Split string on whitespace in Python
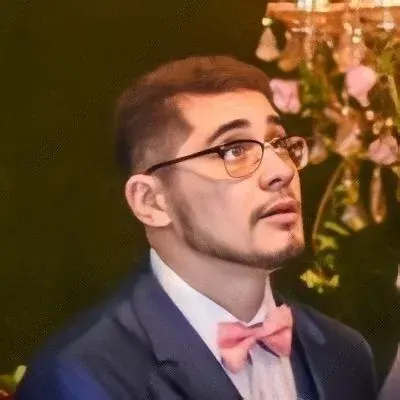
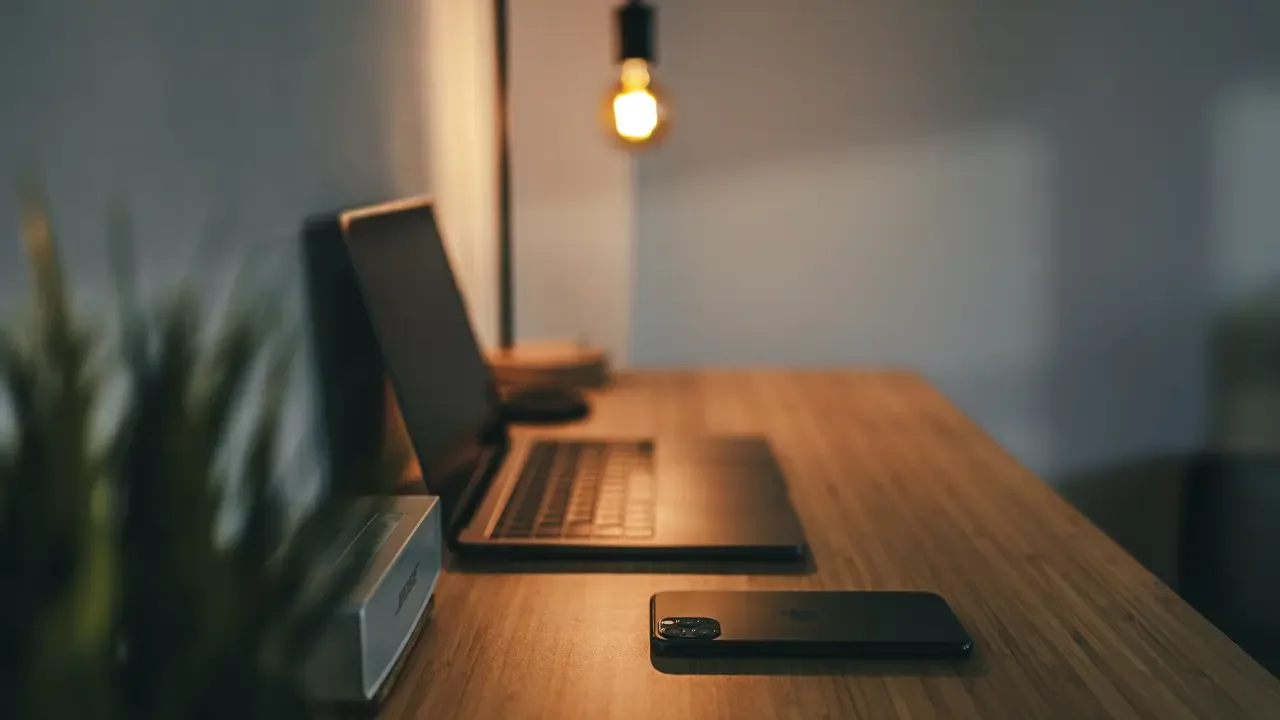
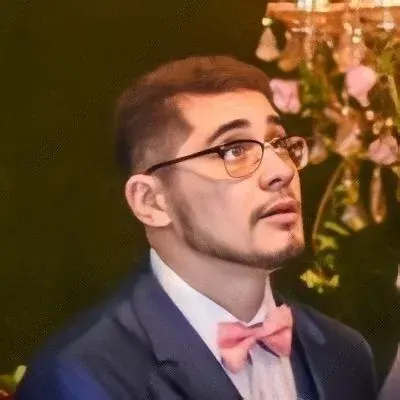
🐍 Splitting Strings on Whitespace in Python: A Simple Guide 🤓
Are you tired of struggling to split a string into words in Python? Look no further, because we've got you covered! In this guide, we'll walk you through the common issues and provide easy solutions, so you can split those strings like a pro. Let's dive in! 💪
Problem: Splitting a String on Whitespace
Consider the following string:
str = "many fancy word \nhello \thi"
And the desired output is:
["many", "fancy", "word", "hello", "hi"]
The challenge here is to split the string on any whitespace character while handling consecutive whitespaces and newline characters properly.
Solution: Using the split()
Function
In Python, you can utilize the built-in split()
function to split a string on whitespace. By default, split()
treats consecutive whitespaces as a single delimiter and removes leading/trailing whitespaces. Here's how you can accomplish it:
words = str.split() # Split the string on whitespace
That's it! The split()
function does all the heavy lifting for you. It automatically handles multiple whitespaces and newlines, and returns a list of words. 🎉
Handling Regex Patterns
But what if you want to split on whitespace characters defined by a specific regular expression pattern? Just like the Java example you provided, where they split on \\s
.
Good news! Python's split()
function also accepts regular expressions as delimiters. You can achieve the same behavior as the Java code using the re
module. Here's a modified version of the solution:
import re
str = "many fancy word \nhello \thi"
words = re.split(r"\s", str) # Split the string on any whitespace
In this code snippet, we import the re
module and use the split()
function from that module, passing \s
as the regular expression pattern. This will split the string on any whitespace character.
Conclusion
Splitting a string on whitespace in Python doesn't have to be challenging. By using the split()
function, you can effortlessly achieve the desired result.
Whether you want to split on consecutive whitespaces or a specific regular expression pattern, Python gives you the flexibility to handle both cases.
Now it's your turn! Try out these techniques in your own code and feel the power of string splitting in Python. 🚀
If you have any questions or other cool string manipulation tricks, share them in the comments below. Happy coding! 😄✨