Split a string by spaces -- preserving quoted substrings -- in Python
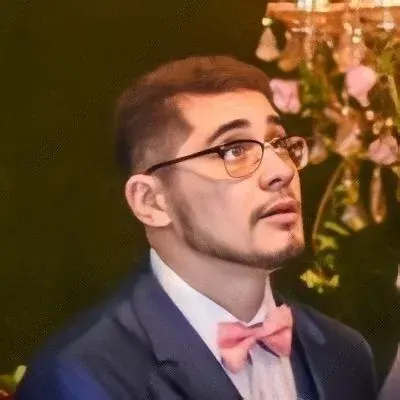
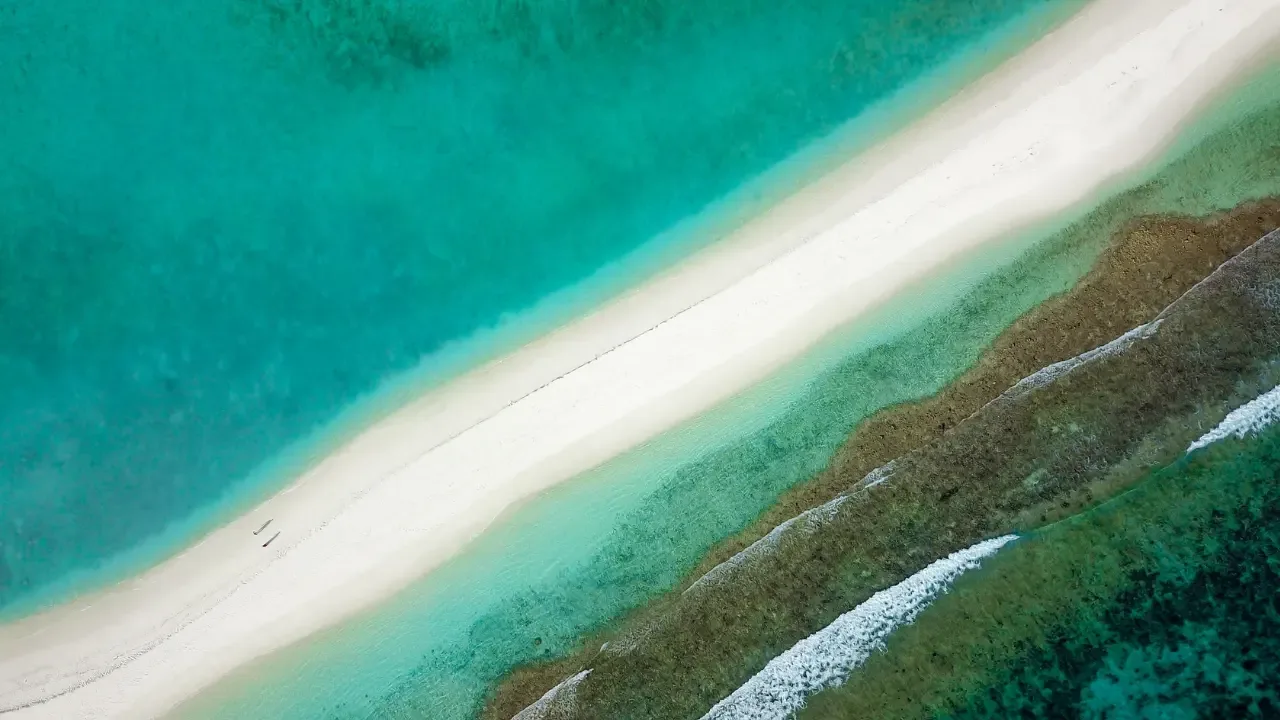
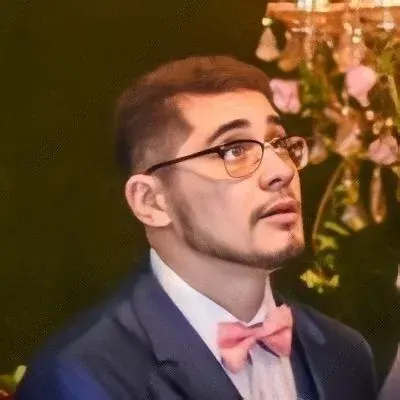
Split a string by spaces, preserving quoted substrings in Python
So you have a string and you want to split it by spaces in Python, but you also need to preserve any substrings that are enclosed in quotes. 🤔
Let's break down this problem and find an easy solution! 😎
Understanding the problem
You have a string like this:
this is "a test"
And you want to split it into a list of separate words, while ignoring spaces within quotes. The desired result would be:
['this', 'is', 'a test']
The solution
To achieve this, we can use regular expressions in Python. There's a handy module called re
which provides support for regular expressions. 🧰
Here's how we can solve this problem step-by-step:
Import the
re
module:
import re
Define the regular expression pattern:
pattern = r'("[^"]*"|\S+)'
("[^"]*"|\S+)
matches either a quoted string ("[^"]*"
) or a non-whitespace character sequence (\S+
). The[^"]*
part matches any character except a double quote, inside double quotes.
Use the
re.findall()
function to find all matches in the string and store the result in a variable:
result = re.findall(pattern, your_string)
your_string
is the string you want to split.result
will store the final list of words.
Voila! You now have the desired result:
print(result)
Output:
['this', 'is', 'a test']
Example usage
Here's an example of how you can use this solution:
import re
def split_string_with_quotes(your_string):
pattern = r'("[^"]*"|\S+)'
result = re.findall(pattern, your_string)
return result
input_string = 'this is "a test"'
output_list = split_string_with_quotes(input_string)
print(output_list)
Output:
['this', 'is', 'a test']
Final thoughts
Splitting a string by spaces while preserving quoted substrings is a common problem, and now you have an easy solution. 😄
Remember, this solution assumes that there are no quotes within quotes, as mentioned in the PS of the question. If your application requires handling such cases, additional logic may be needed.
Now it's your turn! Give it a try and let me know if this solution worked for you. If you have any questions or alternative solutions, feel free to share them in the comments below. Happy coding! 🚀