Separation of business logic and data access in django
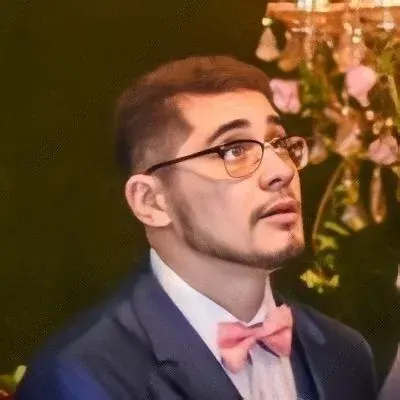
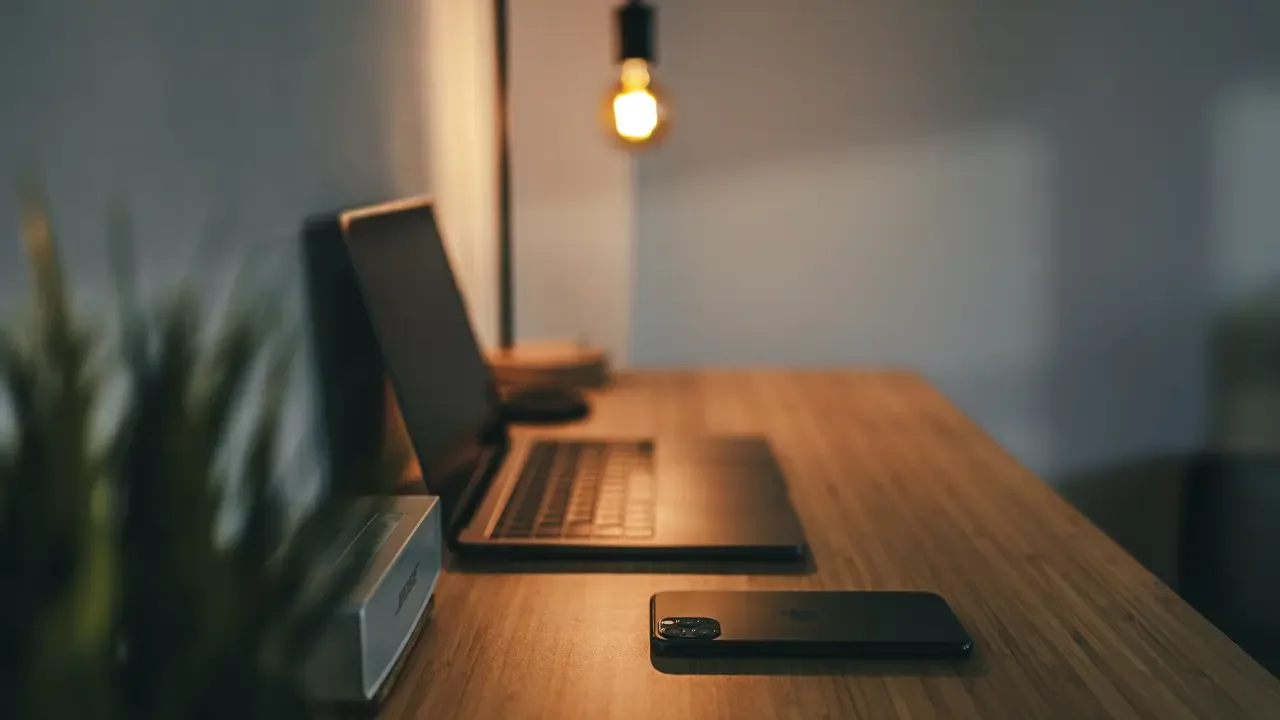
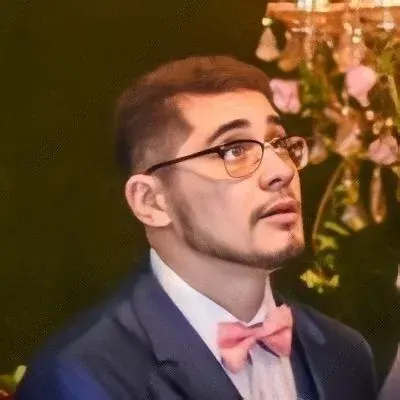
Separation of Business Logic and Data Access in Django
Are you tired of seeing a cluttered and confusing models.py
file in your Django project? Do you find it frustrating that your model, which is supposed to handle database-related tasks, is also responsible for sending emails and interacting with APIs? If you're nodding your head in agreement, then this blog post is for you!
Understanding the Problem
Let's start by addressing the issues you mentioned:
Mixing Responsibilities: It's not ideal for your models to perform tasks beyond data manipulation. When business logic, such as email sending or API calls, creeps into your models, it becomes harder to maintain and understand your codebase.
Dispersed Business Logic: Placing all your business logic in the view can lead to code duplication and inconsistency. Imagine having multiple ways to create a new instance of a
User
model – this lack of uniformity can make your code harder to control and maintain.Non-Deterministic Models: Over time, your models might evolve to include non-deterministic behaviors and side effects. For example, your
get_present_name()
method started out as a simple property but ended up fetching data from an external service and became non-deterministic in terms of database operations. These unexpected changes can introduce bugs and make debugging a nightmare.
Separating Entities in Your Code
To address these challenges, we need to separate our entities into their respective responsibilities – database level entities and application level entities:
Database Level Entities: These entities handle data-related tasks like storing and retrieving data from the database. Ideally, they should be abstracted from any business logic or external dependencies.
Application Level Entities: These entities house our business logic, focusing on what our application does. They interact with the database level entities and orchestrate the necessary actions.
Implementing Best Practices in Django
Now that we understand the concept, let's discuss the best practices to implement this approach in Django:
1. Use Django's Model-View-Controller (MVC) Pattern
Django follows the MVC pattern, with the models acting as the "M" (model) component. However, to achieve separation of concerns, we can further divide the responsibilities within the models.
2. Divide Models into Database and Application Level Entities
Create two separate directories within your app, namely database
and application
. Place your database level entities, which include models and related database operations, inside the database
directory. On the other hand, house your application level entities, containing business logic and application-specific actions, within the application
directory.
3. Utilize Django's Signals
Django provides a powerful mechanism called signals that allows decoupled applications to get notified when certain actions occur. By utilizing signals, you can move certain functionalities, like sending emails or performing additional actions, out of the models and into separate modules within the application level entities. This keeps your models clean and focused solely on data tasks.
4. Leverage Django's Managers
Django's managers allow you to encapsulate complex queries and database operations at the model level. By utilizing managers, you can keep your database level entities clean and focused on data manipulation while exposing simplified interfaces for your application level entities to interact with.
5. Apply the Single Responsibility Principle
As you refactor your code and separate concerns, keep in mind the Single Responsibility Principle. Each entity or module should have a single responsibility and be focused on doing just that.
Conclusion
By implementing the separation of business logic and data access in your Django project, you can improve the maintainability and understandability of your code while promoting code reusability and consistency. Remember to divide your code into database and application level entities, leverage Django's signals and managers, and follow the Single Responsibility Principle.
Share your thoughts about this approach in the comments below. How do you handle separation of concerns in your Django projects? Let's strive for cleaner, more maintainable code together! 💪🐍