Type hinting a collection of a specified type
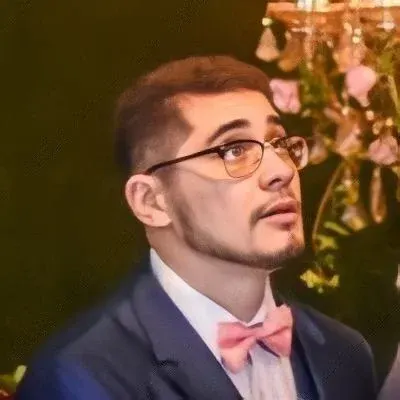
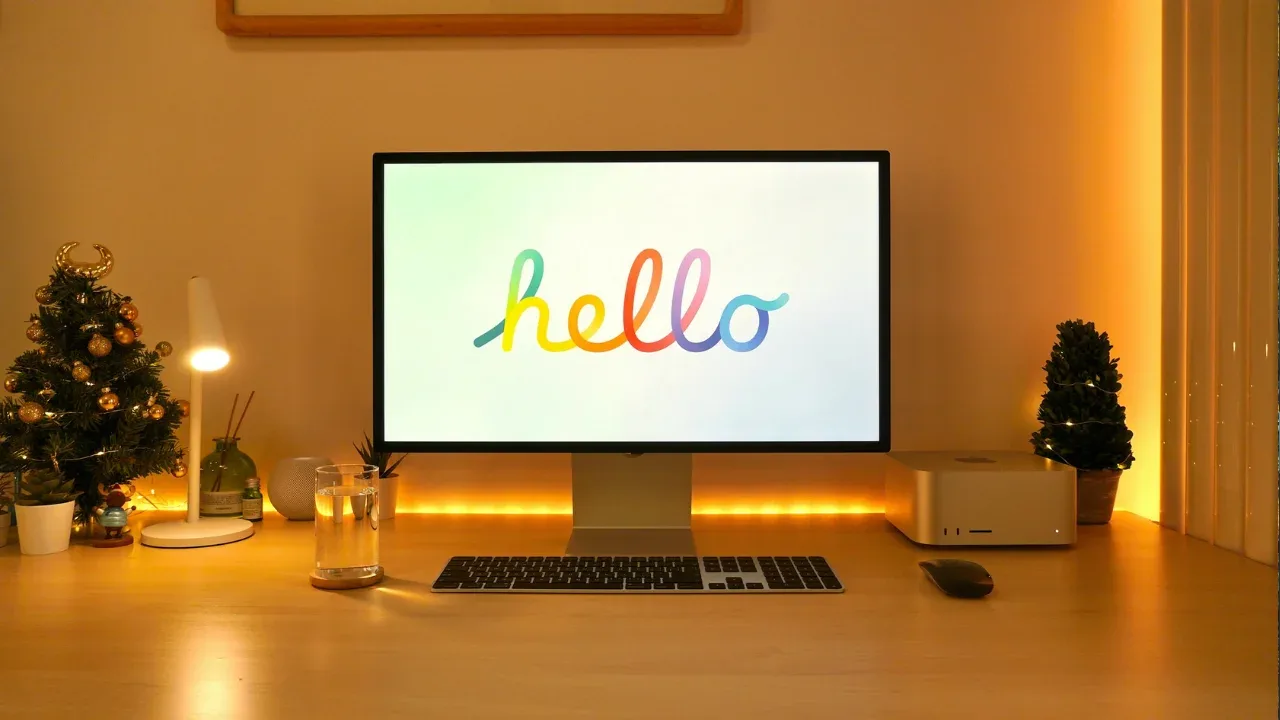
📝 Title: Type Hinting Collections in Python: Annotating Your Way to Clean Code! ✍️🐍
Introduction: Hey there Pythonistas! 👋 Have you ever wondered if it's possible to specify the type of items contained within a collection, such as a homogeneous list, for the purpose of type hinting in PyCharm and other IDEs? 🤔 Well, you're in luck! In this blog post, we'll explore how you can use Python 3's function annotations to achieve just that. So let's dive in and annotate our way to clean and reliable code! 🚀💡
The Problem:
Okay, imagine you have a function my_func
which takes a list of integers as an argument. You want to provide a clear type hint for this collection, but you're not sure how to do it using function annotations. 😕 Let's take a look at a pseudo-code example:
def my_func(l: list<int>):
pass
Seems simple enough, right? Unfortunately, Python doesn't support this syntax. But hold on! Don't lose hope just yet. There's a way to achieve the desired result using function annotations. Let's explore the solution together! 🧐🔍
