Select rows in pandas MultiIndex DataFrame
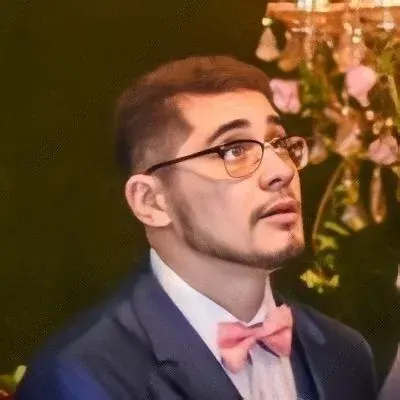
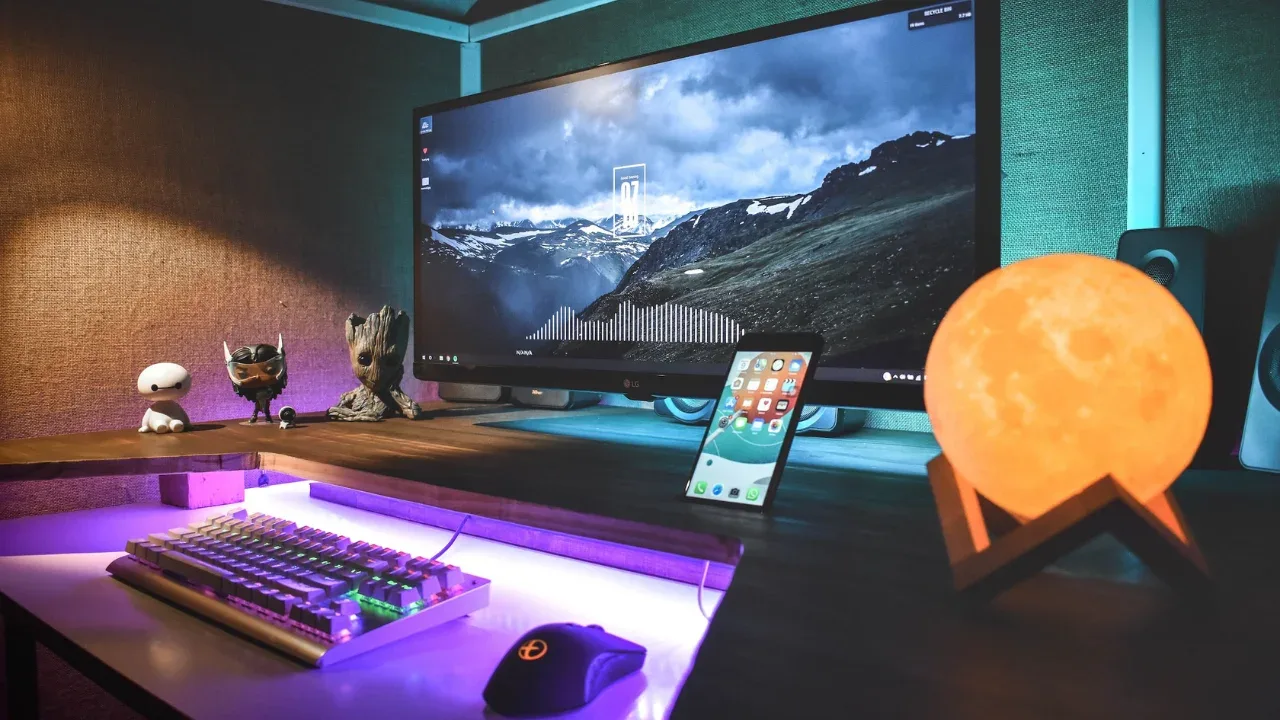
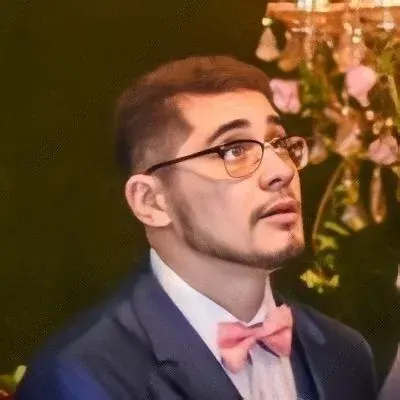
💻 Selecting Rows in Pandas MultiIndex DataFrame
Are you struggling to select and filter rows in a Pandas DataFrame with MultiIndex? Don't worry, we've got you covered! In this guide, we'll address common issues and provide easy solutions to help you understand and master this topic. 🙌
The Example Context
Before we dive into the solutions, let's set the context with an example DataFrame. Imagine we have a DataFrame with a MultiIndex where the first level is labeled "one" and the second level is labeled "two". Here's what it looks like:
mux = pd.MultiIndex.from_arrays([
list('aaaabbbbbccddddd'),
list('tuvwtuvwtuvwtuvw')
], names=['one', 'two'])
df = pd.DataFrame({'col': np.arange(len(mux))}, mux)
col
one two
a t 0
u 1
v 2
w 3
b t 4
u 5
v 6
w 7
t 8
c u 9
v 10
d w 11
t 12
u 13
v 14
w 15
In the following sections, we will address various questions related to selecting rows in this MultiIndex DataFrame.
