TypeError: "dict_keys" object does not support indexing
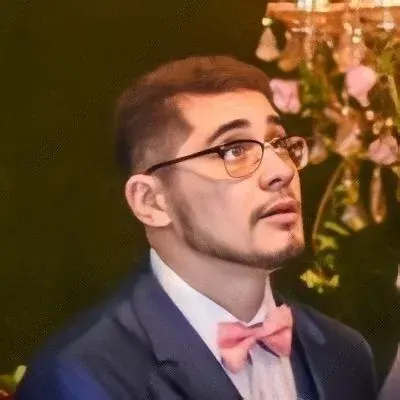
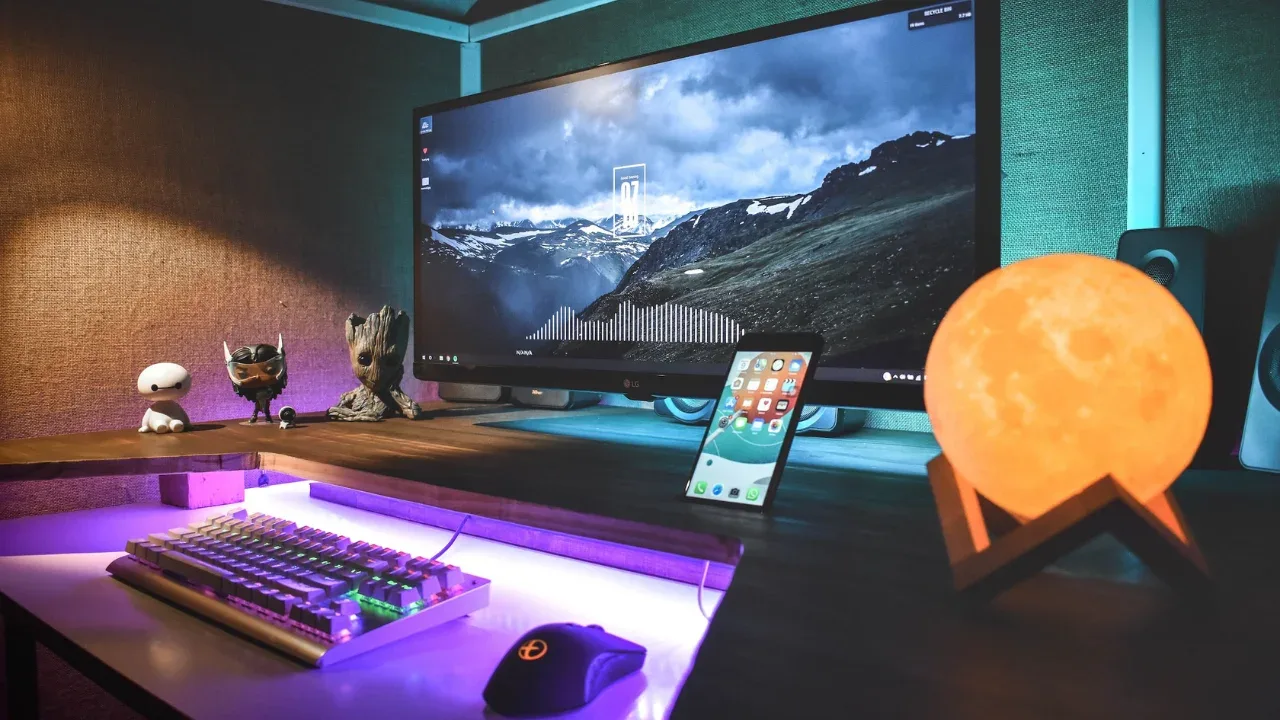
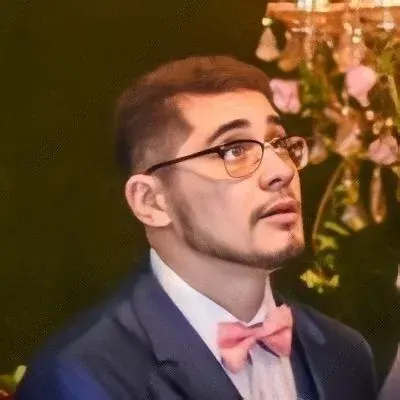
š Title: Solving the 'TypeError: 'dict_keys' object does not support indexing' Error
š Hello there, tech enthusiasts! Have you ever encountered a baffling error message like 'TypeError: 'dict_keys' object does not support indexing'
when running code? Don't worry, you're not alone! In this blog post, we'll dive into what causes this error, discuss common scenarios where it can occur, and most importantly, provide you with easy solutions to fix it. So, let's get started!
š Understanding the Error:
When you see the error message 'TypeError: 'dict_keys' object does not support indexing'
, it generally means that you're trying to perform an indexing operation on an object of the dict_keys
type. In Python, dict_keys
objects are returned when you access the keys of a dictionary using the keys()
method. These objects are iterable, similar to lists, but they do not support direct indexing.
š” Common Scenarios Where the Error Occurs:
The error often arises when users mistakenly try to treat a dict_keys
object as a list and attempt to access its elements using square bracket notation. In the provided context, it's possible that the variable x
being passed to the shuffle
function is not a list but a dict_keys
object instead. Let's dig deeper into the code snippet to understand why this is happening.
š Analyzing the Code:
In the given code snippet, the shuffle
function takes in a parameter x
and shuffles its elements in place. It uses a helper function _randbelow
and a loop to perform the shuffling. However, the error occurs when x
is expected to be a list, but it turns out to be a dict_keys
object instead.
š§ Fixing the Error:
To resolve the error, we need to ensure that the input passed to the shuffle
function is a list. Here are a few simple solutions:
Convert
dict_keys
to a list explicitly:x = list(x)
Here, the
list()
function is used to convert thedict_keys
object to a list.Pass a list directly to the
shuffle
function:my_list = list(x.keys()) # Convert dict_keys to a list shuffle(my_list)
Here, we create a list by passing
x.keys()
to thelist()
function, and then pass the resulting list to theshuffle
function.Modify the code to expect a
dict_keys
object:def shuffle(self, x, random=None, int=int): # Convert dict_keys to a list if required if isinstance(x, dict_keys): x = list(x) # Rest of the code
š Call-to-Action:
Now that you understand how to fix the 'TypeError: 'dict_keys' object does not support indexing'
error, it's time to put your newfound knowledge to use! If you've encountered this error before, let us know how you resolved it in the comments section below. If you found this blog post helpful, don't forget to share it with your fellow developers to simplify their debugging experience.
That's all for now, folks! Happy coding! š