Type hinting / annotation (PEP 484) for numpy.ndarray
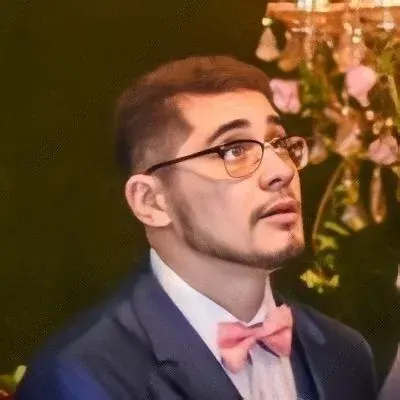
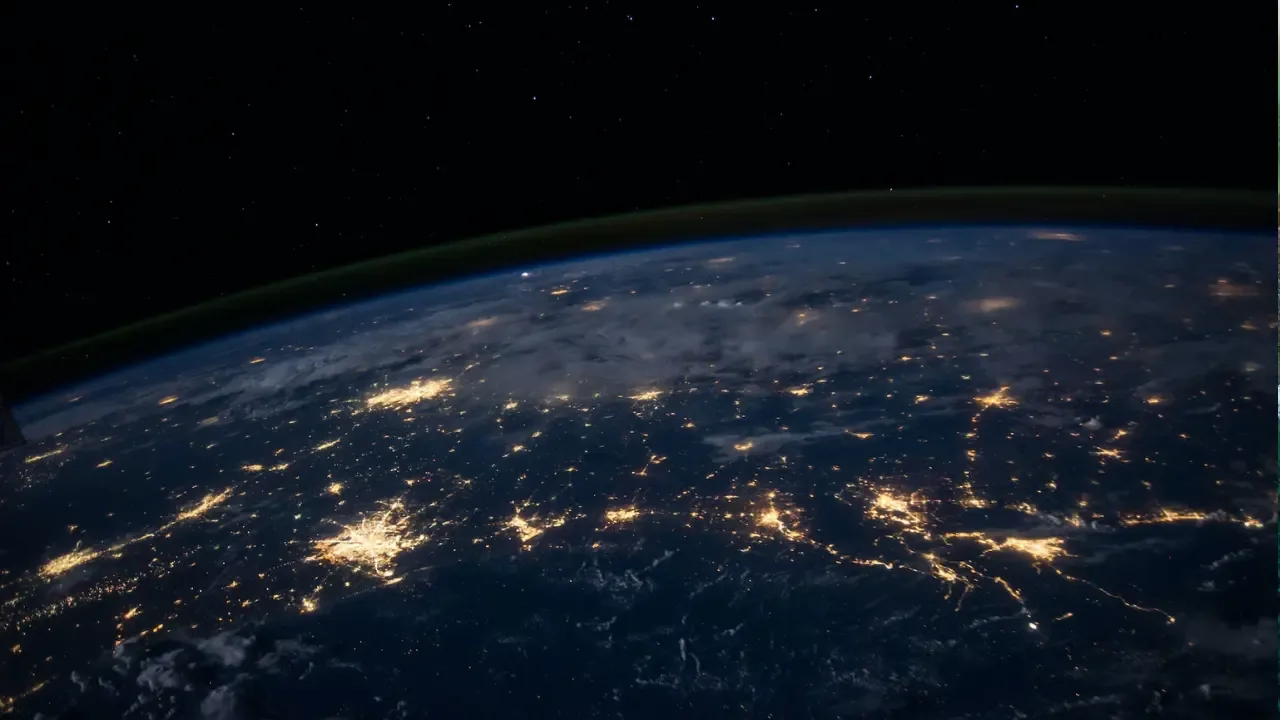
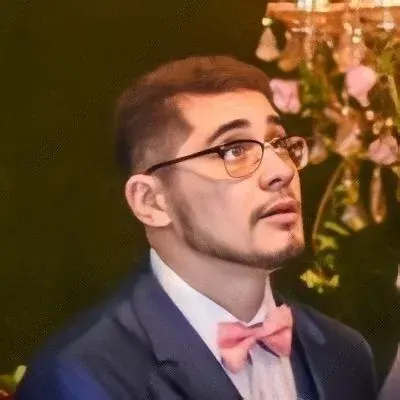
💡 Type Hinting/Annotation for numpy.ndarray: A Comprehensive Guide
Are you tired of using vague type hints like typing.Any
when working with the numpy.ndarray
class? Do you wish there was a more specific and accurate way to annotate your code? Well, you're in luck! In this blog post, we'll explore the concept of type hinting for numpy.ndarray
and provide easy solutions to help you enhance your code readability and maintainability. Let's dive in! 🏊♂️
Understanding the Problem
Currently, when it comes to type hinting the numpy.ndarray
class, there isn't a built-in solution. This can make it challenging to write clear and precise code, especially if you rely heavily on NumPy arrays. Thankfully, there are a couple of approaches we can take to address this issue. Let's explore them one by one.
Approach 1: Type Aliases
One potential solution to improve type hinting for numpy.ndarray
is through the use of type aliases. As mentioned in the initial question, it would be great if NumPy provided a predefined type alias for their array_like
object class. This would allow us to use a more specific type hint in our code.
Unfortunately, at the time of writing this post, NumPy has not officially implemented type aliases for their arrays. However, you can create your own type alias using Python's typing
module. Here's an example:
from typing import List, TypeVar
import numpy as np
NdArray = TypeVar('numpy.ndarray')
def process_data(data: NdArray) -> None:
# Process the NumPy array
pass
# Usage
my_data: NdArray = np.array([...])
process_data(my_data)
By defining NdArray
as a type alias for numpy.ndarray
, you can now use NdArray
as a type hint wherever you need to annotate a NumPy array in your code. While this solution requires a bit of extra work on your part, it significantly improves the clarity and expressiveness of your type hints. 🌟
Approach 2: Custom Type Hints
If you want to take type hinting for numpy.ndarray
to the next level, you can create your own custom type hints. This approach allows you to define specific types for different NumPy arrays based on their shape and dtype. Here's an example:
from typing import Type
import numpy as np
class IntVector(np.ndarray):
def __new__(cls, data):
obj = np.asarray(data).view(cls)
return obj
def __init__(self, data) -> None:
self.dtype = np.int64
self.shape = (len(data),)
In this example, we create a custom type IntVector
, which represents a NumPy array of integers with a 1-dimensional shape. You can define similar custom types for different kinds of arrays you frequently use in your code.
Now, let's see how we can use this custom type hint in our code:
def process_int_vector(vec: IntVector) -> None:
# Process the integer vector
pass
# Usage
my_vector: IntVector = IntVector([1, 2, 3, 4])
process_int_vector(my_vector)
By leveraging custom type hints, you can make your code more self-explanatory and reduce the chances of passing incorrect types to your functions. 💪
Call-to-Action: Your Feedback Matters! 📣
Type hinting for numpy.ndarray
is still a work in progress, and your feedback can make a huge difference. Get involved with the NumPy community, join relevant discussions, and express your interest in having better type hinting support. Together, we can enhance the development experience for all NumPy users. Let's make our voices heard! 🗣️
That's a wrap for this blog post. We hope you found this guide helpful in understanding and addressing the challenges of type hinting for numpy.ndarray
. Start implementing these solutions in your code and level up your Python programming skills. If you have any questions or thoughts to share, feel free to leave a comment below. Happy coding! 😄🚀