Replacing Pandas or Numpy Nan with a None to use with MysqlDB
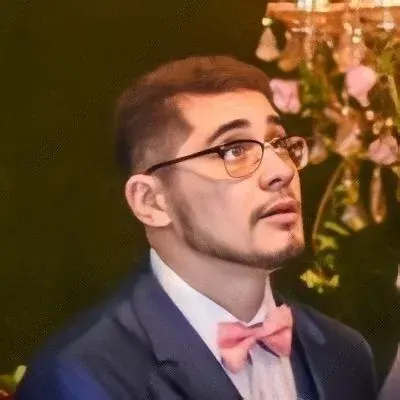
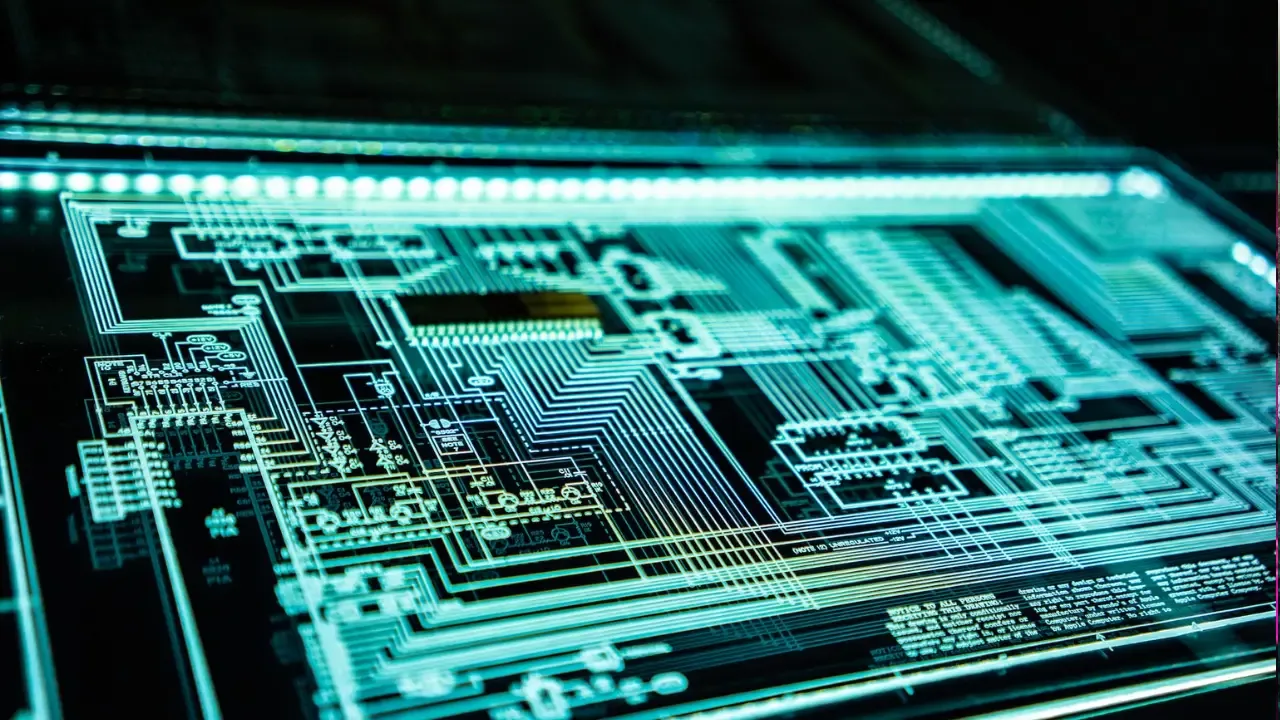
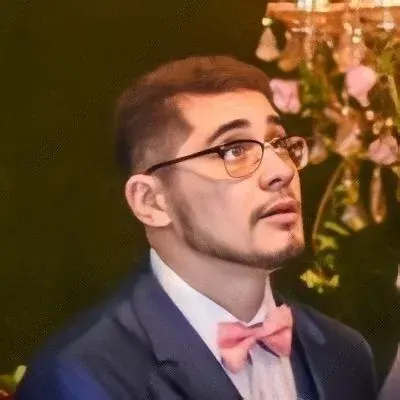
š Title: Replacing Pandas or Numpy Nan with None for MysqlDB: The Ultimate Guide š¼
š Welcome to TechExplained! Today, we'll dive deep into a common issue faced by data scientists and developers who are trying to store Pandas dataframes or Numpy arrays into a MySQL database using MysqlDB. If you've ever encountered an error stating "nan is not in the field list," you've come to the right place! š
Understanding the Problem š¤
š The issue arises from the fact that MysqlDB doesn't recognize the 'nan' value, which represents missing or undefined data in Pandas and Numpy. As a result, attempting to store 'nan' directly into a MySQL database leads to errors.
š Let's break it down with an example:
import pandas as pd
import MySQLdb as mdb
df = pd.DataFrame({'col1': [1, 2, 'NaN'], 'col2': [3, 'NaN', 5]})
con = mdb.connect(host='localhost', user='your_username', passwd='your_password', db='your_database')
df.to_sql('table_name', con)
ā ļø Running the code above would trigger an error: "ProgrammingError: (1064, "You have an error in your SQL syntax;... nan is not in the field list").
Easy Solutions to the Rescue! š
š§ We have a couple of simple approaches to fix this problem, both involving converting 'nan' to the NoneType, which MySQL can handle perfectly fine. Let's explore them together!
Solution 1: Replacing 'nan' with None in the Dataframe š
š Before writing the dataframe to the database, we need to replace all instances of 'nan' with None using the .where()
function from Pandas. Here's how:
df = df.where(pd.notnull(df), None)
š” By applying .where(pd.notnull(df), None)
, we replace all 'nan' occurrences with None, ensuring that MySQL understands and handles them correctly.
Solution 2: Using the numpy.nan_to_num() Function āļø
š¦ If you're working with Numpy arrays, fret not! There's an alternative solution that utilizes the numpy.nan_to_num()
function. Here's how you can implement it:
import numpy as np
np_array = np.array([1, 2, np.nan, 4, 5])
np_array = np.nan_to_num(np_array, nan=None)
š Calling numpy.nan_to_num()
with the parameter nan=None
replaces all 'nan' values with None. After this transformation, you can safely store the array in your MySQL database using MysqlDB.
Let's Put It All Together! š
š» Now that we've learned the two awesome solutions, let's modify our previous code snippet to ensure it works flawlessly:
import pandas as pd
import MySQLdb as mdb
df = pd.DataFrame({'col1': [1, 2, 'NaN'], 'col2': [3, 'NaN', 5]})
df = df.where(pd.notnull(df), None)
con = mdb.connect(host='localhost', user='your_username', passwd='your_password', db='your_database')
df.to_sql('table_name', con)
š Voila! Your dataframe will now be successfully written into your MySQL database without triggering any errors related to 'nan' values. š
š” Pro Tip: Remember to replace 'your_username'
, 'your_password'
, and 'your_database'
with your actual MySQL details.
Engage and Share! š£
š Applause to you for reaching the end! We hope this guide has resolved your issue and empowered you to smoothly store data from Pandas or Numpy into MySQL using MysqlDB. š
šÆ Feel free to share this guide with fellow developers and data enthusiasts who might find it useful! And if you have any other tech-related questions, drop a comment below or reach out on social media. Happy coding! šš©āš»šØāš»
<p align="center">āØāØāØ</p>