TypeError: module.__init__() takes at most 2 arguments (3 given)
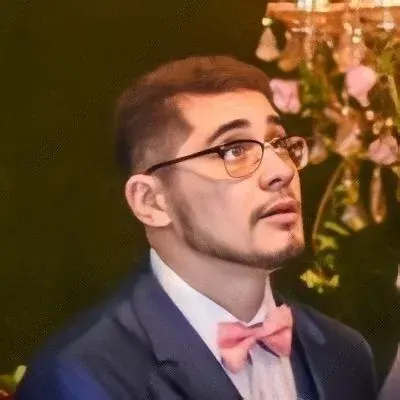
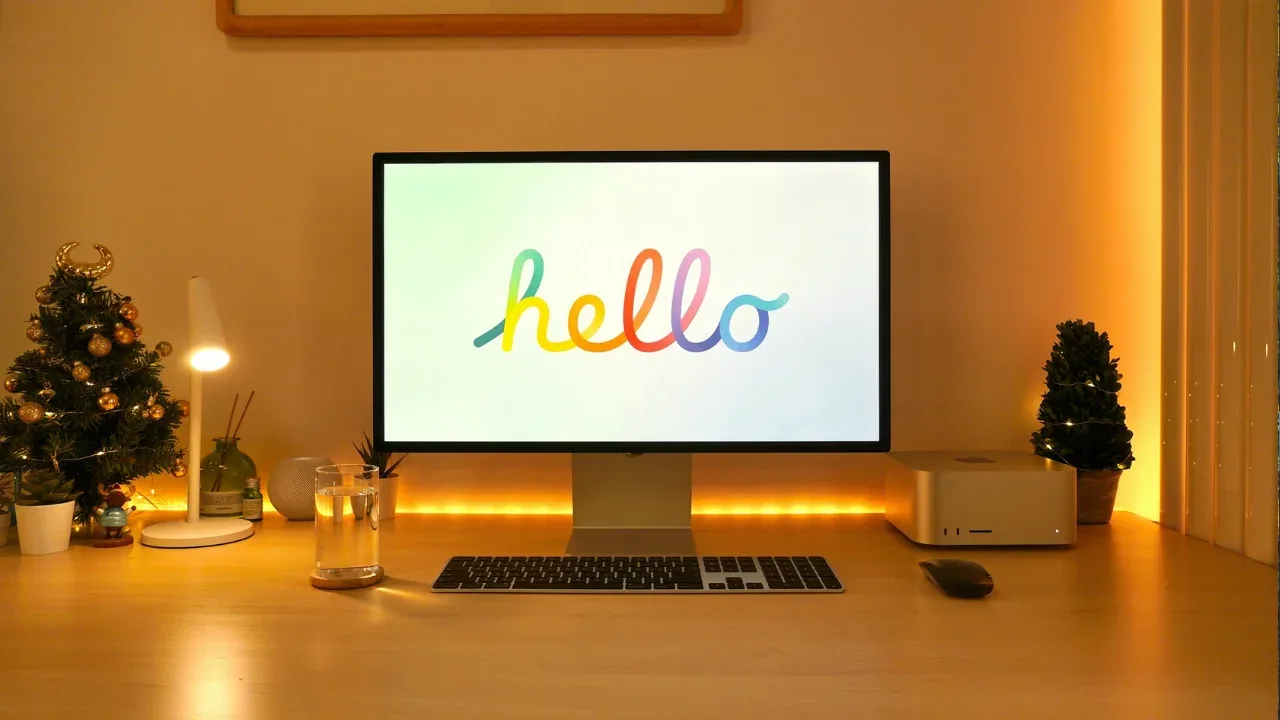
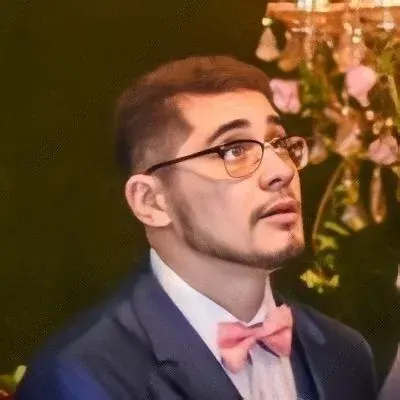
📝🔧🔀💻💥
Title: "Understanding and Fixing the TypeError: module.init() takes at most 2 arguments (3 given)"
Introduction: Hey there, tech enthusiasts! 👋 Are you facing a frustrating TypeError when trying to inherit from a class in Python? Fear not! 🦸 In this blog post, we'll walk you through the common issue of "TypeError: module.init() takes at most 2 arguments (3 given)" and provide you with easy-to-implement solutions. 🚀 So, let's dive right in and resolve this problem together!
Understanding the Context:
So, you've defined a class in a file named Object.py
, and when you try to inherit from this class in another file, calling the constructor throws the following exception:
TypeError: module.__init__() takes at most 2 arguments (3 given)
Accompanied by this code snippet:
import Object
class Visitor(Object):
pass
instance = Visitor() # this line throws the exception
Identifying the Issue:
The error message suggests that we're passing three arguments instead of the expected maximum of two to the constructor of the Object
module. So, what could be the reason for this discrepancy? Let's explore some possible causes and their solutions.
Mistake 1: Incorrect Import Statement It's possible that the import statement is causing the issue. Ensure that you're importing the class correctly from the
Object.py
file by using the following statement:from Object import Object
By explicitly importing the class, you'll eliminate any confusion regarding which module's constructor is being called.
Mistake 2: Accidental Module Initialization Another potential reason for this error is calling the constructor of the module itself by mistake. To fix this, make sure you're inheriting from the
Object
class without using parentheses:class Visitor(Object): pass
Using parentheses would inadvertently attempt to initialize the module instead of inheriting from the class.
Final Thoughts and Call-to-Action: Hooray! 🎉 You've successfully addressed the "TypeError: module.init() takes at most 2 arguments (3 given)" issue that was preventing you from inheriting from a class in Python. Now you can confidently proceed with your coding tasks. 🚀
If you've found this blog post helpful, feel free to share it with your fellow Python developers who might be dealing with similar problems. Additionally, if you have any questions or other tech-related topics you'd like us to cover, please leave a comment below. Let's keep the tech community thriving and learning together! 😊💻
Happy coding! 💡