Split / Explode a column of dictionaries into separate columns with pandas
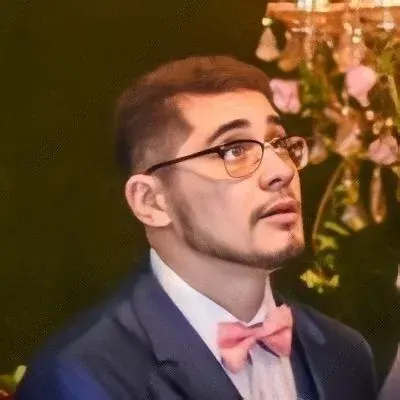
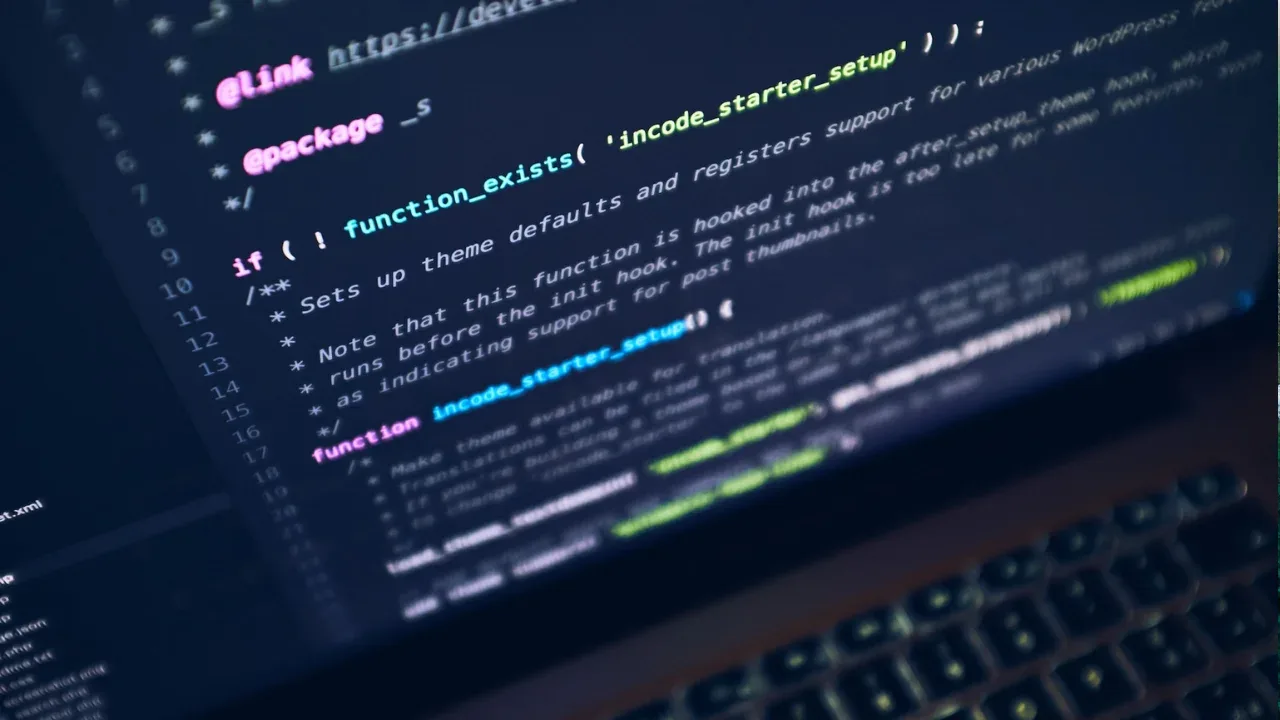
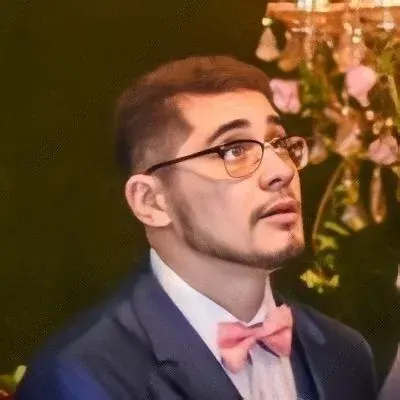
Splitting a column of dictionaries into separate columns with pandas
Are you struggling to split a column of dictionaries into separate columns in a pandas DataFrame? Look no further! In this guide, I'll walk you through a step-by-step solution to this common problem. Let's dive in! 💪
Understanding the problem
You have a pandas DataFrame, df
, with a column called Pollutants
that contains dictionaries. Your goal is to split this column into separate columns, a
, b
, and c
, with the corresponding values from each dictionary.
However, there are a few challenges you need to address. First, the lists within the dictionaries have different lengths. Second, the order of the keys in the dictionaries is consistent, with 'a' coming first, then 'b', and finally 'c'.
The code that USED to work
Previously, you were using the following code to split the column and create a new DataFrame, df2
:
objs = [df, pandas.DataFrame(df['Pollutants'].tolist()).iloc[:, :3]]
df2 = pandas.concat(objs, axis=1).drop('Pollutants', axis=1)
However, recently, you encountered an IndexError: out-of-bounds on slice (end)
error, indicating that the code is no longer working as expected.
Finding a robust solution
To overcome the issues and find a more robust solution, we'll take a different approach. Let's start by converting the Unicode strings into proper dictionaries.
import ast
# Convert Unicode strings to dictionaries
df['Pollutants'] = df['Pollutants'].apply(ast.literal_eval)
Now that the column values are proper dictionaries, we can easily split them into separate columns.
# Split the Pollutants column into separate columns
df2 = pd.concat([df.drop('Pollutants', axis=1), df['Pollutants'].apply(pd.Series)], axis=1)
Let's break down the code:
We drop the original
Pollutants
column fromdf
usingdf.drop('Pollutants', axis=1)
since we will replace it with the new columns.We create new columns by applying
pd.Series
to each value in thePollutants
column usingdf['Pollutants'].apply(pd.Series)
.Finally, we concatenate the two DataFrames,
df.drop('Pollutants', axis=1)
and the newly created columns, usingpd.concat
.
Handling missing values
By default, missing values will be filled with NaN
in the new columns. If desired, you can replace these NaN
values with another value, such as 0, using the fillna()
method:
df2.fillna(0, inplace=True)
Summary
To recap, here are the steps to split a column of dictionaries into separate columns in a pandas DataFrame:
Convert Unicode strings to dictionaries using
ast.literal_eval
.Use
pd.concat
andpd.Series
to split the column into separate columns.Optionally, handle missing values using
fillna()
.
Your turn to try it out!
Now it's your turn to give it a shot! Apply these steps to your code and see if it solves your issue. Make sure to let me know in the comments if you encounter any difficulties or have any questions. 🤔
So go ahead and give it a try! Happy coding! 💻✨