Split string with multiple delimiters in Python
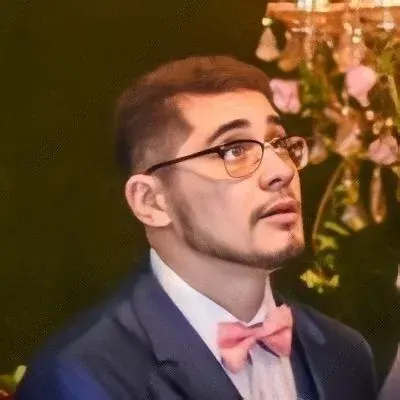
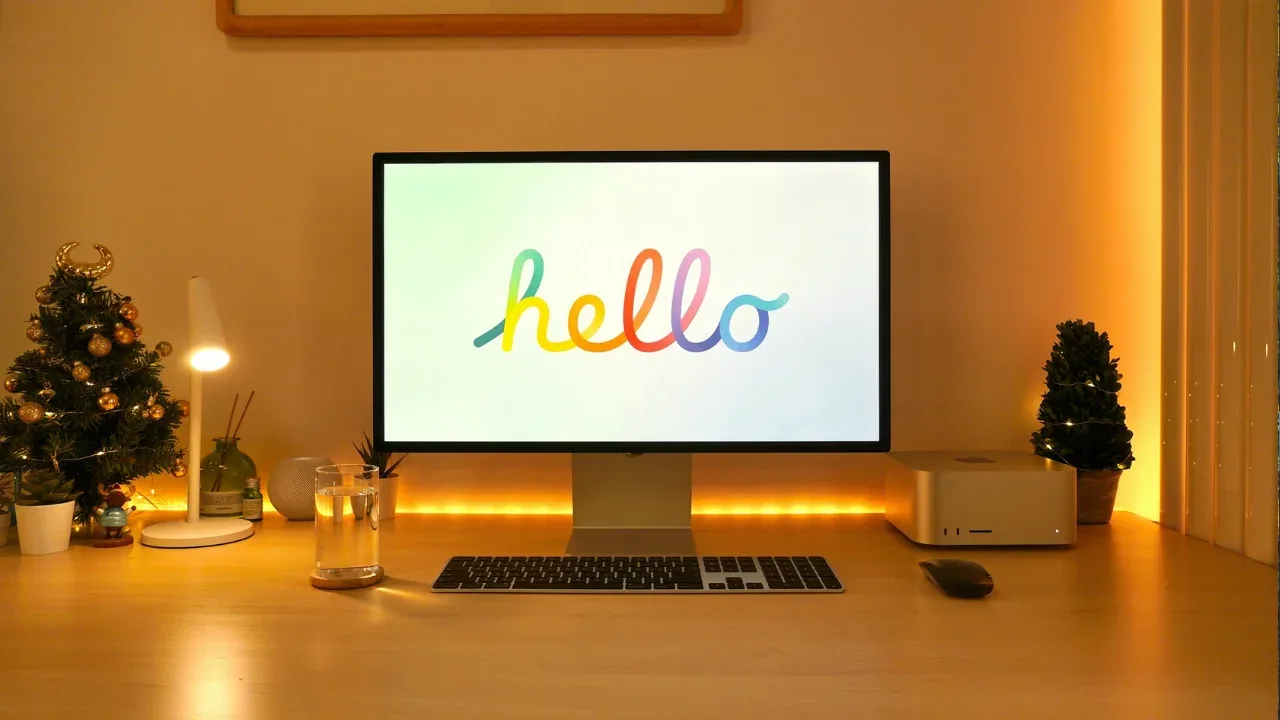
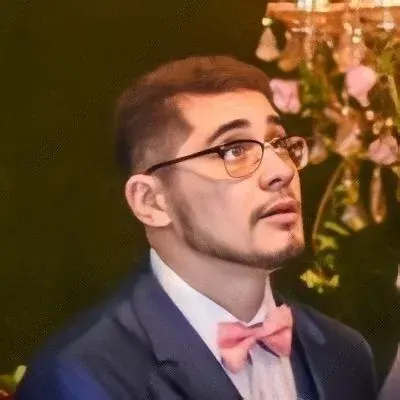
š Tech Blog: Splitting Strings with Multiple Delimiters in Python š
š Welcome to our tech blog! Today, we'll dive into the world of Python and explore how to split strings that have multiple delimiters. If regular expressions scare you, fear not! We've got you covered with easy-to-understand solutions. Let's get started! š
The Problem š¤
Imagine you have a string that needs to be split by either a semicolon (;
) or a comma followed by a space (,
). However, if a comma appears without a trailing space, it should be left untouched. Our goal is to split the string into a list of separate elements based on these delimiters.
The Example š
Let's take a look at an example string to better understand the problem:
"b-staged divinylsiloxane-bis-benzocyclobutene [124221-30-3], mesitylene [000108-67-8]; polymerized 1,2-dihydro-2,2,4-trimethyl quinoline [026780-96-1]"
Our desired output should be:
['b-staged divinylsiloxane-bis-benzocyclobutene [124221-30-3]', 'mesitylene [000108-67-8]', 'polymerized 1,2-dihydro-2,2,4-trimethyl quinoline [026780-96-1]']
The Solution š”
To achieve the desired output, we can leverage the power of Python's re
module (regular expressions) and the split
function. Here's a step-by-step guide on how to do it:
Import the
re
module:
import re
Define the input string:
input_string = "b-staged divinylsiloxane-bis-benzocyclobutene [124221-30-3], mesitylene [000108-67-8]; polymerized 1,2-dihydro-2,2,4-trimethyl quinoline [026780-96-1]"
Use the
re.split
function with a regular expression pattern to split the string:
output_list = re.split(r'[;,]\s', input_string)
The regular expression pattern [;,]\s
matches either a semicolon (;
) or a comma followed by a space (,
).
Testing and Expected Output āļø
Now let's test our solution by printing the output list:
print(output_list)
The expected output should be:
['b-staged divinylsiloxane-bis-benzocyclobutene [124221-30-3]', 'mesitylene [000108-67-8]', 'polymerized 1,2-dihydro-2,2,4-trimethyl quinoline [026780-96-1]']
Voila! The input string has been successfully split into separate elements based on our specified delimiters.
Conclusion and Call-to-Action š
Congratulations! You now know how to split strings with multiple delimiters in Python using regular expressions. Don't let complex-looking problems intimidate you. With the right tools and guidance, you can conquer any coding challenge! šŖ
We hope you found this blog post helpful and easy to understand. If you have any questions, suggestions, or other cool Python topics you'd like us to cover, please leave a comment below. Happy coding! šāØ