Take multiple lists into dataframe
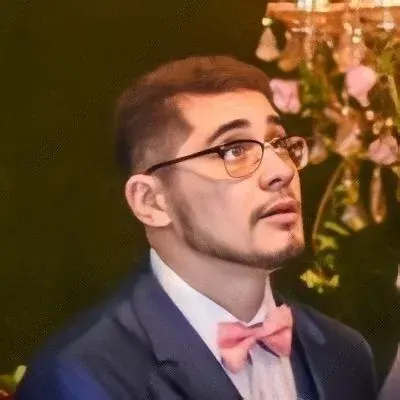
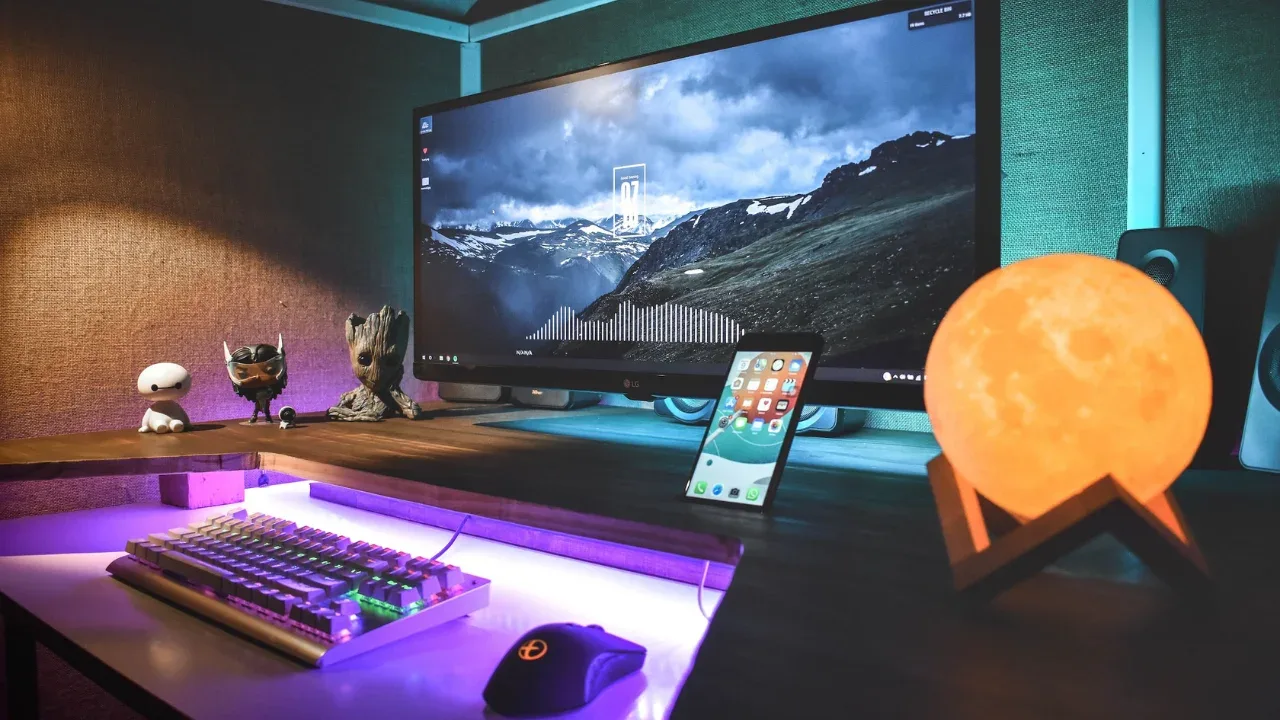
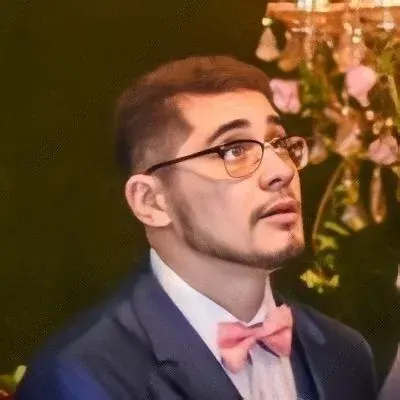
Taking Multiple Lists into a DataFrame: A Simple Guide
Are you struggling with the task of taking multiple lists and transforming them into different columns in a Python DataFrame? Look no further, as we have got you covered! In this guide, we will walk you through common issues that arise during this process and provide simple solutions to help you accomplish your goal effortlessly.
The Problem:
A user encountered difficulties while attempting to use a solution they found on Stack Overflow. Their initial attempts, as outlined below, did not yield the desired outcome:
Attempt 1:
The user tried zipping the lists together using
res = zip(lst1, lst2, lst3)
.However, this approach resulted in just one column, instead of the desired three columns.
Attempt 2:
In an alternative strategy, the user created a DataFrame using a dictionary.
The code snippet they used was as follows:
percentile_list = pd.DataFrame({'lst1Title': [lst1],
'lst2Title': [lst2],
'lst3Title': [lst3]},
columns=['lst1Title', 'lst2Title', 'lst3Title'])
Unfortunately, this approach either produced a DataFrame with one row and three columns (based on the original code), or a DataFrame with three rows and one column when transposed.
The Solution:
To create a DataFrame consisting of a hundred rows and three columns, where each column represents one of the three independent lists, follow these simple steps:
Define three separate lists, let's call them
lst1
,lst2
, andlst3
.Create a dictionary using the column names as keys and the lists as values.
data = {'Column1': lst1, 'Column2': lst2, 'Column3': lst3}
Convert the dictionary into a DataFrame.
df = pd.DataFrame(data)
Verify the dimensions of your DataFrame using
df.shape
. It should display(100, 3)
if each of your original lists has a length of 100.
That's it! You have successfully transformed your multiple lists into a DataFrame with three separate columns, each representing one of the originally provided lists. 🎉
Call to Action:
We hope this guide has been helpful in addressing your concerns about taking multiple lists into a DataFrame. Now it's your turn to try it out and see the amazing results for yourself! Don't forget to share your outcomes and experiences with us by leaving a comment below. Feel free to ask any further questions you may have, and we'll be more than happy to assist you further.
Happy coding! 💻💡