Selecting a row of pandas series/dataframe by integer index
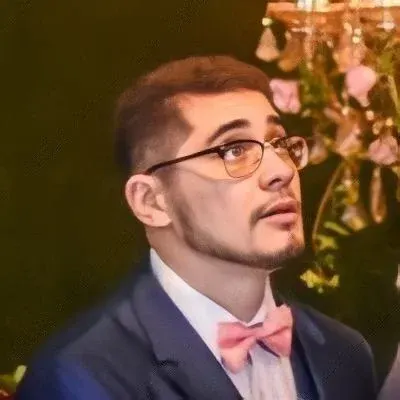
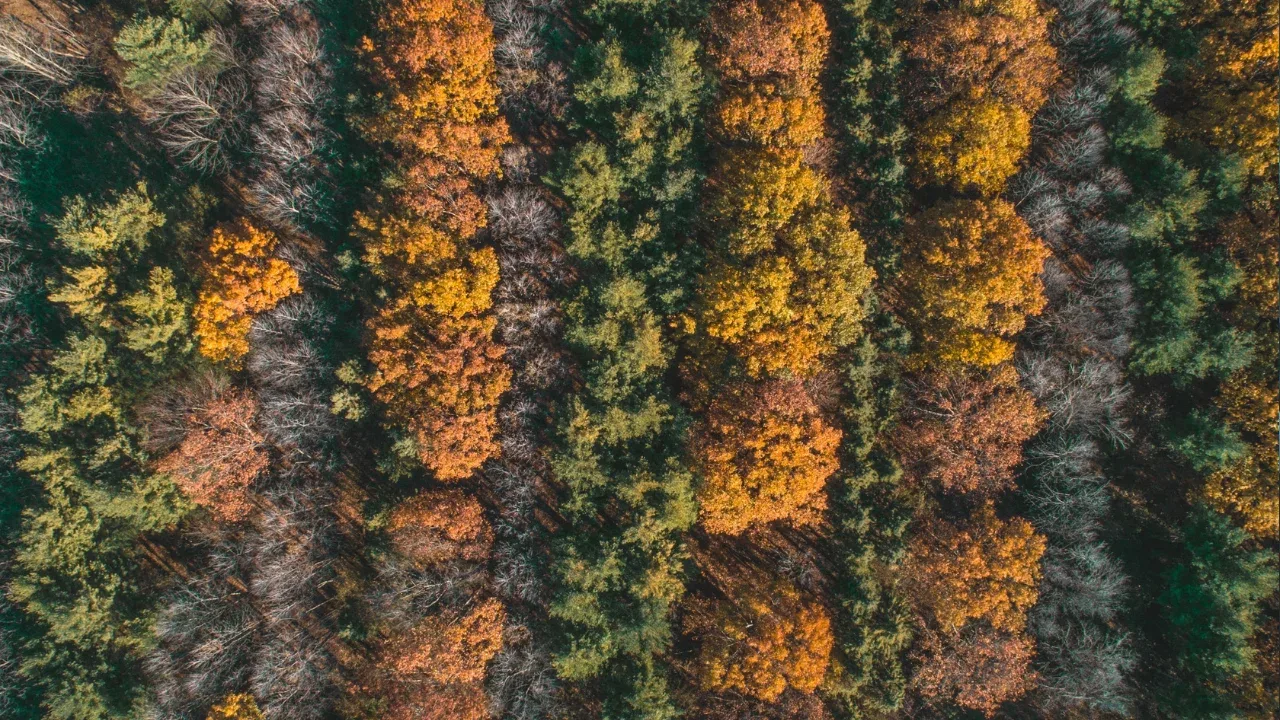
Selecting a Row of Pandas Series/Dataframe by Integer Index
If you've been working with pandas for a while, you may have come across a situation where you wanted to select a single row from a series or dataframe using an integer index. But you might have noticed that using the syntax df[2]
doesn't work, while df.ix[2]
and df[2:3]
both provide the desired result. In this blog post, we'll explore the reasons behind this behavior and provide easy solutions for selecting a row by integer index.
The Confusion
Let's take a quick look at the code example that sparked this question:
In [26]: df.ix[2]
Out[26]:
A 1.027680
B 1.514210
C -1.466963
D -0.162339
Name: 2000-01-03 00:00:00
In [27]: df[2:3]
Out[27]:
A B C D
2000-01-03 1.02768 1.51421 -1.466963 -0.162339
In the above code, df.ix[2]
returns a series representing the third row of the dataframe df
, while df[2:3]
returns a new dataframe containing only that specific row.
