Shuffle DataFrame rows
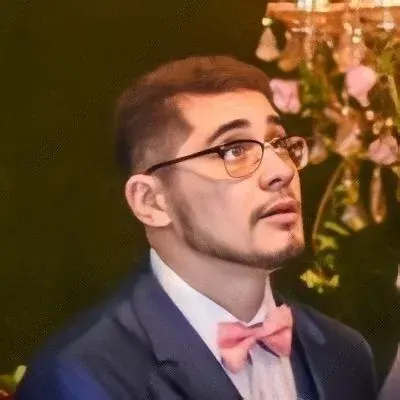
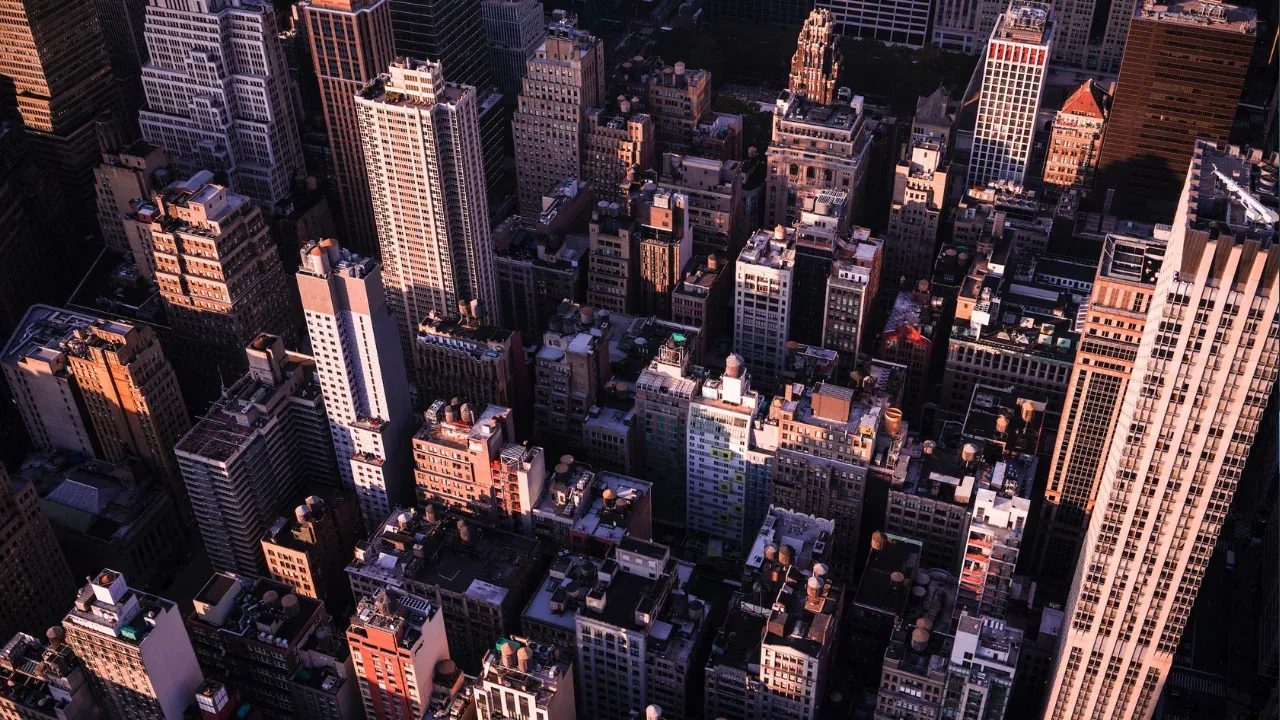
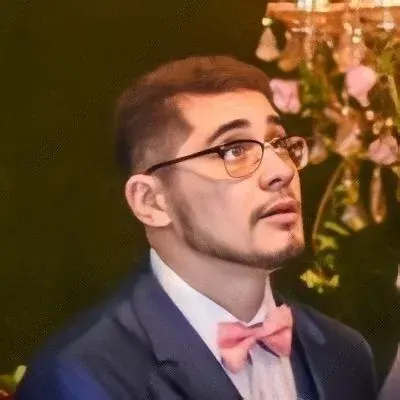
🔀 How to Shuffle DataFrame Rows? 🔀
So you want to shuffle the rows of your DataFrame and mix all the different types together? No worries, I've got you covered! Here's a step-by-step guide to help you achieve exactly that. Let's dive in! 🏊♀️
1. Import the necessary libraries
Before we start shuffling, let's import the libraries we'll be using. In this case, we'll need the pandas
library:
import pandas as pd
2. Create your DataFrame
Assuming you already have your DataFrame loaded from a CSV file, as mentioned in the context, make sure it is stored in a variable. In this example, we'll call it df
.
3. Shuffle the rows
Pandas provides a handy function called sample()
that we can use to shuffle our DataFrame rows. By using the frac
parameter with a value of 1, we can achieve a complete shuffle. Here's how you can do it:
shuffled_df = df.sample(frac=1).reset_index(drop=True)
Let's break it down:
sample(frac=1)
means we're sampling the entire DataFrame, resulting in a complete shuffle.reset_index(drop=True)
resets the index of the shuffled DataFrame, so it doesn't retain the old index from the original DataFrame.
4. Verify the shuffled DataFrame
To make sure the shuffling worked as expected, let's take a look at our updated DataFrame by printing it out:
print(shuffled_df)
5. Save the shuffled DataFrame
If you want to save the shuffled DataFrame into a new CSV file, you can use the to_csv()
function:
shuffled_df.to_csv('shuffled_data.csv', index=False)
Make sure to replace 'shuffled_data.csv'
with the desired file name.
That's it! You've successfully shuffled your DataFrame rows and mixed all different types together. 🎉
Now it's time to put this knowledge into action! Go ahead and give it a try in your own code. If you have any questions or face any issues, feel free to leave a comment below. Happy shuffling! 🕺
📣 Share Your Experience!
Have you ever needed to shuffle DataFrame rows? How did you go about it? Share your experience and let us know if you found this guide helpful. We'd love to hear from you! 💬