Sorting arrays in NumPy by column
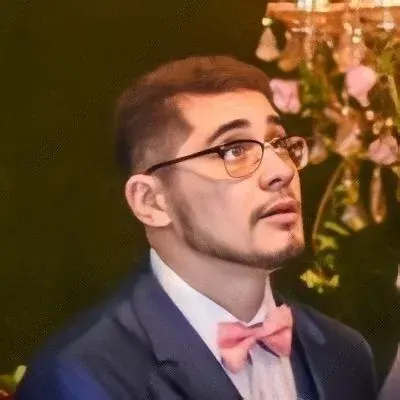
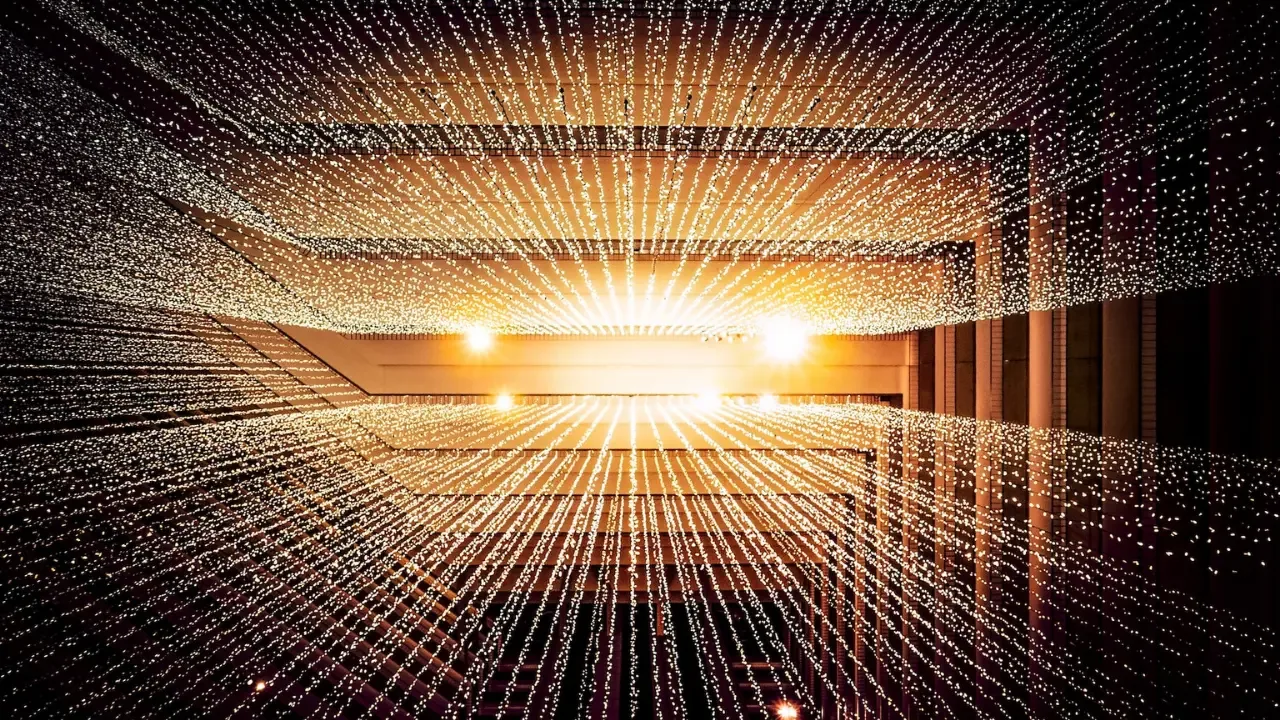
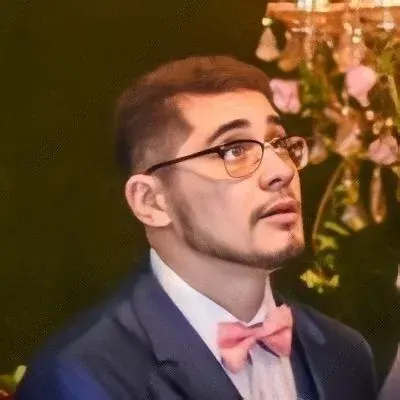
Sorting Arrays in NumPy by Column: A Simple Guide
Sorting arrays by column in NumPy can be a bit tricky if you're not familiar with the right techniques. But don't worry, we're here to help you out! In this guide, we'll cover a common issue: how to sort a NumPy array by its nth column. We'll provide you with easy solutions and even throw in some cool emoji tricks. 😎 So let's get started! 👊
The Problem
Let's say we have the following NumPy array called a
:
a = np.array([[9, 2, 3],
[4, 5, 6],
[7, 0, 5]])
Our goal is to sort the rows of a
by the second column, resulting in the following array:
array([[7, 0, 5],
[9, 2, 3],
[4, 5, 6]])
The Solution
To sort a NumPy array by a specific column, we can use the np.argsort()
function combined with fancy indexing. Here's how you can do it:
sorted_indices = np.argsort(a[:, n])
sorted_array = a[sorted_indices]
In this case, n
represents the index of the column you want to sort by. In our example, we want to sort by the second column (index 1), so n
would be 1.
Let's break it down step by step and see how this solution works:
a[:, n]
selects the entiren
th column of the arraya
. By using fancy indexing, we create a separate array with just the values from that column.np.argsort()
returns the indices that would sort the selected column in ascending order. It essentially gives us a sorted list of indices based on the values in the column.sorted_indices
now contains the indices in the order that would sort the selected column. We can use these indices to rearrange the rows of the original array.Finally,
sorted_array = a[sorted_indices]
creates a new array where the rows are sorted based on the selected column. Voilà! 🎉
Example Usage
To make it crystal clear, let's see our solution in action with the example provided earlier:
import numpy as np
a = np.array([[9, 2, 3],
[4, 5, 6],
[7, 0, 5]])
n = 1 # The second column (index 1)
sorted_indices = np.argsort(a[:, n])
sorted_array = a[sorted_indices]
print(sorted_array)
Output:
[[7 0 5]
[9 2 3]
[4 5 6]]
Easy peasy, right? You've just mastered the art of sorting arrays in NumPy by column! Now you can impress your friends and colleagues with your newfound knowledge. 😉
The Call-to-Action
If you found this guide helpful, be sure to share it with your fellow tech enthusiasts and NumPy users. Spread the knowledge, share the love! ❤️ And if you have any other NumPy-related questions or topics you'd like us to cover, let us know in the comments below. We'd be more than happy to help you out.
Remember, learning is a journey, and we're here to make it a fun and exciting one! Stay curious, keep experimenting, and keep shining like the bright 🌟 tech star that you are! ✨