Return JSON response from Flask view
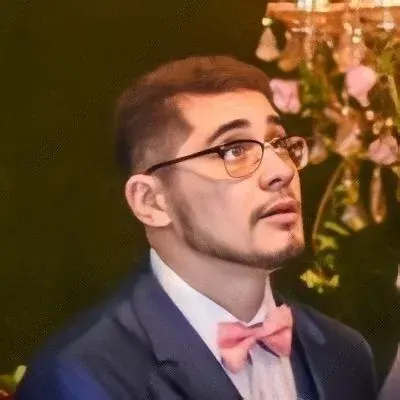
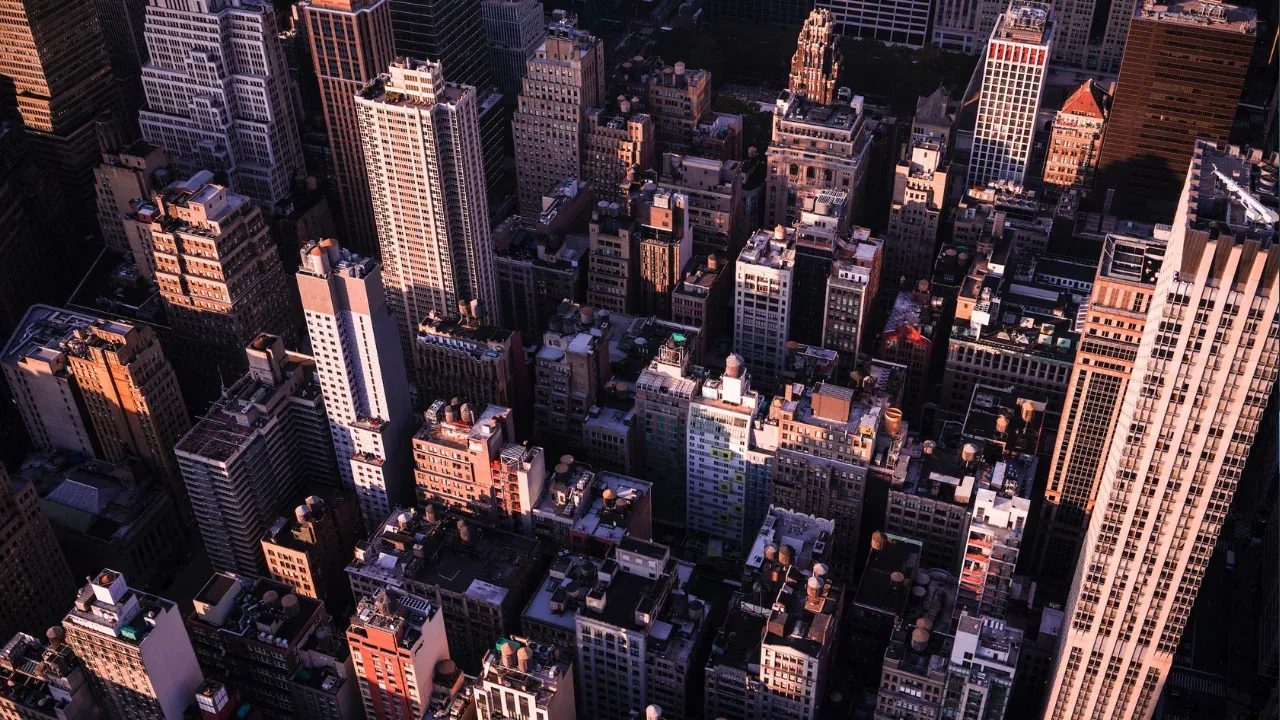
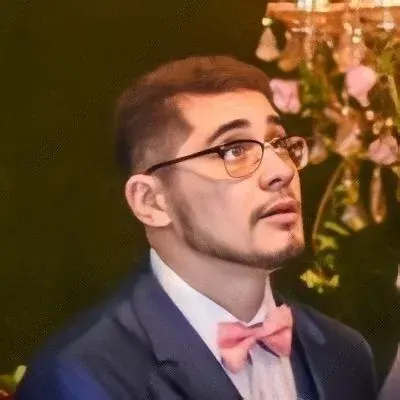
How to Return JSON Response from Flask View 🐍🔥
Are you looking to return a JSON response from a Flask view? We've got you covered! 🙌
In this guide, we will address the common issue of returning JSON responses in Flask and provide you with easy solutions to help you do it in no time. Let's get started! 💪
The Challenge: Returning JSON Response
So, you have a function that analyzes a CSV file with Pandas and produces a dictionary with summary information. Now, you want to return these results as a response from a Flask view. But how can you return a JSON response? 🤔
Here's an example of the code you might be working with:
@app.route("/summary")
def summary():
d = make_summary()
# send it back as json
Solution: Using Flask's jsonify Function 👍
To send a JSON response from your Flask view, you can use Flask's jsonify
function. This function allows you to easily convert your Python dictionary into a JSON response. 🚀
Here's how you can modify your code to return a JSON response:
from flask import jsonify
@app.route("/summary")
def summary():
d = make_summary()
return jsonify(d)
That's it! Now, when you visit the /summary
URL in your Flask application, you will receive a JSON response containing the summary information from your CSV file. Cool, right? 😎
Additional Tips and Tricks 💡
Handling Status Codes 🚦
You can also include status codes in your JSON responses to provide additional information. For example, if your summary function encounters an error, you might want to include a 500
status code to indicate a server error.
from flask import jsonify
@app.route("/summary")
def summary():
try:
d = make_summary()
return jsonify(d), 200
except:
error_response = {"error": "An error occurred during the summary generation."}
return jsonify(error_response), 500
Controlling JSON Formatting 📋
By default, Flask's jsonify
function sorts the keys of your dictionary in alphabetical order and formats the JSON response with indentation. However, if you prefer to control the JSON formatting, you can use the json.dumps
method from the built-in json
module.
import json
@app.route("/summary")
def summary():
d = make_summary()
return json.dumps(d, indent=4), 200
Feel free to explore the options provided by the json.dumps
method to customize the formatting according to your needs.
Conclusion and Next Steps 🎉
Congratulations! You now know how to return a JSON response from a Flask view. We hope this guide helped you overcome any challenges you were facing. 🙌
If you found this guide helpful, make sure to share it with your friends and colleagues who might also benefit from it. And don't forget to subscribe to our newsletter for more useful guides and tutorials.
Now it's time to put your knowledge into practice and create amazing Flask applications that return JSON responses. Happy coding! 💻🚀