TypeError: only integer scalar arrays can be converted to a scalar index with 1D numpy indices array
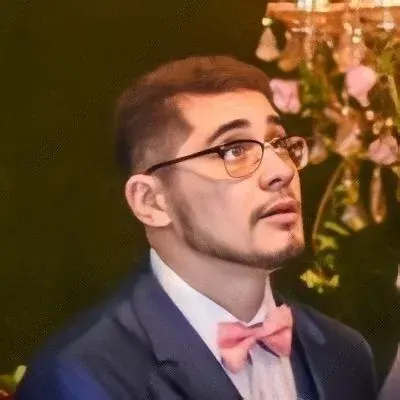
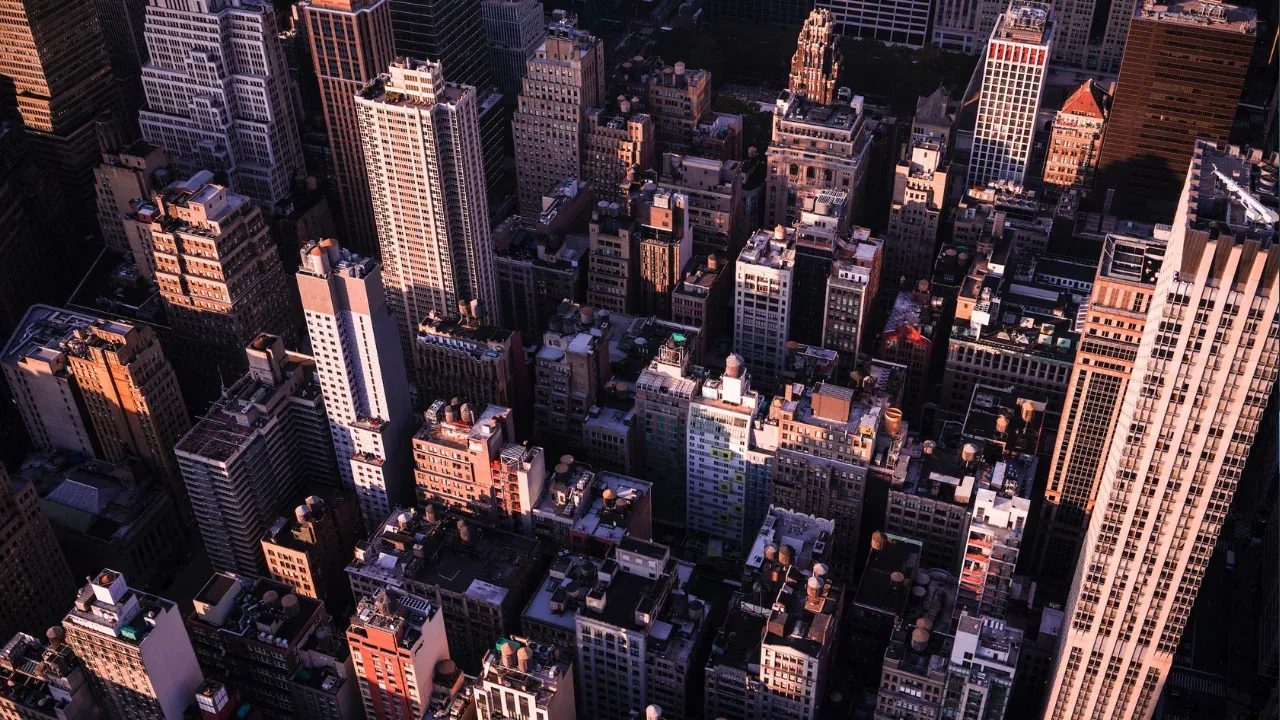
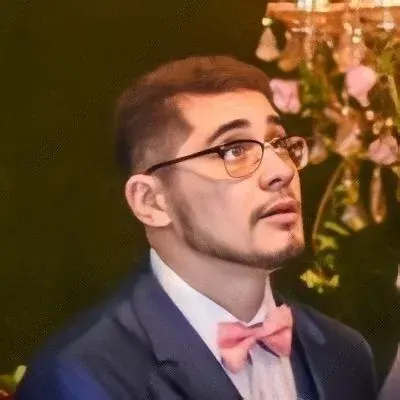
š Blog Post: How to Fix the "TypeError: only integer scalar arrays can be converted to a scalar index with 1D numpy indices array" Error in Python
š Introduction
Are you struggling with the "TypeError: only integer scalar arrays can be converted to a scalar index with 1D numpy indices array" error in Python? Don't worry, you're not alone! In this blog post, we'll dive into the common issues behind this error and provide you with easy solutions to fix it. Whether you're a beginner or an experienced Python developer, this guide will help you understand and resolve this problem efficiently.
