Simplest async/await example possible in Python
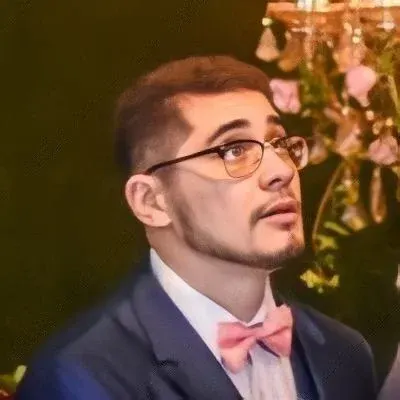
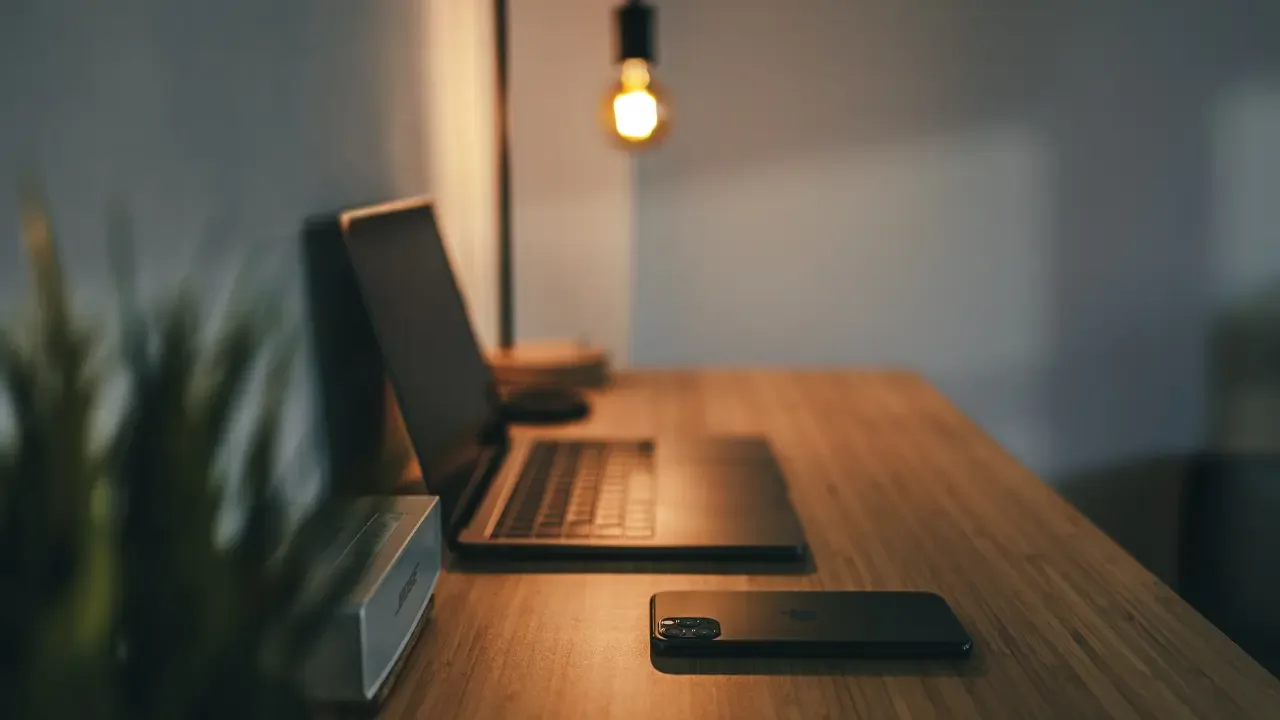
The Simplest Async/Await Example in Python 🐍
Are you tired of diving into complex explanations of async/await in Python? Do you want a minimal example that shows you how these keywords work without the noise of other asyncio functions? Look no further! In this blog post, we'll walk you through a super simple example that demonstrates the power of async/await in just a few lines of code. Let's get started! 💪
The Problem 😫
Many resources available on the internet provide examples of async/await in Python, but they often involve additional functions from the asyncio
library, making it difficult to grasp the core concepts behind these keywords. Our goal is to provide you with the most minimalist async/await example that showcases how they work, using just the two keywords and the necessary code to run the async loop.