Question 1: Selecting a Single Item
Let's start with a simple question: how do we select rows with the value "a" in the "one" level? We can achieve this using the .xs()
method:
df.xs('a', level='one')
col
two
t 0
u 1
v 2
w 3
Additionally, we can easily drop the "one" level from the output using the .droplevel()
method:
df.xs('a', level='one').droplevel('one')
col
two
t 0
u 1
v 2
w 3
✨ Tip: If you need to select rows with multiple values, you can pass a list of values as the argument in df.xs(['a', 'b'], level='one')
.
Question 2: Selecting Multiple Values in a Level
Now, let's move on to selecting rows corresponding to multiple items in the "one" level. If we want to select rows with values "b" and "d", we can use the .loc[]
method with a tuple of values:
df.loc[(['b', 'd'],)]
This will give us the following result:
col
one two
b t 4
u 5
v 6
w 7
t 8
d w 11
t 12
u 13
v 14
w 15
Similarly, we can select rows with multiple sub-level values. For example, to select rows with sub-level values "t" and "w", we can use the .loc[]
method with a tuple:
df.loc[(slice(None), ['t', 'w']), :]
This will give us the following result:
col
one two
a t 0
w 3
b t 4
w 7
t 8
d w 11
t 12
w 15
✨ Tip: When selecting multiple values, use a tuple in the .loc[]
method, and slice(None)
to select all values in a level.
Question 3: Slicing a Single Cross Section (x, y)
If we want to retrieve a single row with specific values for the index, also known as a cross section, we can use the .xs()
method. For example, to retrieve the cross section ('c', 'u')
, we can do:
df.xs(('c', 'u'))
This will give us the following result:
col
one
c 9
Question 4: Slicing Multiple Cross Sections [(a, b), (c, d), ...]
To select multiple rows corresponding to different cross sections, we can use the .xs()
method with a list of cross sections. For example, to select the rows with cross sections ('c', 'u')
and ('a', 'w')
, we can do:
df.xs([('c', 'u'), ('a', 'w')])
This will give us the following result:
col
one
c 9
a 3
✨ Tip: When selecting multiple rows, pass a list of cross sections in the .xs()
method.
Question 5: One Item Sliced per Level
How can we retrieve all rows corresponding to "a" in the "one" level or "t" in the "two" level? We can achieve this using the .xs()
method with drop_level=False
:
df.xs('a', level='one', drop_level=False) | df.xs('t', level='two', drop_level=False)
This will give us the following result:
col
one two
a t 0
u 1
v 2
w 3
b t 4
t 8
d t 12
Question 6: Arbitrary Slicing
If we want to slice specific cross sections, such as selecting rows with sub-levels "u" and "v" for "a" and "b", and rows with sub-level "w" for "d", we can use the .loc[]
method with a list of tuples:
df.loc[[('a', 'u'), ('a', 'v'), ('b', 'u'), ('b', 'v'), ('d', 'w')]]
This will give us the following result:
col
one two
a u 1
v 2
b u 5
v 6
d w 11
w 15
Question 7: Filtering by Numeric Inequality on Individual Levels of the MultiIndex
In this unique setup, we have a numeric level in our MultiIndex. To filter rows where values in the "two" level are greater than 5, we can use the .loc[]
method with a boolean condition:
df2.loc[df2.index.get_level_values('two') > 5]
This will give us the following result:
col
one two
b 7 4
9 5
c 7 10
d 6 11
8 12
8 13
6 15
💡 Tip: Use the .get_level_values()
method to access and filter values in a specific level of the MultiIndex.
That's it! We've covered various common scenarios and provided easy solutions to tackle the challenges of selecting and filtering rows in a Pandas MultiIndex DataFrame. We hope this guide has been helpful in your data analysis journey. 🚀
Now it's your turn! Try out these solutions with your own data and feel free to share your thoughts and experiences in the comments below. Let us know if you have other questions or if there are any specific topics you would like us to cover next. Keep coding and stay curious! 👩💻👨💻