š” Understanding the Problem
The error message you encountered is a bit puzzling, especially when you've already confirmed that your array is 1-D, integer, and scalar. So, what's causing the issue?
In your specific example, the error occurs when you're trying to access element(s) from the X_train
array using the indices
array. Let's look at the code snippet causing the error:
out_images = X_train[indices.astype(int)] # this is where I get the error
š Finding the Solution
Check Your Imports
Before we dive deeper into the issue, let's ensure that you've imported the necessary libraries correctly. In your case, you're using the NumPy library. Make sure you have imported it at the beginning of your code:
import numpy as np
Confirm Your Data Types
Although you mentioned that you already checked the data types, let's quickly go over it again. Ensure that both the X_train
array and the indices
array have the correct data type. You can verify this by printing their data types:
print(X_train.dtype) # Output: int
print(indices.dtype) # Output: int
Verify the Shape of Your Arrays
While the error message specifically mentions the data type, it's worth confirming that the shape of the arrays is also correct. Use the shape
attribute to validate the dimensions:
print(X_train.shape) # Output: (2000000,)
print(indices.shape) # Output: (50000,)
Make sure that the shapes align with your expectations. The X_train
array should have a shape of (2000000,)
and the indices
array should have a shape of (50000,)
.
Check the Array Values
Next, let's examine the values inside the arrays. Ensure that all the values in the indices
array are valid and within the range of the X_train
array. You can print the minimum and maximum values for both arrays:
print(np.min(indices))
print(np.max(indices))
print(np.min(X_train))
print(np.max(X_train))
Double-checking these values will help ensure that there are no accidental mistakes or inconsistencies causing the error.
Inspect the Calculation
In your code, you're using the np.random.choice
function to randomly select indices based on the provided probabilities. While the usage of this function looks fine, let's review the calculations leading up to it.
Specifically, look into the creation and normalization of the train_probs
array. Verify that these calculations produce the desired probabilities and that the length of train_probs
matches the length of X_train
:
print(len(X_train))
print(len(train_probs))
Ensure that both lengths are the same. If not, double-check your calculations to determine any discrepancies.
Try a Different Access Method
If you've followed the previous steps and the error persists, you can try an alternative method to access the elements from the X_train
array. Replace the problematic line of code with the following, and see if it resolves the issue:
out_images = np.take(X_train, indices.astype(int))
Updating NumPy Version
In rare cases, this error might be related to a bug in the NumPy library. Updating your NumPy version to the latest stable release could potentially address the issue. You can update NumPy using the following command:
pip install numpy --upgrade
š¬ Wrap Up and Engage with the Community
We've explored various solutions to fix the "TypeError: only integer scalar arrays can be converted to a scalar index with 1D numpy indices array" error. By following the steps outlined in this guide, you should be able to troubleshoot and resolve the problem.
If you have any other questions or encountered a different solution, please share them in the comments below. Let's learn and help each other overcome programming challenges! šŖ
Now, it's your turn! Have you ever faced this error? How did you solve it? Share your experiences with us, and let's create a vibrant conversation around this topic. š£ļøš¬
Remember, tech problems are opportunities for growth! Keep coding and stay curious! šāØ