Storing Python dictionaries
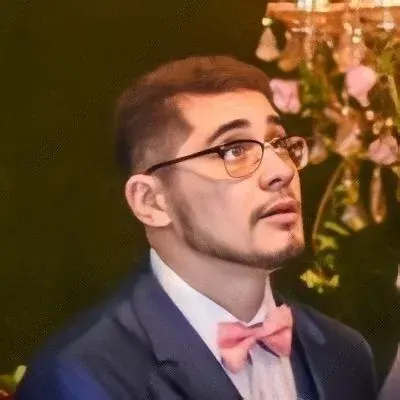
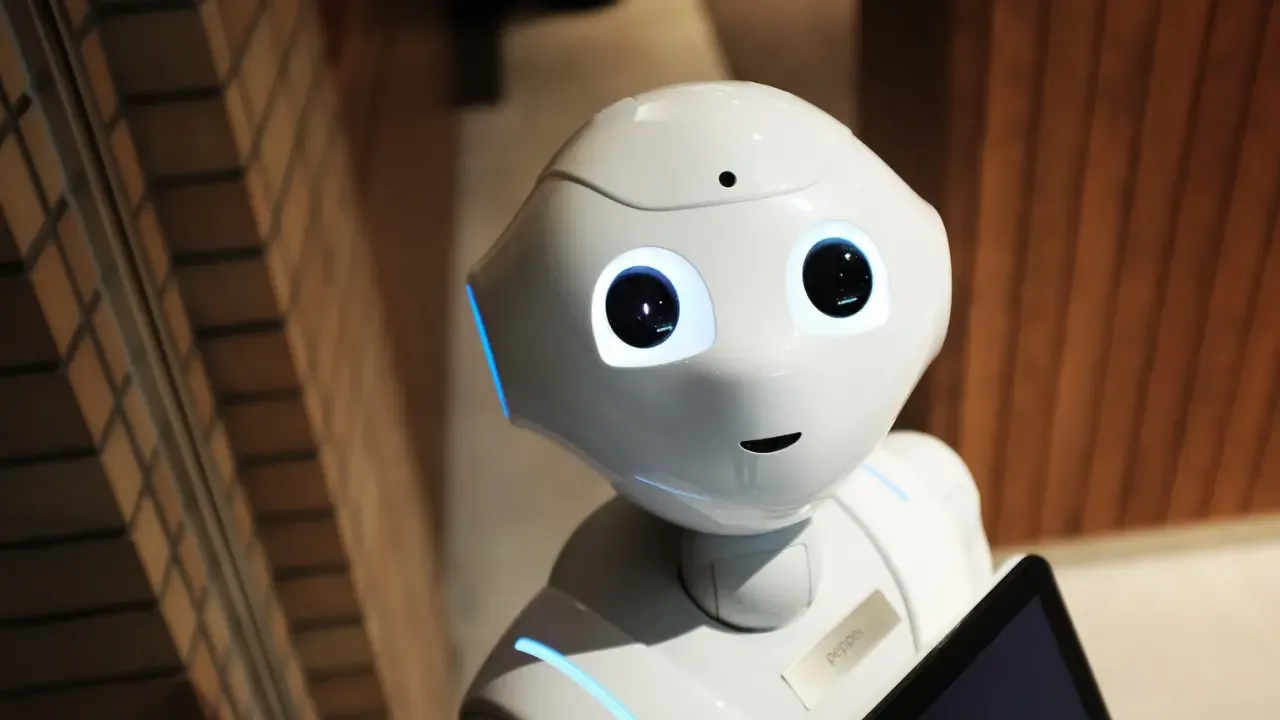
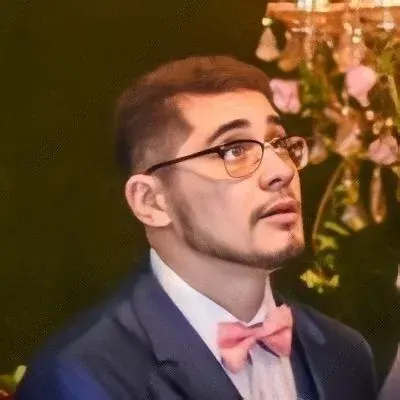
📝 Storing Python dictionaries made easy! 🐍🗂️
Are you tired of dealing with CSV files to store your Python dictionaries? 🤔 Don't worry, there are simpler ways to store your precious data! In this blog post, we will explore how to store dictionaries in JSON 📄 or pickle 🥒 files, and how to effortlessly bring them back to life when you need them. Let's dive in! 💦
The Challenge
CSV files have their own limitations, which can make handling dictionaries a bit tricky. But fear not! We have elegant alternatives. 🎩✨
Storing in JSON 📄
To store dictionaries in a JSON file, Python provides built-in support that's both easy to use and widely compatible. Here's how you can save your dictionary:
import json
data = {'key1': 'keyinfo', 'key2': 'keyinfo2'}
with open('data.json', 'w') as json_file:
json.dump(data, json_file)
By using the json
module, you can transform your dictionary into a JSON string and write it to a file. The resulting data.json
file will contain your stored dictionary. 🎉
Loading from JSON 📄
Retrieving your dictionary from a JSON file is just as simple! Here's how you can load it back into Python:
import json
with open('data.json', 'r') as json_file:
data = json.load(json_file)
print(data) # Output: {'key1': 'keyinfo', 'key2': 'keyinfo2'}
Using the json.load()
method, you can bring your dictionary back to life with all its original key-value pairs intact. 🧙♂️✨
Storing in Pickle 🥒
If you prefer a more efficient way of storing your dictionaries, Python's pickle
module can pickle your data into a binary format. The advantage of this approach is that it preserves the object types and supports more complex data structures. Here's how you can pickle your dictionary:
import pickle
data = {'key1': 'keyinfo', 'key2': 'keyinfo2'}
with open('data.pickle', 'wb') as pickle_file:
pickle.dump(data, pickle_file)
In this example, we use the pickle.dump()
method to pickle the dictionary into a binary file called data.pickle
.
Loading from Pickle 🥒
To load your pickled dictionary back into Python, follow these steps:
import pickle
with open('data.pickle', 'rb') as pickle_file:
data = pickle.load(pickle_file)
print(data) # Output: {'key1': 'keyinfo', 'key2': 'keyinfo2'}
Using the pickle.load()
method, your dictionary will be restored with all its original glory! 🌟🔮
What's Next? 🚀
Now that you know how to easily store and load Python dictionaries using JSON and pickle files, you can level up your data handling game! Feel free to explore more features of these modules and adapt them to your specific needs. 🤓
If you have any questions or want to share your experiences, leave a comment below. Let's discuss and learn together! 💬📚
Happy coding! 💻🎉