Understand Python swapping: why is a, b = b, a not always equivalent to b, a = a, b?
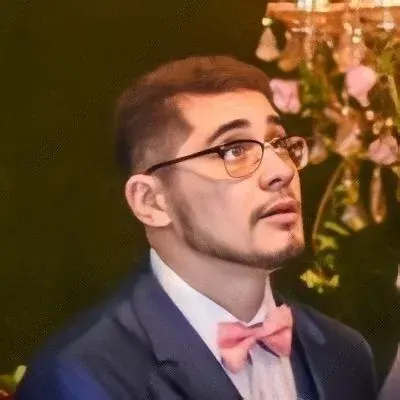
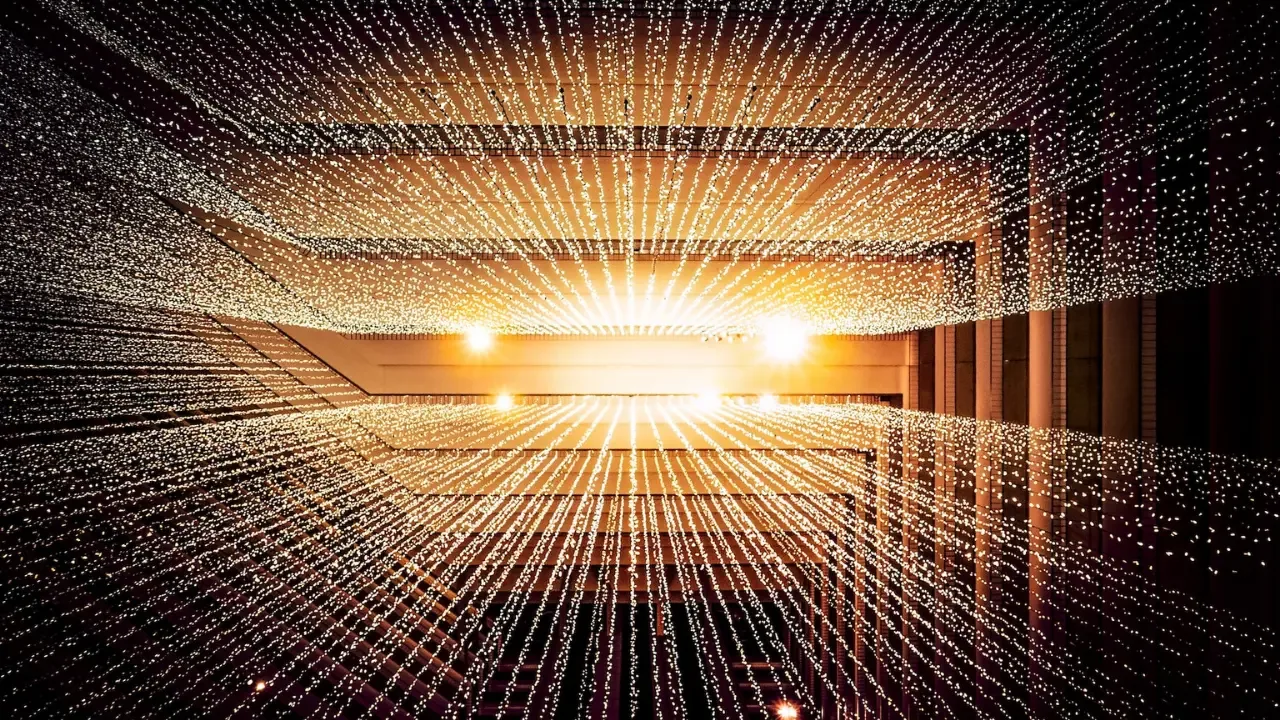
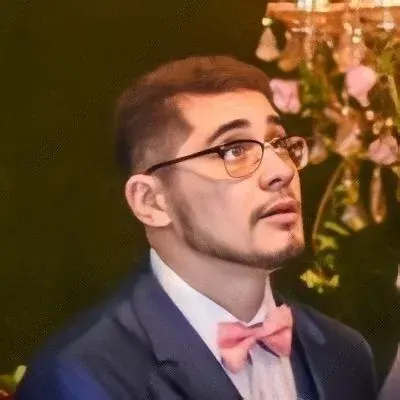
Understanding Python Swapping: Why is a, b = b, a
Not Always Equivalent to b, a = a, b
?
Are you an avid Python programmer who loves exploring the intricacies of the language? If so, you might have come across a puzzling scenario while swapping values between two variables. You may have noticed that swapping a
and b
using the popular method a, b = b, a
sometimes doesn't yield the same result as b, a = a, b
. 😲
In this blog post, we'll dive deep into this phenomenon, unravel the mystery behind it, provide simple solutions to avoid such issues, and empower you to write robust Python code. Let's get started! 🚀
The Pythonic Swap
The Python community loves elegant and concise code, and the commonly used method to swap the values of two variables is a, b = b, a
. It's simple, readable, and widely recommended. For instance, consider the following code snippet:
a = 1
b = 2
a, b = b, a
print(a, b)
# Output: 2, 1
As expected, the values of a
and b
get swapped successfully, and the output is as desired.
The Unexpected Twist
However, there may be cases where this swapping magic doesn't work as expected. Let's explore an example to understand this concept better:
nums = [1, 2, 4, 3]
i = 2
nums[i], nums[nums[i]-1] = nums[nums[i]-1], nums[i]
print(nums)
# Output: [1, 2, 4, 3]
nums = [1, 2, 4, 3]
i = 2
nums[nums[i]-1], nums[i] = nums[i], nums[nums[i]-1]
print(nums)
# Output: [1, 2, 3, 4]
Surprising, isn't it? In the first scenario, where we use nums[i], nums[nums[i]-1] = nums[nums[i]-1], nums[i]
, the values in the nums
list remain the same. However, when we swap the values using nums[nums[i]-1], nums[i] = nums[i], nums[nums[i]-1]
, the swap occurs successfully.
The Explanation
The reason behind this discrepancy lies in the order of evaluation of expressions in Python. In Python, the expressions on the right side of the =
symbol are evaluated first before performing the assignments. In the case of a, b = b, a
, this doesn't matter as both a
and b
are separate variables. But when swapping values within a data structure like a list, the order of evaluation plays a crucial role.
The Solution
To ensure consistent results and avoid confusion while swapping variables within lists or other data structures in Python, follow these guidelines:
Avoid using values computed from the left side of the assignment within the right side of the assignment. Instead, store these values in temporary variables before performing the swap.
Here's an updated version of the previous example using temporary variables:
nums = [1, 2, 4, 3]
i = 2
temp = nums[i]
nums[i], nums[temp-1] = nums[temp-1], nums[i]
print(nums)
# Output: [1, 2, 3, 4]
If you prefer a one-liner, consider using the
temp
variable within a list comprehension to achieve the desired swap:
nums = [1, 2, 4, 3]
i = 2
nums[:] = [nums[i] if x == nums[i] - 1 else nums[i] - 1 if x == i else x for x in nums]
print(nums)
# Output: [1, 2, 3, 4]
Empowering the Community
We hope this blog post helped you understand the subtle intricacies of swapping variables in Python and how a, b = b, a
might not always be equivalent to b, a = a, b
. The key takeaway is that the order of evaluation can impact swapping operations within data structures like lists.
If you found this blog post useful and want to learn more about Python or any other tech topics, be sure to follow our blog and engage with our community. Feel free to share your thoughts, examples, or concerns in the comments section below. Together, let's unravel the mysteries of the tech world! ✨