Split a Pandas column of lists into multiple columns
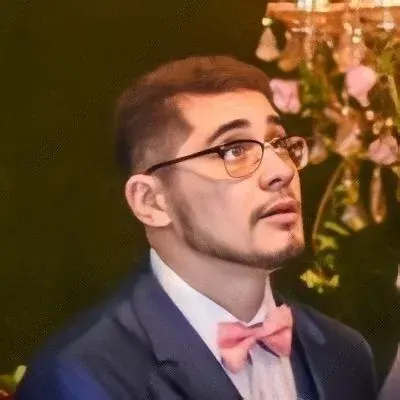
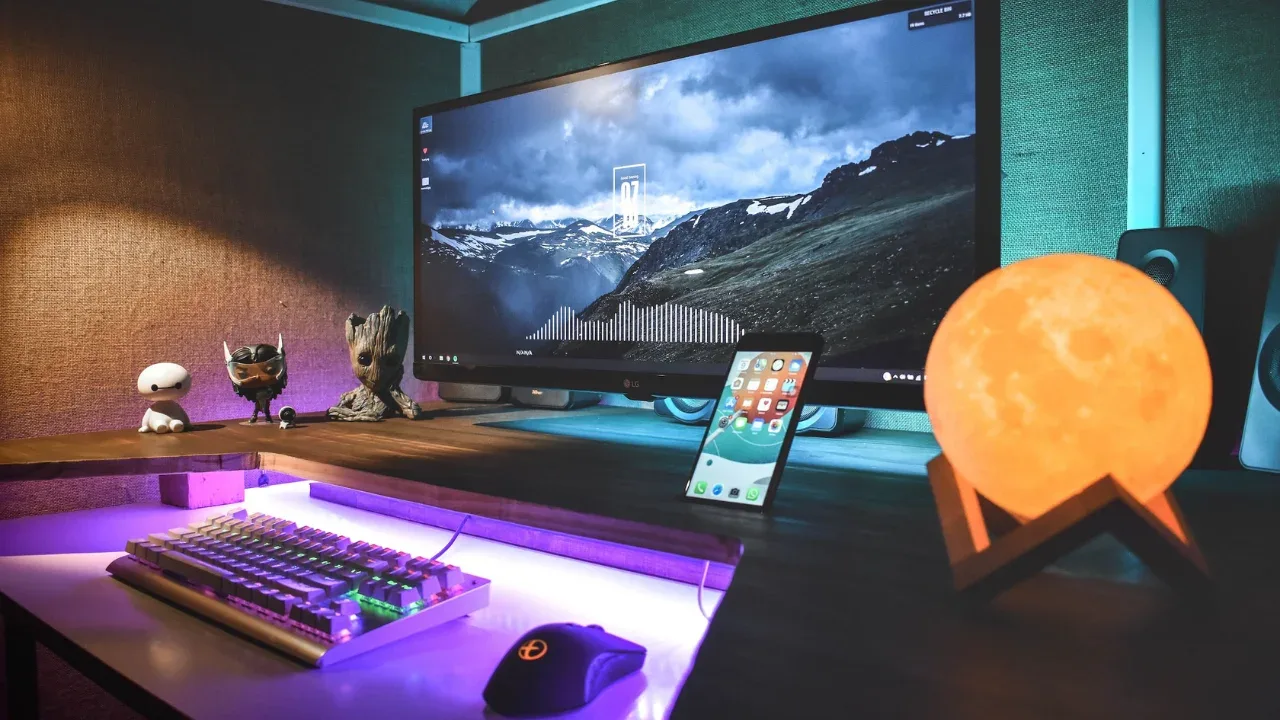
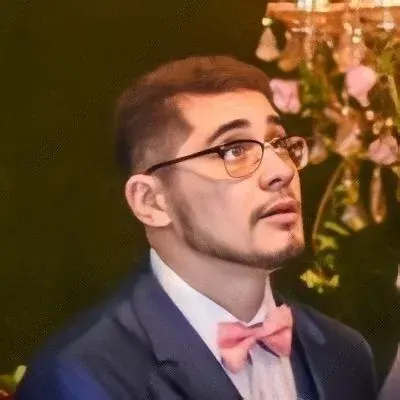
Splitting a Pandas Column of Lists into Multiple Columns
If you're working with a Pandas DataFrame and have a column that contains lists, you might find yourself in a situation where you need to split that column into multiple columns. This can be useful for various reasons, such as improving data clarity or performing further analysis.
In this blog post, we'll address the common issue of splitting a Pandas column of lists into multiple columns. We'll provide easy-to-understand solutions and guide you step-by-step towards achieving the desired result.
The Problem
Let's start by examining the problem at hand. Suppose we have a Pandas DataFrame with a single column called "teams." Each row in this column contains a list of team names:
import pandas as pd
df = pd.DataFrame({"teams": [["SF", "NYG"] for _ in range(7)]})
teams
0 [SF, NYG]
1 [SF, NYG]
2 [SF, NYG]
3 [SF, NYG]
4 [SF, NYG]
5 [SF, NYG]
6 [SF, NYG]
Your goal is to split this column of lists into two separate columns, resulting in a DataFrame that looks like this:
team1 team2
0 SF NYG
1 SF NYG
2 SF NYG
3 SF NYG
4 SF NYG
5 SF NYG
6 SF NYG
Solution
To solve this problem, we can use the apply
method in conjunction with the pd.Series
constructor.
Here's the code to accomplish this:
df[['team1', 'team2']] = df['teams'].apply(pd.Series)
Let's break down this code:
df['teams']
selects the column we want to split..apply(pd.Series)
applies thepd.Series
constructor to each element in the selected column. This constructor automatically splits the list into separate columns.df[['team1', 'team2']]
creates two new columns in the original DataFrame, labeled as "team1" and "team2."
By running this code, we can obtain the desired result:
team1 team2
0 SF NYG
1 SF NYG
2 SF NYG
3 SF NYG
4 SF NYG
5 SF NYG
6 SF NYG
See how easy it is?
Conclusion
Splitting a Pandas column of lists into multiple columns is a common task, and with the solution explained in this blog post, you can effortlessly achieve the desired result. By using the apply
method and the pd.Series
constructor, you can efficiently split the lists and create new columns without much hassle.
Now that you know how to solve this problem, try applying this technique to your own data frames and explore the possibilities it offers.
Have you ever encountered this issue or faced any other difficulties while working with Pandas? Let us know in the comments below! We'd love to hear about your experiences and help you find solutions.
Don't forget to share this blog post with your fellow data enthusiasts. Sharing is caring, and together we can make data manipulation easier for everyone! 😊