Selecting with complex criteria from pandas.DataFrame
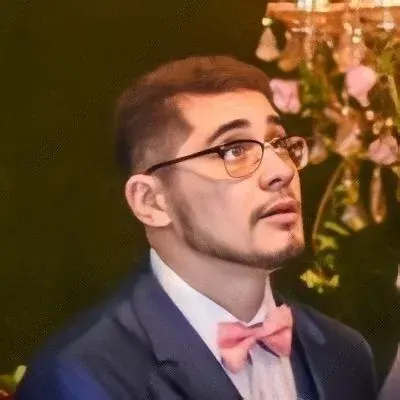
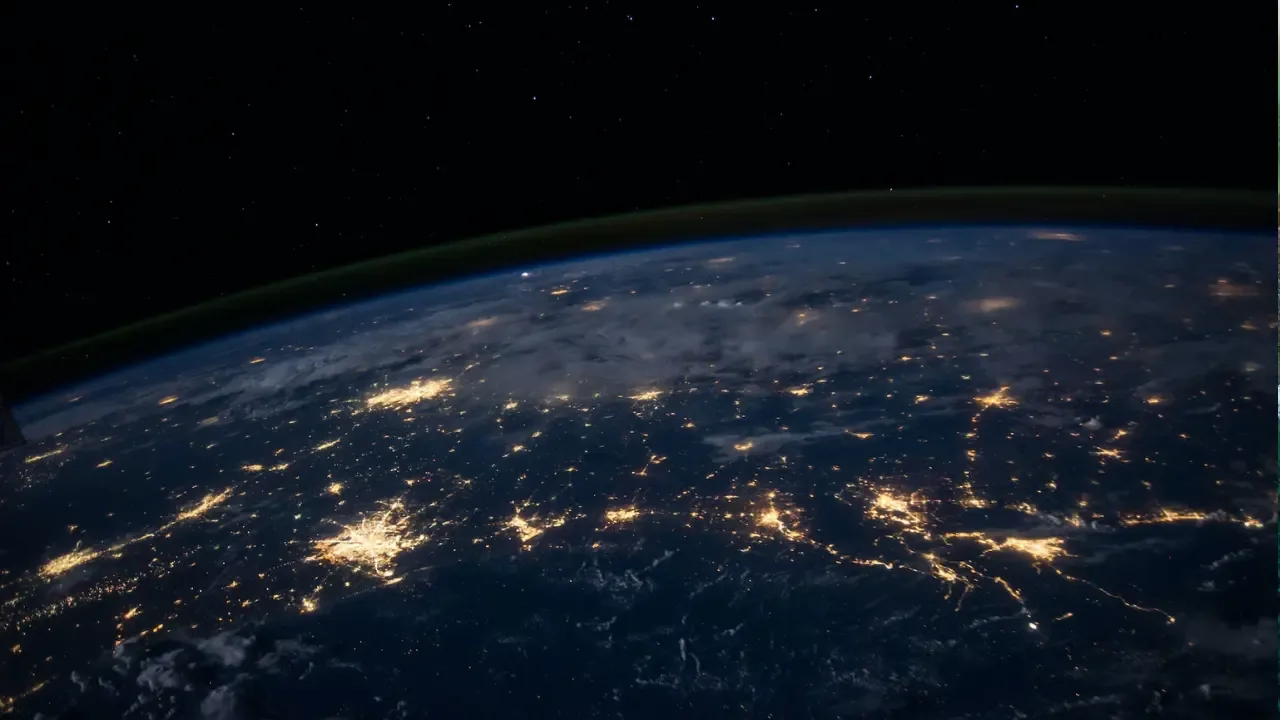
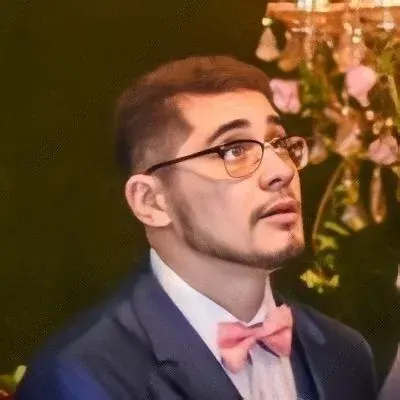
📝 Selecting with Complex Criteria in Pandas: A Complete Guide
Are you struggling with selecting values from a Pandas DataFrame using complex criteria? Look no further, because we've got you covered! In this blog post, we'll tackle the common issue of selecting values from one column based on conditions in other columns. We'll use the powerful methods and idioms of Pandas to make your life easier.
The Scenario
Let's start by setting the context. Imagine you have a simple DataFrame (let's call it df
) with three columns: 'A', 'B', and 'C'. Each column contains randomly generated values. Here's an example:
import pandas as pd
from random import randint
df = pd.DataFrame({'A': [randint(1, 9) for x in range(10)],
'B': [randint(1, 9)*10 for x in range(10)],
'C': [randint(1, 9)*100 for x in range(10)]})
print(df)
The output of this code snippet would be a DataFrame that looks something like this:
A B C
0 3 50 600
1 5 60 400
2 2 90 200
3 7 60 100
4 9 20 300
5 5 40 300
6 1 40 700
7 2 10 600
8 8 30 200
9 2 40 200
The Challenge
Now that we have our DataFrame, let's address the challenge at hand. We want to select values from column 'A' based on the following criteria:
The corresponding values in column 'B' should be greater than 50.
The corresponding values in column 'C' should not be equal to 900.
The Solution
Pandas offers several ways to tackle this problem, so let's explore a couple of easy and efficient solutions:
Solution 1: Using Boolean Indexing
One way to solve this challenge is by using boolean indexing. We can create a boolean mask by applying the specified conditions to the DataFrame. Then, we can use this mask to select the desired values from column 'A'. Here's how you can do it:
mask = (df['B'] > 50) & (df['C'] != 900)
selected_values = df.loc[mask, 'A']
In this code snippet, we create a boolean mask using the conditions df['B'] > 50
and df['C'] != 900
. We then use this mask with the .loc
indexer to select the corresponding values from column 'A'.
Solution 2: Using Query
Another approach is to use the query
method provided by Pandas. With the query
method, we can write our conditions in a more expressive and intuitive way. Here's how you can leverage the query
method to solve our challenge:
selected_values = df.query('(B > 50) & (C != 900)')['A']
In this concise code snippet, we directly use the conditions B > 50
and C != 900
within the query
method. This allows us to easily select the desired values from column 'A'.
The Call-to-Action
Congratulations! You've learned two efficient ways to select values from a Pandas DataFrame based on complex criteria. Now it's time to put your newfound knowledge into practice. Experiment with these solutions on your own data or try applying them to similar problems you encounter in your work.
Don't forget to share your thoughts and experiences with us. We'd love to hear how these solutions have helped you. So go ahead and leave a comment below or share this blog post with your fellow data enthusiasts!
Happy coding! 👩💻💪📊
About the author: This blog post was written by [Your Name]. [Your Name] is a tech writer who is passionate about simplifying complex concepts and making them accessible to everyone. [Your Name] writes regularly on their tech blog, where they explore various topics related to data analysis, programming, and more. Be sure to check out their blog for more informative content!