Saving UTF-8 texts with json.dumps as UTF-8, not as a \u escape sequence
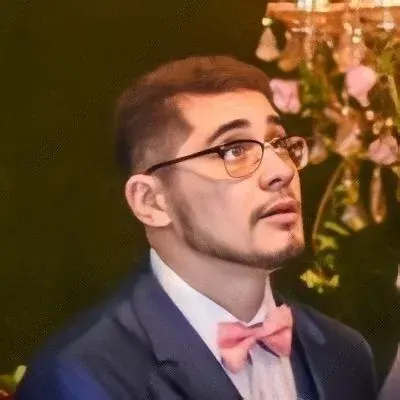
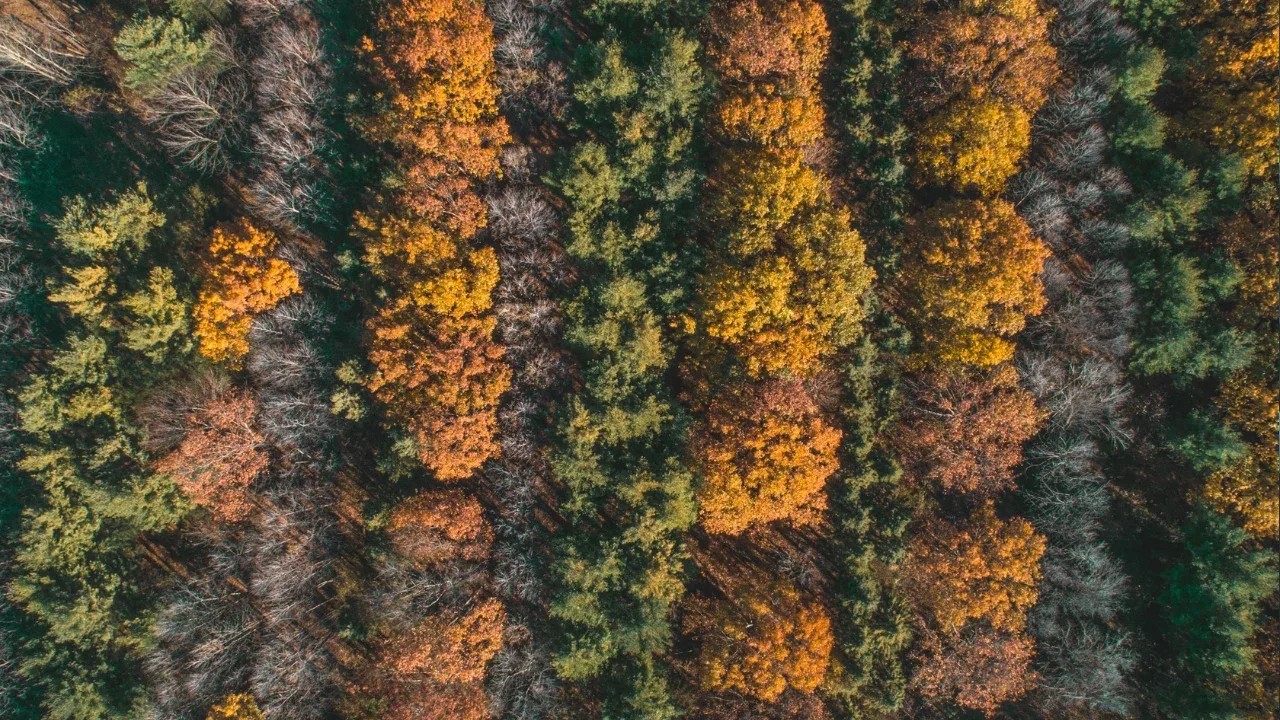
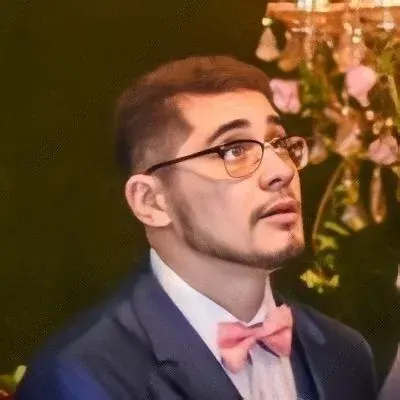
📝 Blog Post: Saving UTF-8 Texts with json.dumps
Do you find it frustrating when your JSON dumps include \u
escape sequences instead of human-readable UTF-8 characters? Well, you're not alone! Many developers face this issue when they need to verify or edit text files with JSON dumps. But fear not, because in this blog post, we're going to address this problem head-on and provide you with easy solutions. Let's dive in! 💪
Understanding the Issue
The problem lies in how the json.dumps
function handles UTF-8 texts. By default, it escapes non-ASCII characters using \uXXXX
notation, which can be cumbersome and hard to read for us humans. Let's take a look at an example to understand this better:
import json
json_string = json.dumps("ברי צקלה")
print(json_string)
Output:
"\u05d1\u05e8\u05d9 \u05e6\u05e7\u05dc\u05d4"
As you can see, the output contains escape sequences instead of the actual UTF-8 characters. This can be a real pain when you're dealing with large JSON files or when you want to manually verify the data.
The Solution: Ensuring UTF-8 JSON Strings
Fortunately, there's a straightforward solution to this problem. The json.dumps
function provides an option to specify the encoding format. To save UTF-8 texts as UTF-8 in the JSON file, you can use the ensure_ascii=False
parameter. Let's modify our previous example to include this fix:
import json
json_string = json.dumps("ברי צקלה", ensure_ascii=False)
print(json_string)
Output:
"ברי צקלה"
Hooray! 🎉 Now our JSON string contains the actual UTF-8 characters instead of escape sequences. It's so much easier to read and work with, especially for your users who might need to verify or edit text files with JSON dumps.
A Better Approach: Writing to a File
While printing the JSON string is useful for demonstration purposes, it's often more practical to write the JSON data to a file. Let's see how we can achieve that:
import json
data = "ברי צקלה"
with open("output.json", "w", encoding="utf-8") as f:
json.dump(data, f, ensure_ascii=False)
print("Data saved to output.json")
In this example, we're opening a file called "output.json" in write mode and specifying the encoding as UTF-8. Then, we're using the json.dump
function to write our data to the file with the ensure_ascii=False
parameter. Now you have a JSON file with human-readable UTF-8 characters!
Your Turn to Try
Now that you've learned the easy solutions to saving UTF-8 texts with json.dumps
, it's time to put your new knowledge into practice. Try it out in your own projects and see the difference it makes. Don't forget to share your results with us! We'd love to hear about your experiences. 😊
Wrapping Up
In this blog post, we addressed the common issue of saving UTF-8 texts with json.dumps
as \u
escape sequences instead of human-readable characters. We provided easy solutions to ensure UTF-8 JSON strings, both for printing and writing to files. Now you can confidently work with JSON dumps and empower your users with more readable data.
If you found this article helpful, or if you have any questions or suggestions, please let us know in the comments below. Happy coding! 💻✨