The Solution:
The good news is that while the list<int>
syntax doesn't work directly, we can achieve the same effect using Docstring type hints. Let's take a look at an updated version of our function using Docstring:
def my_func(l):
"""
:type l: list[int]
"""
pass
By adding a type hint comment in the Docstring, we are effectively specifying that the parameter l
is expected to be a list containing integers. Voila! 🎉
Now, whenever we use my_func
and hover over the parameter in an IDE like PyCharm, we'll see the expected type hint information. This can immensely help you and your teammates understand the required types and catch potential bugs early on! 🐞🔎📝
Call-to-Action: Are you excited to start annotating your collections with specified types using Python's function annotations? 🙌💻 Give it a try in your next project, and share your experience in th
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
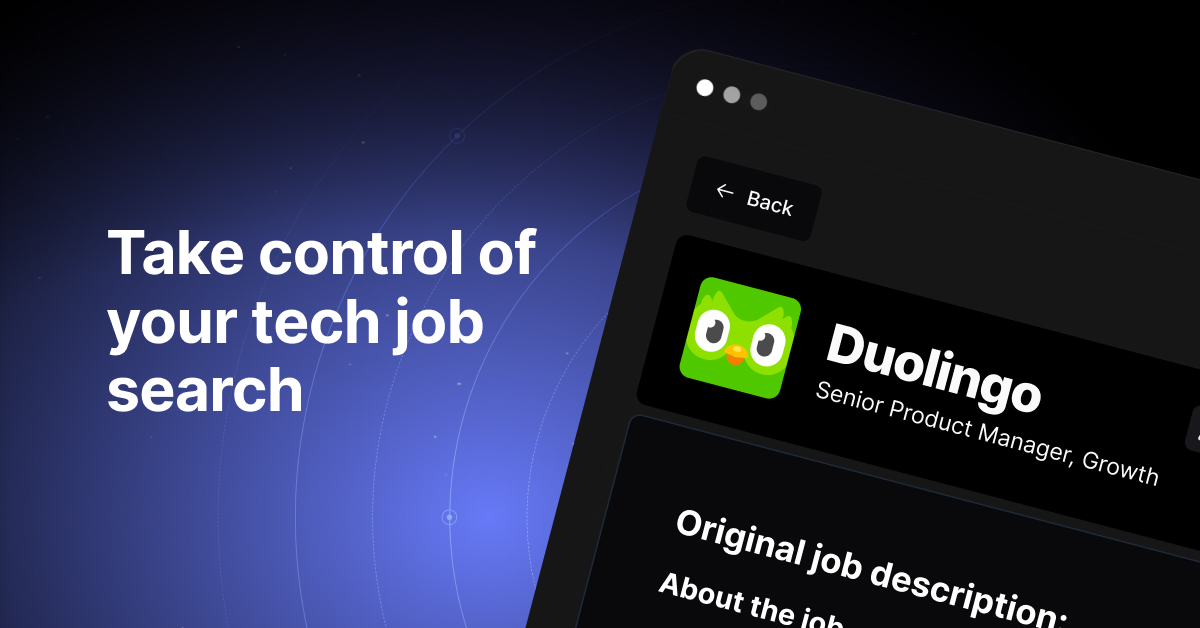