String comparison in Python: is vs. ==
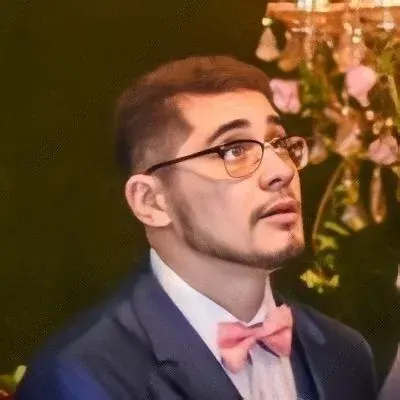
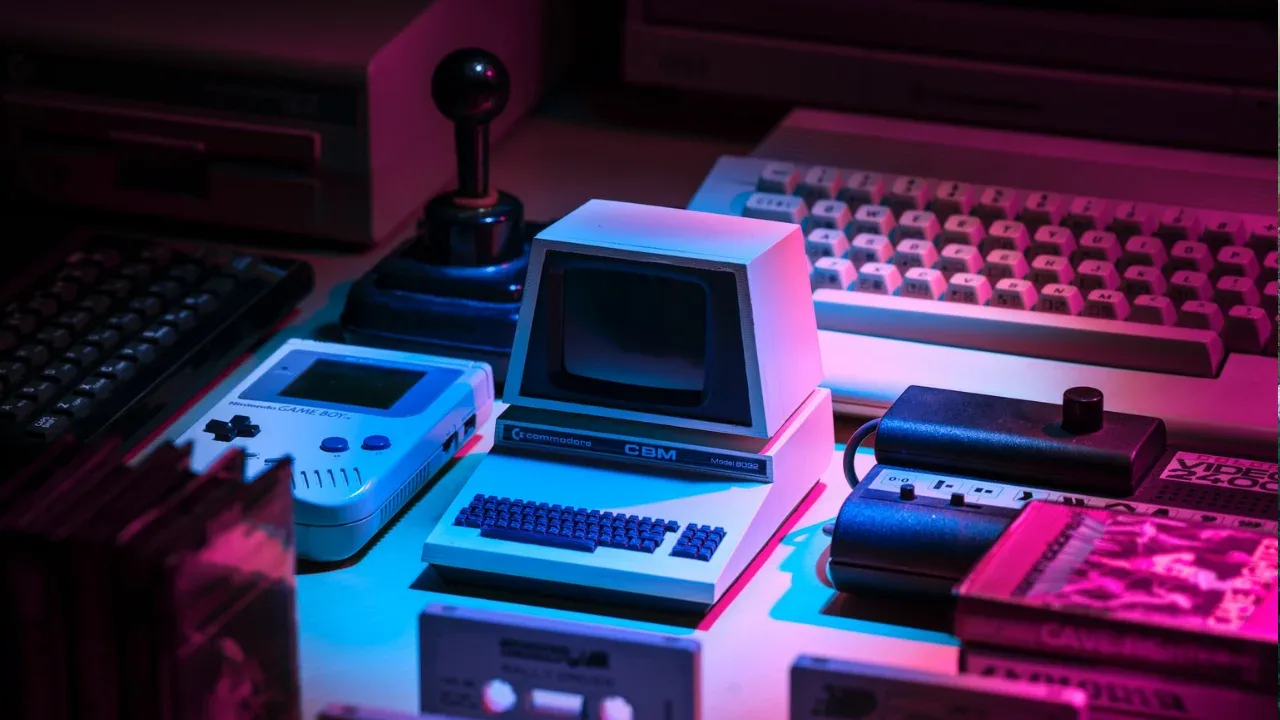
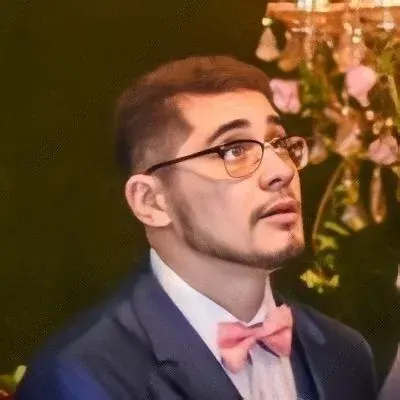
String Comparison in Python: is
vs ==
Have you ever encountered issues when comparing strings in Python? ๐ The confusion between using is
and ==
can lead to unexpected behaviors and bugs in your code. In this guide, we will address common problems associated with string comparison in Python and provide easy solutions to avoid them. Let's dive in! ๐ป๐
The Background Story
To set the stage, let's look at a real-life scenario shared by a fellow Python developer. They noticed that a script they were writing was acting peculiarly and traced it back to an infinite loop. The loop condition was defined as while line is not ''
. However, when they debugged the code, they discovered that line
was indeed an empty string (''
). ๐ฑ
while line is not '':
# Loop logic here
After some investigation, they realized that changing the condition to != ''
instead of is not ''
resolved the issue. This unexpected behavior raised questions about the appropriate use of is
and ==
operators in string comparison. Let's examine this in more detail. ๐ต๏ธโโ๏ธ๐
Understanding the Difference
In Python, both is
and ==
operators serve distinct purposes when it comes to comparing strings or any other objects. It is crucial to understand their differences to ensure correct usage. ๐ฅโ
is
Operator
The is
operator checks if two objects refer to the same memory location, or in simpler terms, if they are the same object. It compares the identity of the objects rather than their content. This is commonly used when you need to check if two variables point to the exact same object instance. Examples include comparing against singletons like None
or checking object identity. ๐งฉ๐
==
Operator
On the other hand, the ==
operator compares the values of two objects to check if they are equal. It checks if the content or value of the objects is the same, rather than their identity. This is the operator you should use for most comparison scenarios, especially when comparing string values. โ๏ธ๐
Best Practices and Solutions
Now that we understand the difference between is
and ==
, let's discuss some best practices and easy solutions to avoid common pitfalls in string comparison.
1. Comparing String Values
When comparing strings for equality, always use the ==
operator. This compares the actual content of the strings, ensuring accurate and expected results. In our earlier example, the developer encountered trouble because they used is not ''
instead of != ''
. Switching to != ''
corrected the problem. ๐โ
while line != '':
# Loop logic here
2. Comparing String Identifiers
If you specifically need to check if two strings refer to the exact same object instance rather than their content, then you can use the is
operator. This is a rare scenario and generally not necessary for typical string comparisons. Be cautious when using is
as it can lead to unexpected behavior if you're not comparing object identities intentionally. ๐งโ ๏ธ
3. Handling None
When comparing a string against None
, it is recommended to use is
for clarity and explicitness. Here's an example:
my_string = None
if my_string is None:
print("String is None")
Call-to-Action: Share Your Experiences!
We hope this guide has clarified the differences between is
and ==
operators when comparing strings in Python. Understanding these nuances will help you write more reliable and bug-free code. ๐๐
Have you ever encountered issues or bugs related to string comparison? Share your experiences and join the conversation! ๐ฌ๐ง Let us know in the comments below, and don't forget to share this post with your fellow Pythonistas. Happy coding! ๐ปโค๏ธ