Running shell command and capturing the output
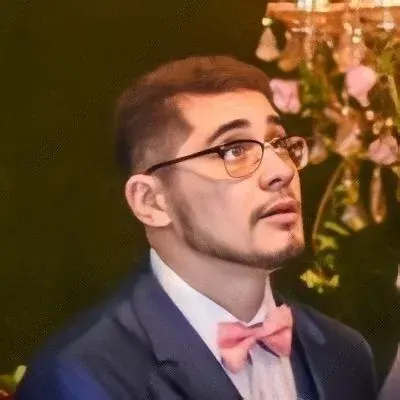
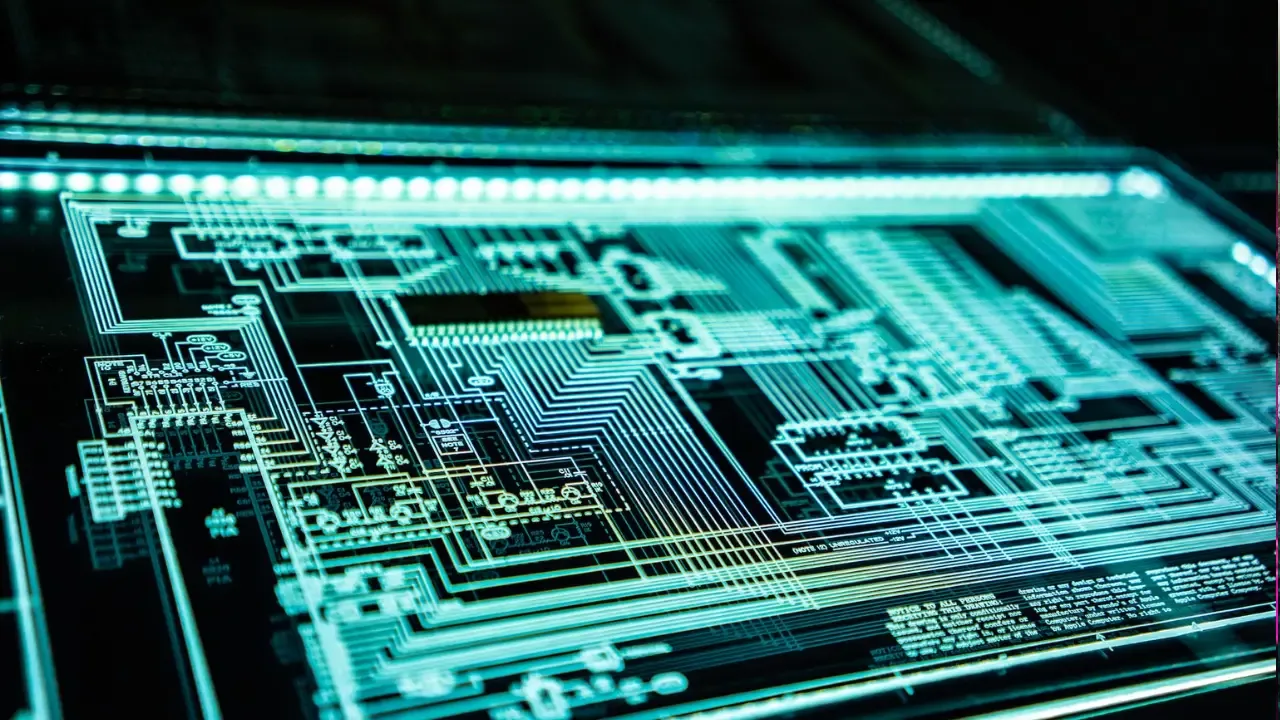
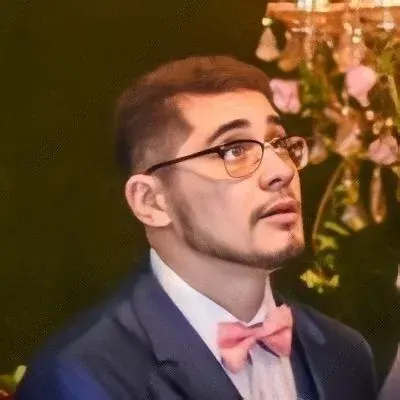
📝 Blog Post: Running Shell Command and Capturing the Output
Are you tired of running shell commands in your code and struggling to capture their output? 😩 Look no further! In this blog post, we will explore a simple and easy solution to execute shell commands and retrieve their output as a string. 💪🔍
The Challenge: Capturing Shell Command Output
Imagine you need to write a function that executes a shell command and returns its output as a string, regardless of whether it's an error or success message. 🤔 You want the same result that you would have obtained by running the command directly in the command line. But how can you achieve this in your code? Let's find out! 🚀
The Solution: Running Shell Commands with Python
To accomplish this, we can leverage the power of Python's subprocess
module. 🐍 In particular, we will use the subprocess.check_output()
function, which allows us to run a shell command and capture its output as a string.
Here's how you can implement the run_command()
function:
import subprocess
def run_command(cmd):
try:
output = subprocess.check_output(cmd, shell=True)
return output.decode().strip()
except subprocess.CalledProcessError as e:
return e.output.decode().strip()
Let's break down what's happening in this code:
We import the
subprocess
module, which provides the necessary tools to run shell commands.The
run_command()
function accepts acmd
argument, which represents the shell command we want to execute.Inside the function, we use
subprocess.check_output()
to run the command and capture its output.If the command successfully executes, we decode the output and strip any leading or trailing whitespace (using
decode().strip()
), and return it as a string.If the command fails, raising a
subprocess.CalledProcessError
exception, we capture the error output, decode it, strip any whitespace, and return it as a string.
Here's an example of using the run_command()
function with the given mysqladmin
command from the question:
print(run_command('mysqladmin create test -uroot -pmysqladmin12'))
# Output: mysqladmin: CREATE DATABASE failed; error: 'Can't create database 'test'; database exists'
And just like that, you can execute shell commands and capture their output with ease! 😎
Wrapping Up and Taking Action
Running shell commands and capturing their output is no longer a headache! 🙌 We've explored an easy-to-implement solution using Python's subprocess
module. Now it's time for you to try it yourself! Use this newfound knowledge to handle shell commands in your code more effectively. 🚀
Have you encountered any challenges while capturing shell command output? Do you have alternative solutions or questions? Don't hesitate to share them in the comments below! Let's discuss and learn from each other. 👇😄
Don't forget to share this blog post with your friends and colleagues who might find it helpful. Together, we can make shell command execution more manageable! 💪🌟
Happy coding! ✨🔥
References:
Python Documentation -
subprocess
Note: The code examples in this blog post are written in Python 3.