difference between using Base.__init__
and super().__init__
? 🤔
Yes, there is! Let's take a look at the code snippet below to understand the difference:
class Base(object):
def __init__(self):
print("Base created")
class ChildA(Base):
def __init__(self):
Base.__init__(self)
class ChildB(Base):
def __init__(self):
super(ChildB, self).__init__()
ChildA()
ChildB()
In the code above, both ChildA
and ChildB
inherit from Base
and have their own __init__()
methods. However, they use different ways to call the superclass's __init__()
method.
🚀 When ChildA
uses Base.__init__(self)
, it directly invokes the __init__()
method of the Base
class. This is known as explicitly calling the superclass's method.
🌟 On the other hand, ChildB
uses super(ChildB, self).__init__()
, which utilizes the power of super()
. This is known as calling the superclass's method using the super()
function.
The magic of super()
🔮
Using super()
with __init__()
methods brings some nifty advantages:
1️⃣ Dynamic inheritance: When using super()
, you don't have to hardcode the superclass's name like Base.__init__(self)
. Instead, super()
figures it out dynamically based on the class it's called from. This means you can easily change the inheritance structure without having to update all the references to the superclass.
For example, if we decided to change the inheritance of ChildB
from Base
to another class named Parent
, we could do it with a single change in the class ChildB
definition:
class ChildB(Parent):
def __init__(self):
super().__init__() # No need to update the superclass name!
2️⃣ Support for multiple inheritance: super()
shines even brighter when dealing with multiple inheritance. It automatically handles the complexities of calling the right superclass's method in the method resolution order (MRO). This ensures that all the inherited classes' __init__()
methods are invoked correctly.
Easy solutions to common problems 💡
Problem: MRO-related confusion 🤯
Sometimes, when dealing with complex inheritance structures, you might encounter unexpected results due to the method resolution order (MRO).
Solution 👉 Use super()
consistently throughout your codebase. This ensures a consistent MRO and prevents any surprises. 🎉
Problem: Passing arguments to the superclass's __init__()
method 🎁
What if the superclass's __init__()
method requires additional arguments?
Solution 👉 You can simply pass the required arguments to the super().__init__()
call in the subclass's __init__()
method. For example:
class Base(object):
def __init__(self, name):
self.name = name
class ChildA(Base):
def __init__(self, name, age):
super().__init__(name)
self.age = age
child = ChildA("Alice", 25)
print(child.name) # Output: Alice
print(child.age) # Output: 25
Empower your Python code with super()
! 💪
Now that you have a solid understanding of super()
with __init__()
methods, go forth and wield its power in your Python code! 🚀
Remember to use super()
consistently, embrace multiple inheritance with confidence, and pass the required arguments to the superclass's __init__()
method when needed.
If you found this post helpful, share it with your fellow Python enthusiasts and let us know what other Python topics you'd like us to explore next! ❤️🐍
Keep coding and happy Python-ing! 👨💻✨