Serialising an Enum member to JSON
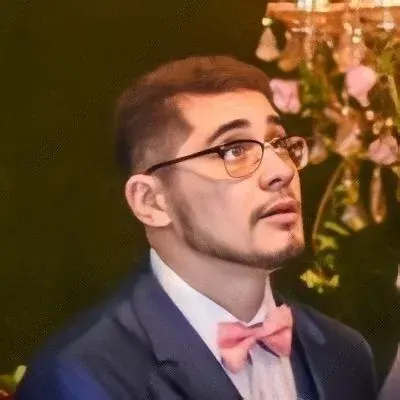
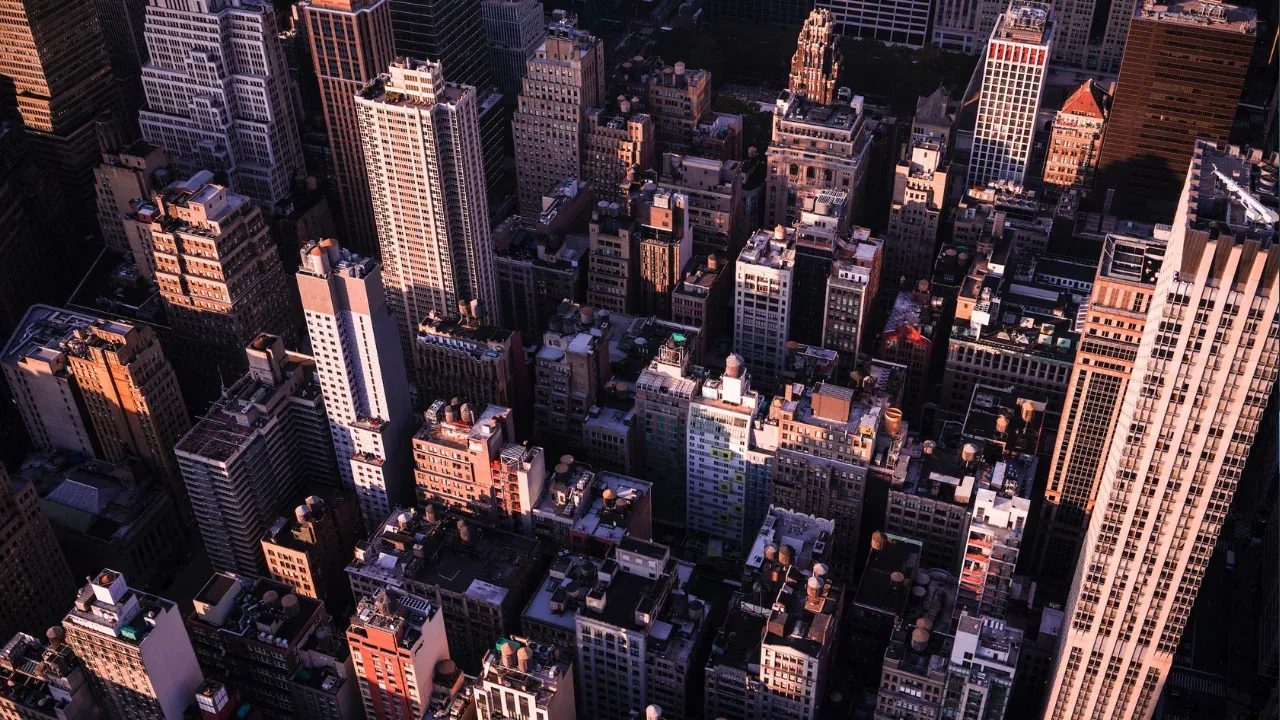
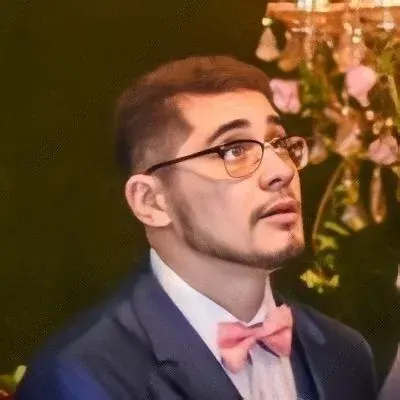
📝 Title: Serialising an Enum member to JSON: A Complete Guide
Welcome, tech enthusiasts! Today, we'll dive into the world of serialising Enum members to JSON in Python 🐍. Have you ever encountered the pesky error message, "TypeError: <Status.success: 0> is not JSON serializable"? Fear not! In this post, we'll unravel the mystery and provide you with easy solutions to tackle this problem. Let's get started! 💪
Understanding the Problem
When attempting to serialise an Enum member to JSON using the json.dumps()
function, you might encounter the TypeError mentioned earlier. This occurs because the default JSON encoder does not recognize the Enum type and its members. 😔
Easy Solution #1: Implement a Custom JSON Encoder
One solution is to create a custom JSON encoder class that extends the json.JSONEncoder
class. This encoder will be responsible for serialising the Enum members to JSON. Here's an example:
from enum import Enum
import json
class EnumEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, Enum):
return obj.value
return super().default(obj)
# Usage example:
class Status(Enum):
success = 0
json.dumps(Status.success, cls=EnumEncoder)
Nice job! By using the cls
parameter of the json.dumps()
function, we pass our custom EnumEncoder
class for serialisation, and voilà! 🌟 The Enum member will now be successfully serialised to JSON.
Easy Solution #2: Implement a Custom Encoder Function
Another approach is to define a custom encoder function to convert Enum members to their values. Here's how you can do it:
from enum import Enum
import json
def enum_converter(obj):
if isinstance(obj, Enum):
return obj.value
# Usage example:
class Status(Enum):
success = 0
json.dumps(Status.success, default=enum_converter)
You're doing great! By specifying the default
parameter in json.dumps()
, we provide our custom enum_converter
function to handle the serialisation of Enum members. Now, you can generate JSON without any errors! 🎉
Call-to-Action: Share Your Success Stories!
Congratulations, tech whizzes! You've learned two easy solutions to serialise Enum members to JSON in Python. 🚀 Now, it's time to put your knowledge into practice and share your success stories with us! Have you encountered any other issues during JSON serialisation? Let us know in the comments below and join the discussion. 💬
If you found this post helpful, don't forget to share it with your fellow developers. Together, we can conquer the world of Python Enum serialisation to JSON!
Happy coding! 😄👩💻👨💻