The Solution 🚀
To achieve our goal, let's consider the following example:
import asyncio
async def async_foo():
print("async_foo started")
await asyncio.sleep(5)
print("async_foo done")
async def main():
await async_foo() # directly await async_foo()
print('Do some actions 1')
await asyncio.sleep(5)
print('Do some actions 2')
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
In this example, we have two async functions: async_foo()
and main()
. The async_foo()
function prints a message, awaits for 5 seconds using asyncio.sleep()
, and then prints another message. On the other hand, the main()
function awaits for async_foo()
to complete, performs some actions, awaits for 5 seconds again, and finally performs more actions.
The key idea here is the await
keyword. By directly await
-ing the async_foo()
function in the main()
function, we can ensure that it runs within the async loop and behaves asynchronously without the need for the ensure_future()
function.
Explanation 📝
When we execute the code above, several things happen:
The
main()
function is scheduled to run within the event loop usingloop.run_until_complete(main())
.The
async_foo()
function is called within themain()
function.The
async_foo()
function starts its execution and prints "async_foo started".The
await asyncio.sleep(5)
line withinasync_foo()
suspends the execution ofasync_foo()
and allows other tasks within the event loop to run.While
async_foo()
is waiting, the program proceeds to execute the next line withinmain()
."Do some actions 1" is printed.
The
await asyncio.sleep(5)
line withinmain()
suspends the execution ofmain()
for 5 seconds."Do some actions 2" is printed.
After 5 seconds have passed,
async_foo()
resumes execution and prints "async_foo done".The event loop completes, and the program terminates.
Conclusion 🎉
Congratulations! 🎉 You've just created and understood the simplest async/await example in Python. You don't need any fancy ensure_future()
function or complicated asyncio logic. By directly await
-ing an async function within your main function, you can leverage the power of asynchronous programming.
Now that you grasp the core concepts behind async/await, don't stop here! Explore more about asyncio and the vast possibilities it offers. Share your experience, ask questions, and engage with the Python community.
Keep coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
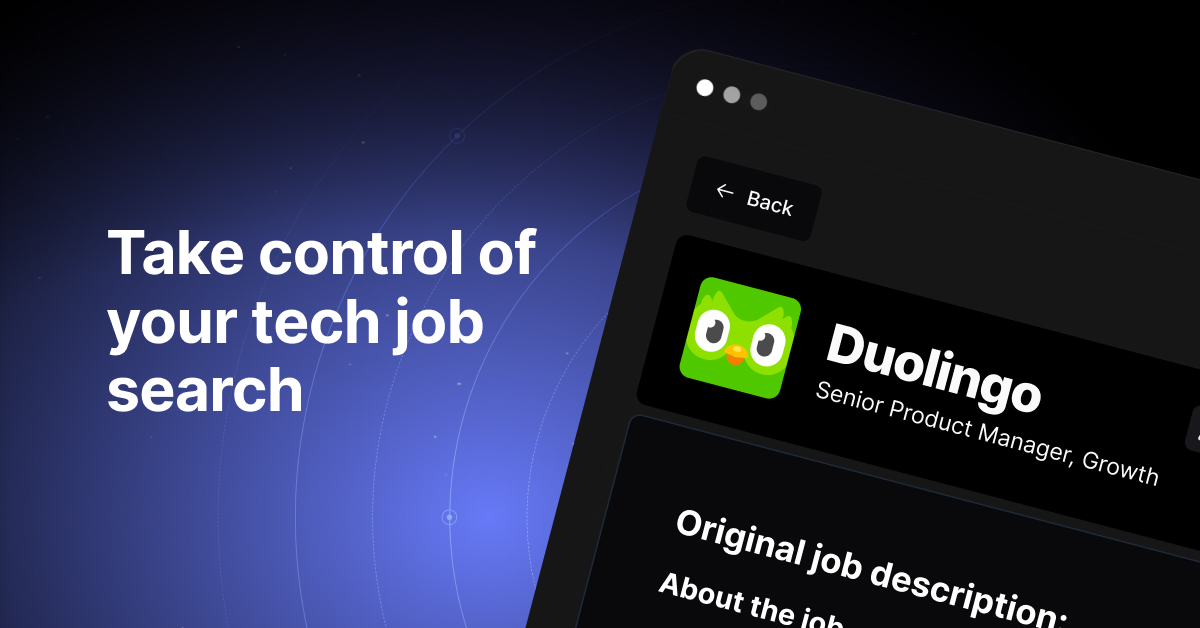