It's natural to expect that df[2]
would work in a similar way as df[2:3]
, following the typical Python indexing convention. However, the behavior is different, which leads to confusion and the question at hand.
The Underlying Reason
The key to understanding this behavior lies in the fact that pandas treats row indexing differently from column indexing.
When you use square brackets to select a column by label, such as df['column_name']
, pandas knows that you are referring to a column and provides the expected result. However, when you use square brackets to select a row by integer index, such as df[2]
, pandas doesn't have a consistent way to differentiate between selecting a column and selecting a row.
This ambiguity in indexing notation is the primary reason why df[2]
doesn't work to select a row, while df.ix[2]
or df[2:3]
are valid alternatives.
Easy Solutions
Now that we understand the underlying reason for this behavior, let's explore a couple of easy solutions to select a row by integer index:
Solution 1: Use iloc
One straightforward solution is to use the iloc
indexer, which allows you to select rows and columns by integer location.
To select a single row based on its integer index, you can use the following syntax:
row = df.iloc[2]
print(row)
This will print the desired row as a pandas series.
Solution 2: Convert the Row to a Dataframe
If you prefer to obtain the row as a dataframe rather than a series, you can wrap the row selection in double brackets, like this:
row = df[[2]]
print(row)
This will yield a new dataframe containing just the selected row.
Conclusion
While it may be tempting to expect df[2]
to work for selecting a row by its integer index, pandas' indexing notation has its own quirks and distinctions.
To select a row from a series or a dataframe, you can use the df.ix[2]
or df[2:3]
syntax. Alternatively, you can use the iloc
indexer or wrap the row selection in double brackets to obtain a series or dataframe, respectively.
By understanding the design reasons behind pandas' indexing behavior and knowing these easy solutions, you'll be well-equipped to confidently select rows based on integer indexes in your pandas explorations.
Have you ever encountered this indexing challenge in pandas? How did you overcome it? Share your experiences and tips in the comments below!
👉 Keep exploring pandas with our other blog posts on data manipulation and analysis. Discover new tricks and techniques to level up your data science skills! 👈
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
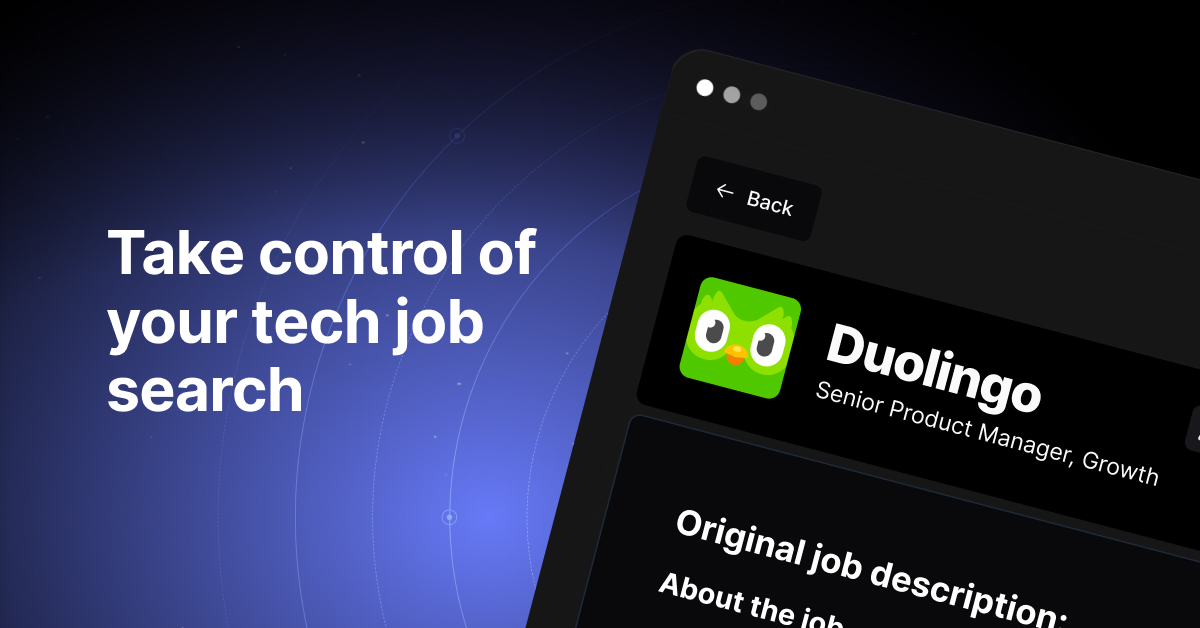