TransactionManagementError "You can"t execute queries until the end of the "atomic" block" while using signals, but only during Unit Testing
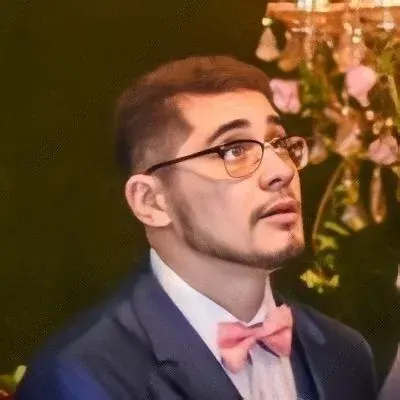
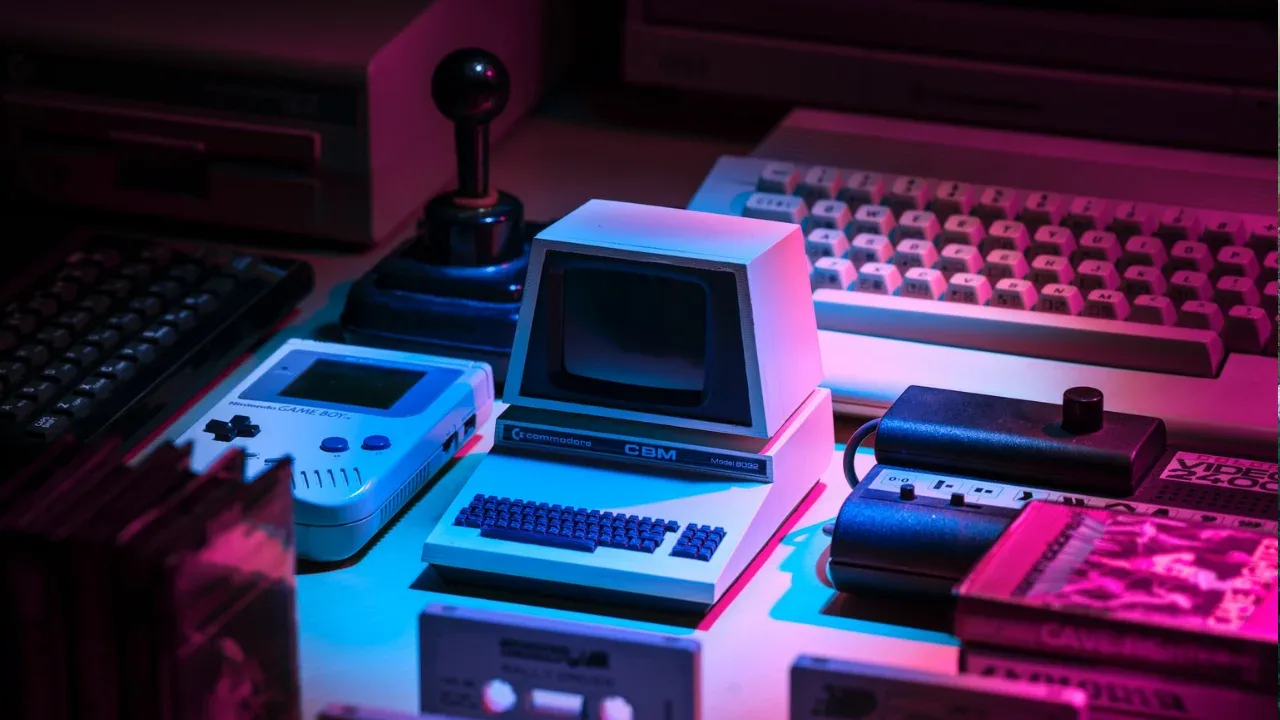
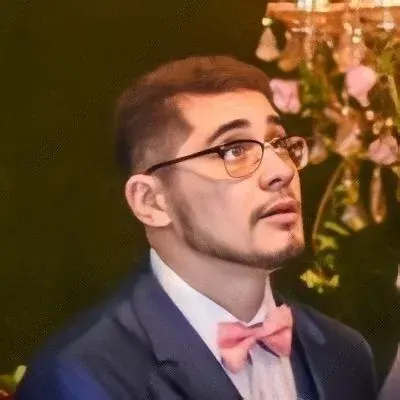
🔒💾 TransactionManagementError: "You can't execute queries until the end of the 'atomic' block" during Unit Testing
Are you experiencing a TransactionManagementError while using signals in Django during Unit Testing? If so, you're not alone! This error can be quite frustrating, especially when everything seems to work fine during manual testing. But don't worry, I'm here to help!
🔎 Understanding the Problem
So, what's causing this error? Let's take a closer look at the code snippets you provided.
In your views.py
, you have a mobileRegister
view that creates a new User instance and saves it. Then, in your signal.py
, you have a create_user_profile
function that is triggered by the post_save
signal of the User model. Inside this function, you're attempting to create related models (Company, CompanyContact, UserProfile, GCMDevice).
The error occurs specifically during Unit Testing, but not during manual testing. This suggests that the issue is related to the way transactions are handled in Django's testing framework.
⚙️ The Solution
To fix this issue, you can use the @transaction.atomic
decorator or the transaction.atomic()
context manager to wrap your test methods. This will ensure that all database operations within the block are executed within a single transaction.
Here's how you can modify your tests.py
to use @transaction.atomic
:
from django.test import TestCase
from django.db import transaction
class AuthTestCase(TestCase):
fixtures = ['nextgencatalogs/fixtures.json']
@transaction.atomic
def test_registration_api_get(self):
# Test code goes here
@transaction.atomic
def test_registration_api_post(self):
# Test code goes here
Alternatively, you can use the transaction.atomic()
context manager:
from django.test import TestCase
from django.db import transaction
class AuthTestCase(TestCase):
fixtures = ['nextgencatalogs/fixtures.json']
def test_registration_api_get(self):
with transaction.atomic():
# Test code goes here
def test_registration_api_post(self):
with transaction.atomic():
# Test code goes here
By using the transaction.atomic
decorator or context manager, you ensure that all database operations within the test methods are executed within a single transaction, preventing the "atomic block" error from occurring.
💡 Pro Tip: Instead of repeating the transaction.atomic()
block in each test method, you can also consider using a setUpClass
method to apply the atomic transaction to all test methods within the class.
📝 Wrapping Up
You have now learned about the TransactionManagementError that occurs during Unit Testing in Django when using signals. By using the @transaction.atomic
decorator or the transaction.atomic()
context manager, you can ensure that all database operations within the test methods are executed within a single transaction, resolving the issue.
Now it's your turn to give it a try! Apply the suggested solutions to your code and see if it resolves the TransactionManagementError during Unit Testing. Don't forget to share your results and any other questions or concerns you have about Django's testing framework.
📢 Call-to-Action: Have you encountered any other challenging issues in Django development? Let me know in the comments section below and I'll be glad to help you out!