"TypeError: a bytes-like object is required, not "str"" when handling file content in Python 3
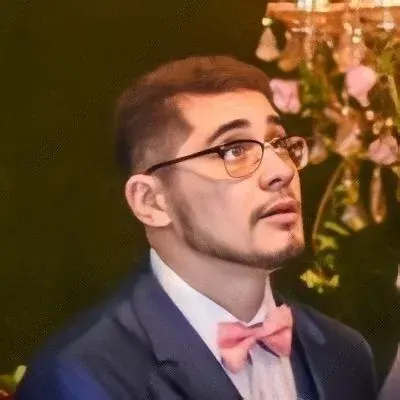
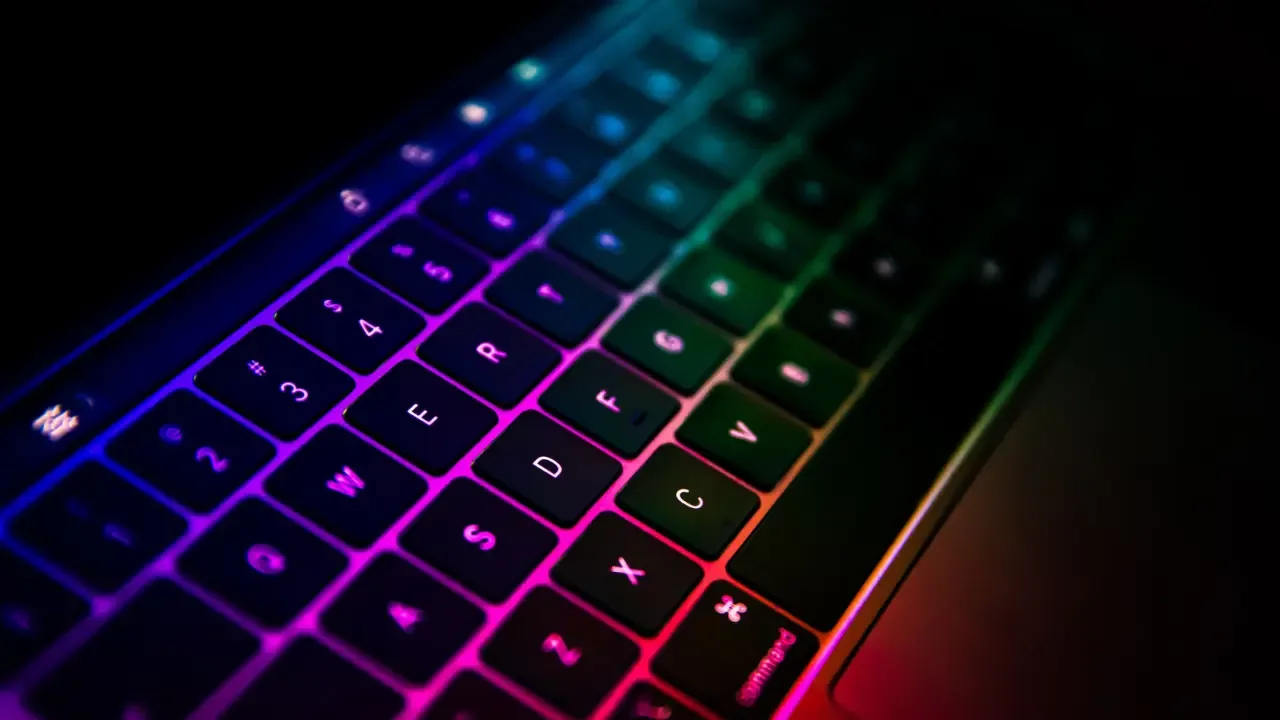
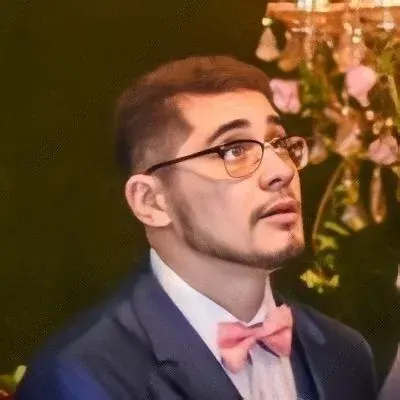
🐍🔥 Python 3 File Content Handling Error: TypeError 🚫❌
So, you've migrated to Python 3.5 like a boss 🎩✨, and suddenly you encounter the dreaded TypeError: a bytes-like object is required, not 'str'
💔😭. Fear not, my friend! I'm here to help you fix this problem and get your code working smoothly again 🙌🔧. Let's dive into it!
The Context 📚🔍
You provided a piece of code that was working fine in Python 2.7, but when you made the switch to Python 3.5, the trouble began. Here's a snippet of the code for reference:
with open(fname, 'rb') as f:
lines = [x.strip() for x in f.readlines()]
for line in lines:
tmp = line.strip().lower()
if 'some-pattern' in tmp: continue
# ... code
The issue arises specifically on the line if 'some-pattern' in tmp: continue
, and Python throws a TypeError
at you, saying a bytes-like object is required, not 'str'
😫.
Understanding the Problem 🕵️♀️🔎
The problem lies in how Python 3 handles file reading. In Python 3, the contents read from a file opened in binary mode ('rb'
) are of type bytes
, not str
like in Python 2. So when you strip and lower the line (tmp = line.strip().lower()
), it becomes a str
object. When you try to check if 'some-pattern'
is present in tmp
using the in
operator, Python expects a bytes-like object for comparison, resulting in a TypeError
.
The Solution 💡🛠
To fix this, we need to ensure that the search pattern ('some-pattern'
in this case) is also a bytes
object. Let's modify the code accordingly:
with open(fname, 'rb') as f:
lines = [x.strip() for x in f.readlines()]
for line in lines:
tmp = line.strip().lower()
if b'some-pattern' in tmp: continue
# ... code
By adding the b
prefix before 'some-pattern'
, we convert it into a bytes
object in Python 3, allowing it to be compared with tmp
without raising any TypeError
.
Final Thoughts 🤔💭
Congratulations, my friend! You've successfully fixed the TypeError
and adapted your code for Python 3. You're back on track to continue your Pythonic adventures 🚀🐍! Remember, in Python 3, handling file content may require you to be mindful of the byte-like nature of the data.
If you have any more Python-related questions or come across any further roadblocks, feel free to reach out! I'm always here to help you on your coding journey 🤗💻.
Stay curious, keep coding! 🌟👩💻
PS: If you found this article helpful, remember to share it with your fellow Pythonistas! Let's spread the Python love! 💙🐍
👉 Do you have any other Python questions or need help with coding in general? Contact me, and let's solve your tech problems together! 💪💥