@staticmethod vs @classmethod in Python
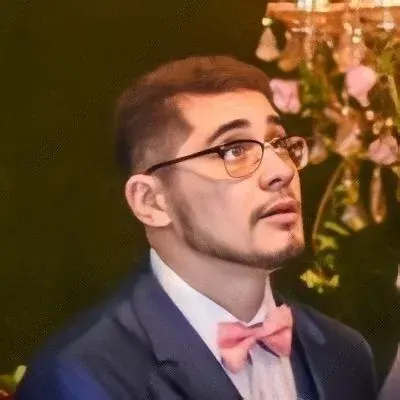
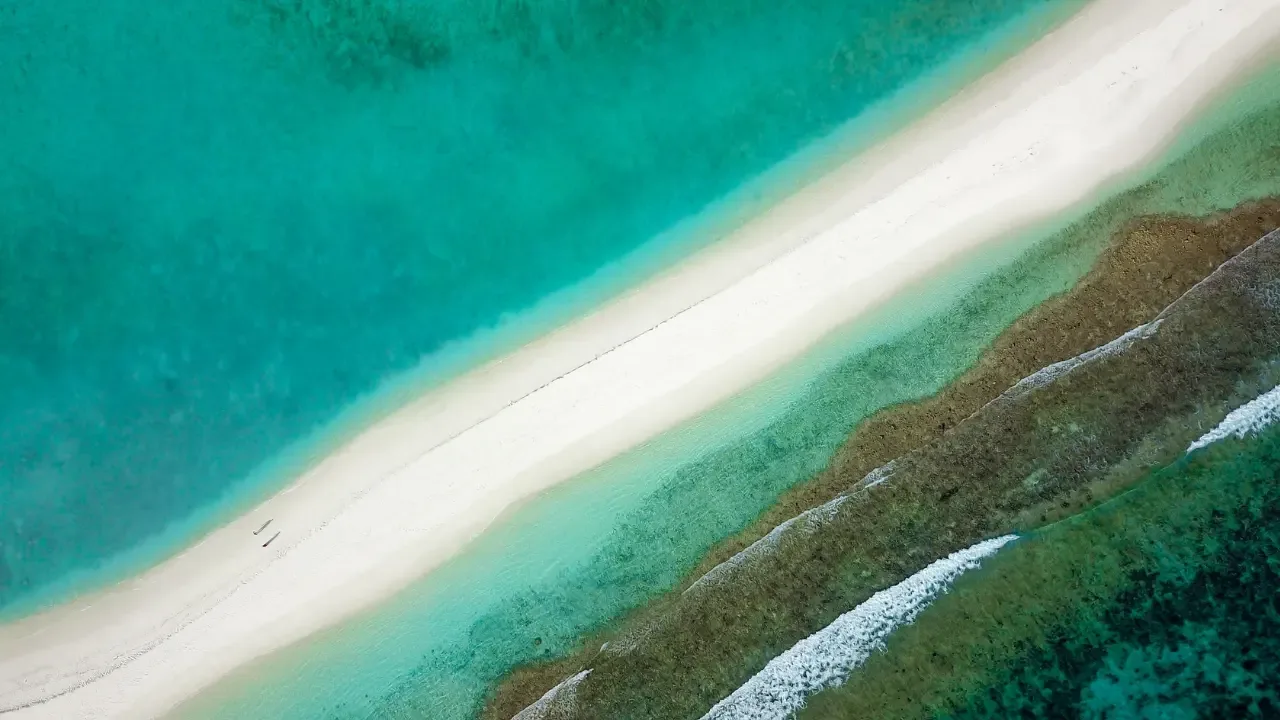
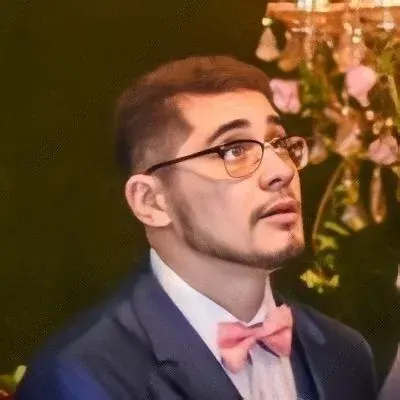
๐ข Hey Pythonistas! ๐๐
Are you wondering about the difference between @staticmethod
and @classmethod
in Python? ๐ค You've come to the right place! In this blog post, we'll dive deep into understanding these decorators and their unique functionalities. ๐ก
What's the Buzz about @staticmethod and @classmethod? ๐๐
Let's start with the basics. Both @staticmethod
and @classmethod
are decorators used to define special behaviors within classes. However, they have distinct purposes and are used in different scenarios.
Understanding @staticmethod ๐ง ๐ง
When you decorate a method with @staticmethod
, you're telling Python that the method is purely related to the class it belongs to. It doesn't rely on any instance variables or class-level variables. In simpler terms, it's an independent function encapsulated within the class. ๐ค
class MyClass:
@staticmethod
def my_static_method():
# Code goes here
Static methods are especially useful when you need to group utility functions together or when you want a method to be accessed without instantiating the class. Think of methods that perform generic calculations or provide common functionality across instances. ๐งฎ๐ข
Unveiling @classmethod ๐ฉ๐
Unlike @staticmethod
, @classmethod
decorators are used for methods that require access to the class itself. These methods have a special first parameter, commonly named cls
, which represents the class being operated on. This enables them to access and modify class-level variables and behaviors. ๐ซ๐
class MyClass:
@classmethod
def my_class_method(cls):
# Code goes here
Class methods come in handy when you need to manipulate or modify class-level attributes, create alternative constructors, or implement design patterns like factory methods. ๐ญ๐ก
Lightbulb Moment: Spotting the Difference ๐กโจ
Now, let's break down the key differences between @staticmethod
and @classmethod
:
๐น Purpose: Static methods are independent and don't rely on class or instance-specific data. Class methods, on the other hand, have access to the class and its attributes.
๐น First Parameter: Static methods have no obligatory parameters. Class methods have the cls
parameter, which refers to the class itself.
๐น Inheritance: Static methods can't be overridden by subclasses. Class methods can be overridden and customized in inheriting classes.
Solutions at Hand โ๐ก
If you find yourself scratching your head over whether to use @staticmethod
or @classmethod
, keep these tips in mind:
๐ธ Use @staticmethod
when you have self-contained methods that are unrelated to instance or class data.
๐ธ Use @classmethod
when you need to modify or access class-specific data or behaviors.
Remember, the right choice depends on the problem you're solving and the design of your class hierarchy. Choose wisely, young Pythonista! ๐งโโ๏ธโจ
Join the Pythonista Community! ๐ค๐
Have you encountered situations where @staticmethod
or @classmethod
made a difference in your code? Share your experiences and insights with our amazing community in the comments below! ๐ฃ๏ธ๐ฌ
Happy coding! ๐ป๐