Split string every nth character
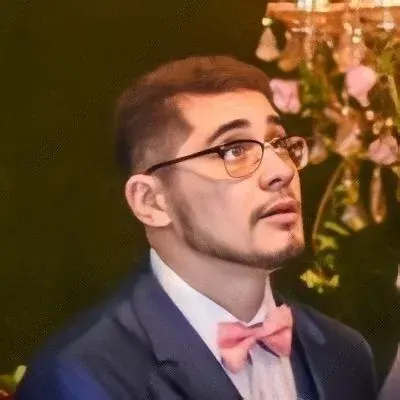
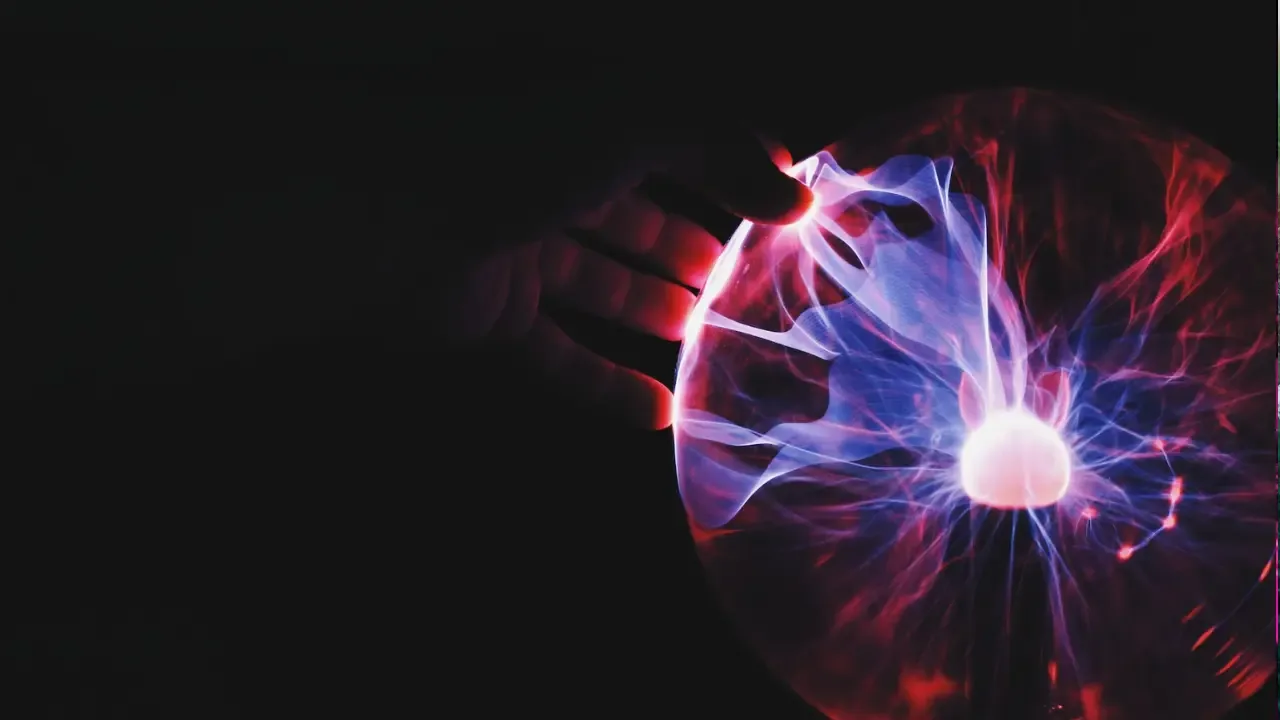
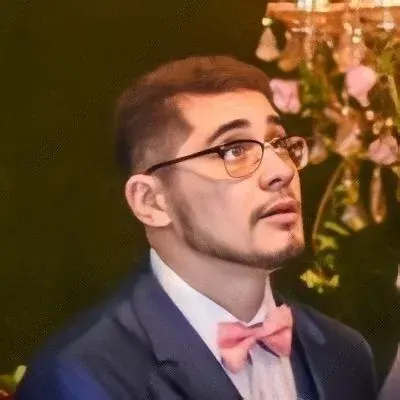
Splitting Strings Every nth Character Made Easy ๐งต
Do you ever find yourself needing to split a string into smaller chunks of a specific length? Maybe you have a string of numbers and you want to split it into groups of two, three, or any other number of characters. Well, you're in luck! In this blog post, we'll explore different approaches to splitting a string every nth character, and provide you with easy solutions to tackle this problem head-on.
The Problem ๐
Let's take a look at an example to better understand the problem at hand. Suppose we have the string:
'1234567890'
And we want to split it into groups of two characters. The desired output would be a list of strings, where each string represents a group:
['12', '34', '56', '78', '90']
So, how can we achieve this splitting magic? Let's dive in and see!
Solutions ๐ก
Solution 1: Using a Loop
One common solution is to iterate over the string and create substrings of the desired length using a loop. Here's how you can do it in Python:
def split_string(string, n):
return [string[i:i+n] for i in range(0, len(string), n)]
Let's break down this solution step-by-step:
We define a function
split_string
that takes two parameters:string
(the input string we want to split) andn
(the desired length of each substring).Inside the function, we use a list comprehension to create substrings of length
n
. Therange(0, len(string), n)
part generates a sequence of indices that starts from 0 and increments byn
in each iteration of the loop.We access the characters in the string using slicing (
string[i:i+n]
) and add each substring to the resulting list.Finally, we return the list of substrings.
Let's give it a try:
string = '1234567890'
result = split_string(string, 2)
print(result) # Output: ['12', '34', '56', '78', '90']
Solution 2: Using Regular Expressions
If you prefer a more flexible solution or your splitting requirements are more complex, you can use regular expressions. Here's an example implementation in Python using the re
module:
import re
def split_string_regex(string, n):
pattern = f'.{{1,{n}}}'
return re.findall(pattern, string)
In this solution, we're using the re.findall
function to find all matches of a given pattern in the input string. The pattern '.{1,n}'
specifies that we want to match sequences of any character (except newline) with a minimum length of 1 and a maximum length of n
.
Let's test it out:
string = '1234567890'
result = split_string_regex(string, 2)
print(result) # Output: ['12', '34', '56', '78', '90']
๐ฅ Your Turn to Split Some Strings!
Now that you have two easy solutions to split strings every nth character, it's time for you to put them into practice! Grab some strings and unleash the power of string splitting with these versatile techniques.
Conclusion ๐
Splitting strings into smaller chunks can be a common task when working with text data. In this blog post, we explored two straightforward solutions to split strings every nth character. Whether you choose the looping approach or embrace the power of regular expressions, you now have the tools to conquer this challenge.
Remember, the key is to understand the problem and choose the solution that best fits your needs. So go ahead, split those strings and conquer the world of text manipulation!
Share Your Experience! ๐
Have you encountered any interesting scenarios where splitting strings every nth character proved to be useful? Share your experiences and any additional tips in the comments below. Don't forget to spread the word by sharing this blog post with your fellow programmers. Happy string splitting! ๐๐