String formatting: % vs. .format vs. f-string literal
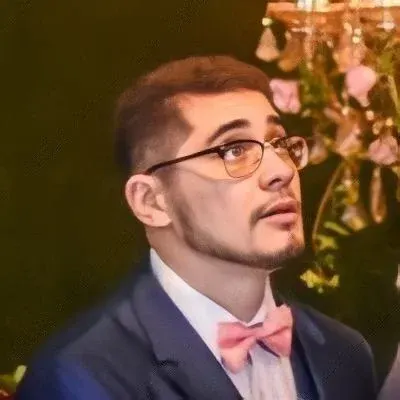
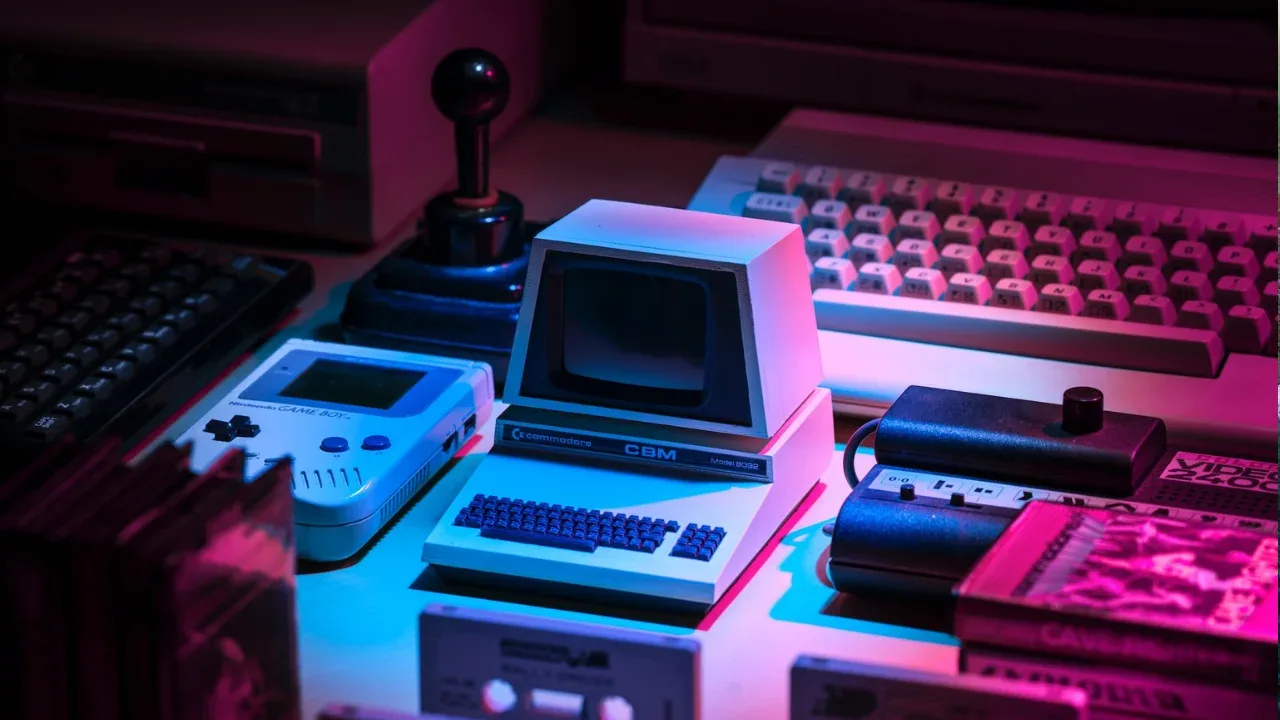
String Formatting: % vs. .format vs. f-string literal
<picture> <source srcset="https://images.unsplash.com/photo-1553473926-3c20a5f37c64" media="(min-width: 1200px)"> <source srcset="https://images.unsplash.com/photo-1553473926-3c20a5f37c64?w=1200" media="(min-width: 992px)"> <source srcset="https://images.unsplash.com/photo-1553473926-3c20a5f37c64?w=992" media="(min-width: 768px)"> <source srcset="https://images.unsplash.com/photo-1553473926-3c20a5f37c64?w=768" media="(min-width: 576px)"> <img src="https://images.unsplash.com/photo-1553473926-3c20a5f37c64?w=576" alt="String Formatting" style="width:auto;"> </picture>
If you've been coding in Python for a while, you may have come across different methods of formatting strings. It can be confusing to choose between the % operator, .format()
method, or the newer f-string literals. In this blog post, we'll explore the differences and help you decide which method is best for your situation.
The Different Methods of String Formatting
1. The % Operator
The % operator is the oldest string formatting method in Python. It is used in older versions of Python, before version 2.6. Here's an example:
name = "Alice"
message = "Hello %s" % name
The % operator allows you to substitute values into a string by using placeholders marked with %
. It is quite similar to how you would format strings in other languages like C.
2. The .format()
Method
Introduced in Python 2.6, the .format()
method offers more flexibility in string formatting. Here's an example:
name = "Alice"
message = "Hello {}".format(name)
With .format()
, you can use placeholders marked with {}
. These placeholders can be indexed or named arguments, allowing greater control over the string formatting.
