Static methods in Python?
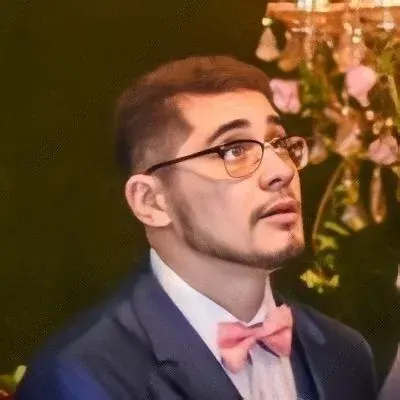
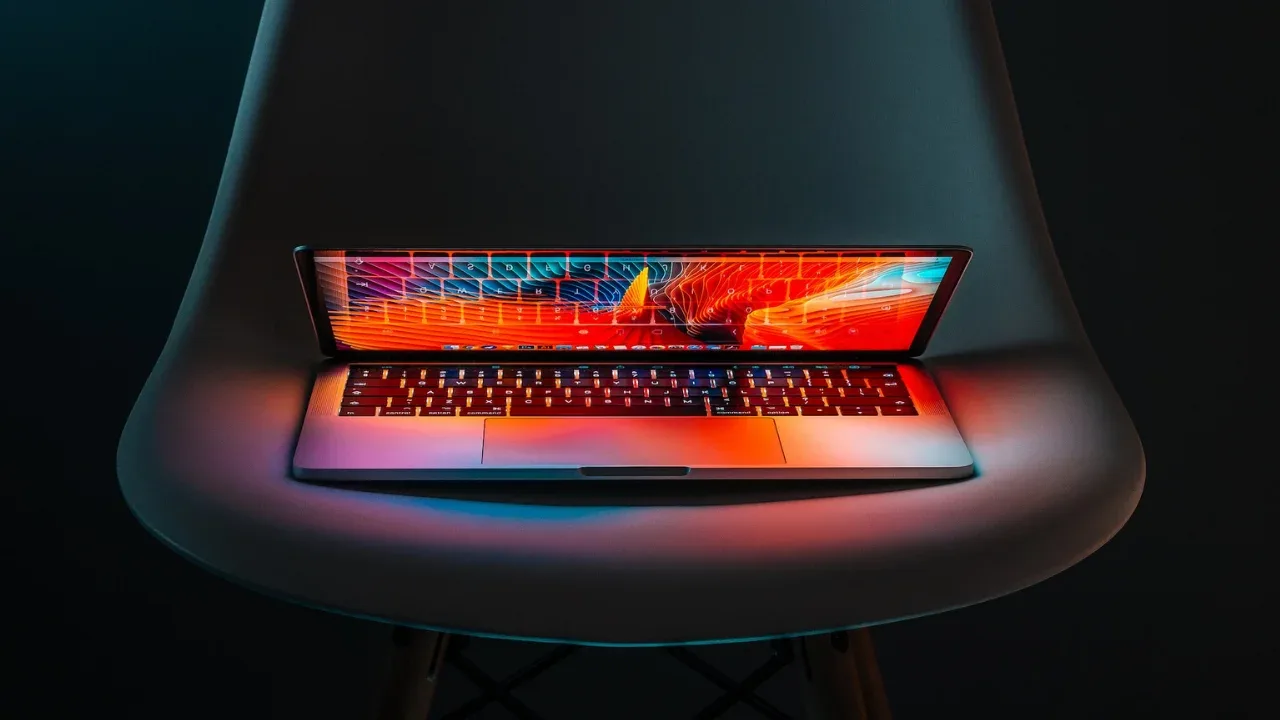
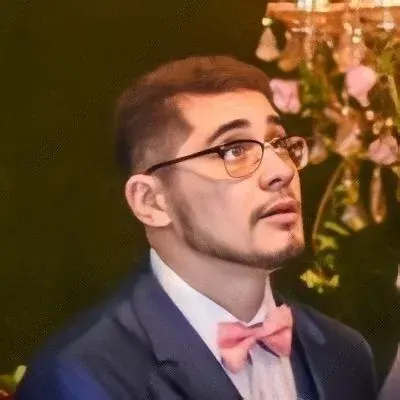
๐ Unleash the Power of Static Methods in Python ๐
Are you ready to level up your Python game? Today, we're diving deep into the world of static methods! ๐คฏ
So, what exactly are static methods in Python? ๐ค Simply put, static methods are functions defined within a class that can be called directly on the class itself, rather than on an instance of the class. This means you don't need to create an object to use a static method! ๐
Common Pitfall Alert! โ ๏ธ One common misconception is that you can call a static method using an instance of the class. Well, here's the truth bomb, my friend. Static methods can only be called using the class name! ๐งจ
Let's dive into the โจ magic โจ of static methods and explore a practical example.
The Power of Static Methods
Consider a scenario where you want to calculate the area of a circle. You might create a Circle class with a static method to help you out:
class Circle:
@staticmethod
def calculate_area(radius):
return 3.14 * radius * radius
Voilร ! ๐ฉ Now you can call the static method directly on the class, without having to create an instance:
print(Circle.calculate_area(5))
The output, in this case, would be 78.5
. Pretty neat, huh? ๐
The Benefits of Static Methods
Static methods serve some practical purposes:
๐ They enable you to organize your code within a class, even if the method doesn't require any instance-specific data. It's a great way to keep things tidy and maintainable.
๐ Static methods can be easily tested without the need to create an instance of the class. Testing just got a whole lot easier! ๐งชโ
๐ They facilitate the creation of utility functions that don't depend on any external state. Have a bunch of unrelated functions? Bundle them up in a static method paradise! ๐
Now, think of all the possibilities! You can use static methods for data validation, utility functions, or even for creating alternative constructors. The sky's the limit! โ๏ธ๐
Level Up Your Python Game
To harness the true power of static methods in your Python programs, keep these things in mind:
1๏ธโฃ Decorate your method: Use the @staticmethod
decorator in Python to declare a static method within a class.
2๏ธโฃ No self-argument: Unlike regular instance methods that accept self
as the first parameter, static methods have no implicit first argument. So, no need to pass an instance when calling them!
3๏ธโฃ Clear code structure: Utilize static methods to keep your code organized, especially for methods that don't rely on instance-specific data.
Now that you've learned the ins and outs of static methods, go forth and conquer the Python world! ๐๐
Remember, with great power comes great responsibility. Use static methods wisely and watch your code flourish. โจ
Share your thoughts in the comments below and tell us about the coolest way you've utilized static methods! Let's inspire each other! ๐กโ๏ธ
Happy coding! ๐ป๐