Set value for particular cell in pandas DataFrame using index
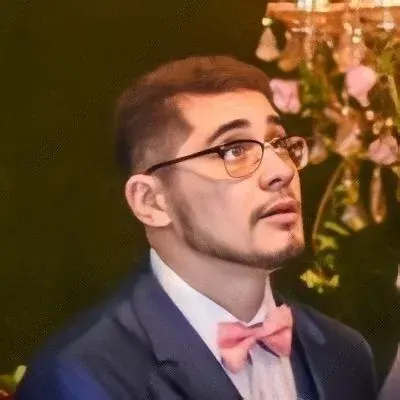
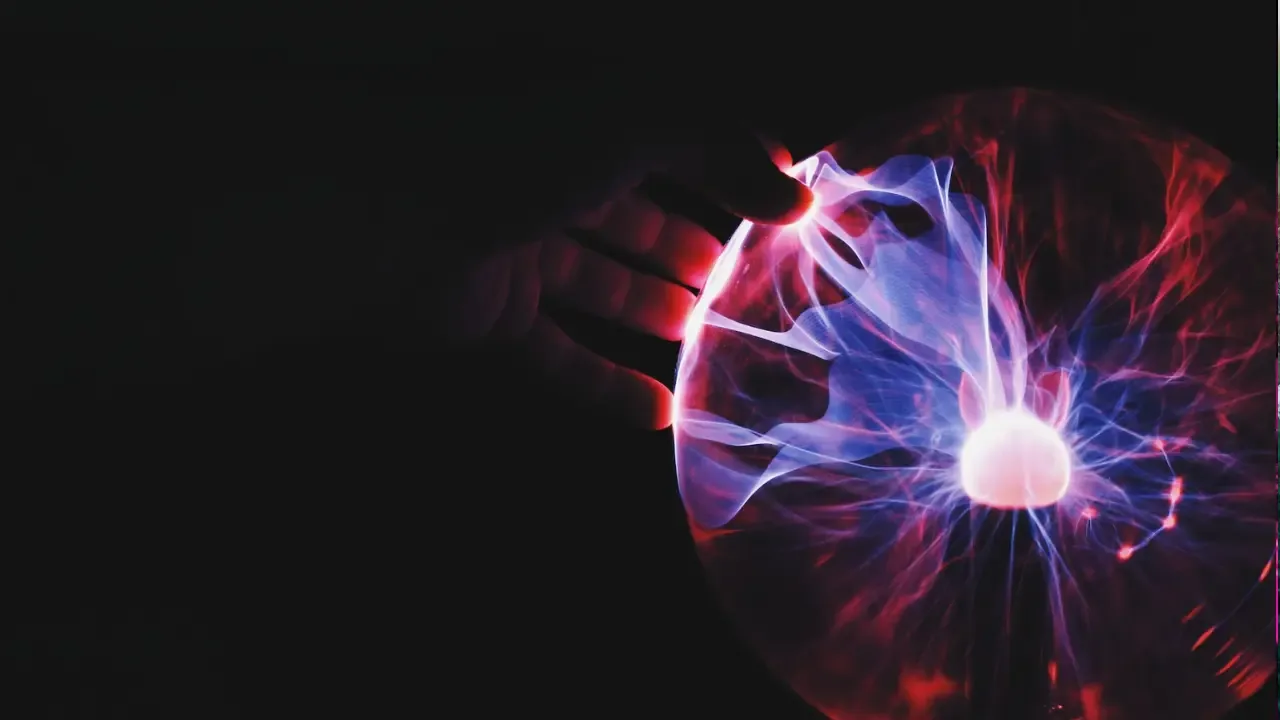
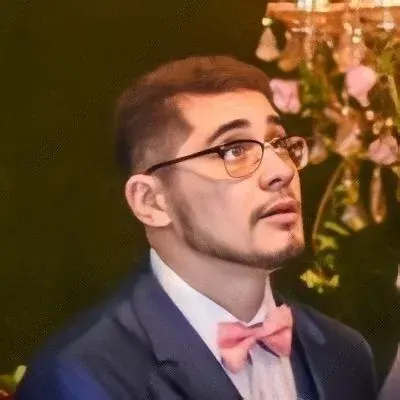
How to Set a Value for a Particular Cell in a Pandas DataFrame using Index 🐼
Have you ever faced the problem of assigning a value to a specific cell in a Pandas DataFrame, only to find that the DataFrame remains unchanged? 😩 Don't worry, you're not alone! It's a common issue that can be a bit tricky to solve. But fear not, because we're here to help you out! 🙌
The Problem 🤔
Let's start by looking at the context of the problem. Suppose you have created a Pandas DataFrame like this:
import pandas as pd
df = pd.DataFrame(index=['A','B','C'], columns=['x','y'])
And you have this initial DataFrame:
x y
A NaN NaN
B NaN NaN
C NaN NaN
Now, you want to assign a value to a specific cell, for example, the cell in row C
and column x
. You expect to get the following result:
x y
A NaN NaN
B NaN NaN
C 10 NaN
You try the following code:
df.xs('C')['x'] = 10
But to your surprise, the contents of df
remain unchanged, and the DataFrame still contains only NaN
values. 😱
The Solution 💡
The issue here is that when you access a specific row using the xs()
method and try to assign a value to a cell, Pandas creates a copy of that row instead of modifying the original DataFrame. Thus, your assignment doesn't affect the original DataFrame.
To overcome this problem, you can use the at
accessor or the loc
indexer to set the value for a specific cell. Let's explore both approaches:
Using the at
Accessor 🔒
The at
accessor allows you to access a single value for a row/column pair by using label-based indexing. Here's how you can use it to set a value for a particular cell:
df.at['C', 'x'] = 10
This code directly modifies the original DataFrame, and you will get the desired result:
x y
A NaN NaN
B NaN NaN
C 10 NaN
Using the loc
Indexer 📍
The loc
indexer can also be used to set a value for a specific cell in a Pandas DataFrame. Here's how you can achieve it:
df.loc['C', 'x'] = 10
Again, this code modifies the original DataFrame and yields the expected result.
Conclusion 🏁
By using either the at
accessor or the loc
indexer, you can easily set a value for a particular cell in a Pandas DataFrame, ensuring that the changes are applied to the original DataFrame. No more frustration with imaginary assignments!
We hope this guide has helped you solve your problem efficiently. Now, go ahead and conquer those DataFrames like a pro! If you have any other questions or suggestions, feel free to leave a comment below. Happy coding! 💻✨