3. F-String Literals
F-strings, introduced in Python 3.6, are the newest and most concise method of string formatting. Here's an example:
name = "Alice"
message = f"Hello {name}"
F-strings allow you to directly embed variables into the string by prefixing it with an f
. You can place the variable name inside {}
, and it will be replaced with its value.
Comparing the Methods
Now that we've seen the different methods, let's compare them based on common situations.
1. Simple String Formatting
All three methods can be used for simple string formatting where you are just substituting a single value into a string. Here's how it looks with each method:
% Operator:
name = "Alice"
message = "Hello %s" % name
.format()
Method:
name = "Alice"
message = "Hello {}".format(name)
F-String Literal:
name = "Alice"
message = f"Hello {name}"
In this case, all methods achieve the same outcome. It's mainly a matter of personal preference or compatibility with different Python versions.
2. Named Arguments
Using named arguments can make your code more readable and maintainable. The .format()
method and f-string literals shine in this scenario. Here's an example:
name = "Alice"
message = "Hello {kwarg}".format(kwarg=name)
name = "Alice"
message = f"Hello {name}"
With the % operator, using named arguments is possible but involves more syntax. Here's an example:
name = "Alice"
message = "Hello %(kwarg)s" % {'kwarg': name}
3. Formatting Numbers and Dates
The .format()
method is particularly useful when dealing with specific formatting requirements, such as formatting numbers or dates. Here's an example:
pi = 3.141592653589793
formatted_pi = "The value of pi is {:.2f}".format(pi)
4. Performance Considerations
String formatting in Python is generally fast, but there are cases where it can impact runtime performance. If you need to perform string formatting in a loop or in a performance-critical section of your code, there are a few best practices you can follow to minimize the impact:
Use f-strings: F-strings are generally faster than the other methods.
Avoid unnecessary conversions: Converting values unnecessarily can add overhead. For example, if you are formatting an integer, don't convert it to a string unless necessary.
Pre-compute values: If you have values that don't change during the loop, consider pre-computing them outside the loop to avoid repeated computations.
These considerations are important in cases where string formatting is performed frequently or in large quantities.
Conclusion
In conclusion, all three methods of string formatting have their strengths and weaknesses. The % operator is simpler and more familiar to those coming from other programming languages. .format()
provides more flexibility, especially when dealing with named arguments. F-strings offer a concise and readable syntax, but they are limited to Python 3.6 and above.
When choosing a method, consider the specific requirements of your project, the Python version you are using, and any performance considerations. Ultimately, it comes down to personal preference and coding style.
Now that you're equipped with the knowledge of these string formatting methods, go ahead and use them in your code. Experiment and find the one that suits you best!
Let us know in the comments which method you prefer and any tips you have for string formatting. Happy coding! π©βπ»π¬
References
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
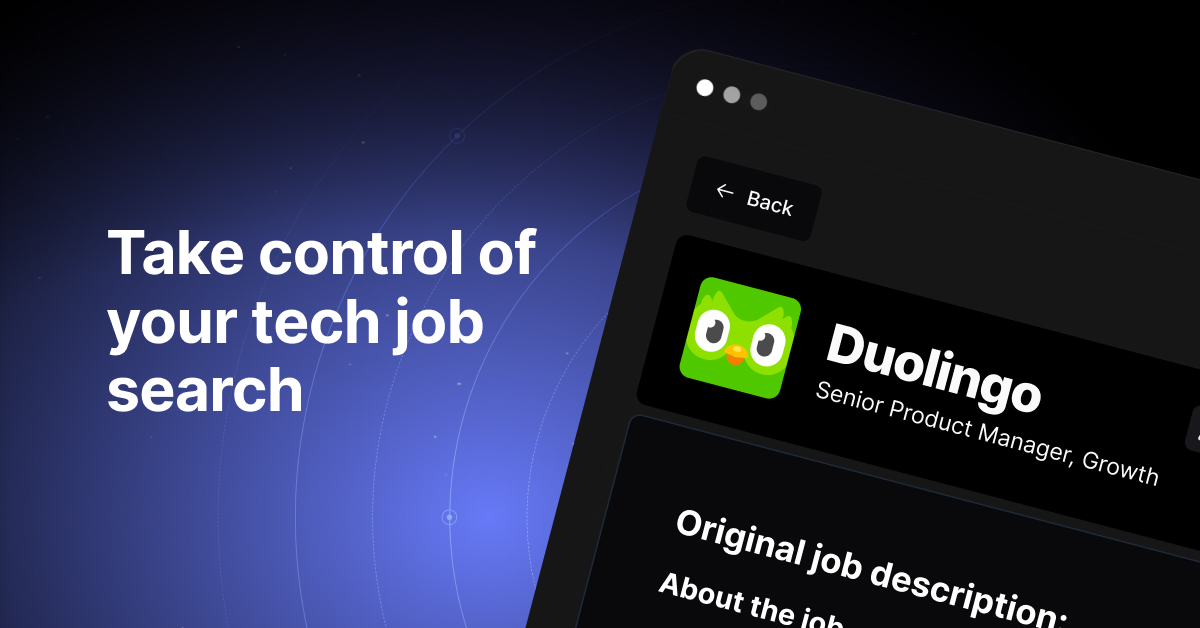