Replacing blank values (white space) with NaN in pandas
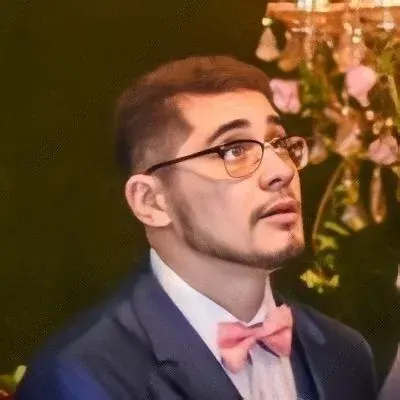
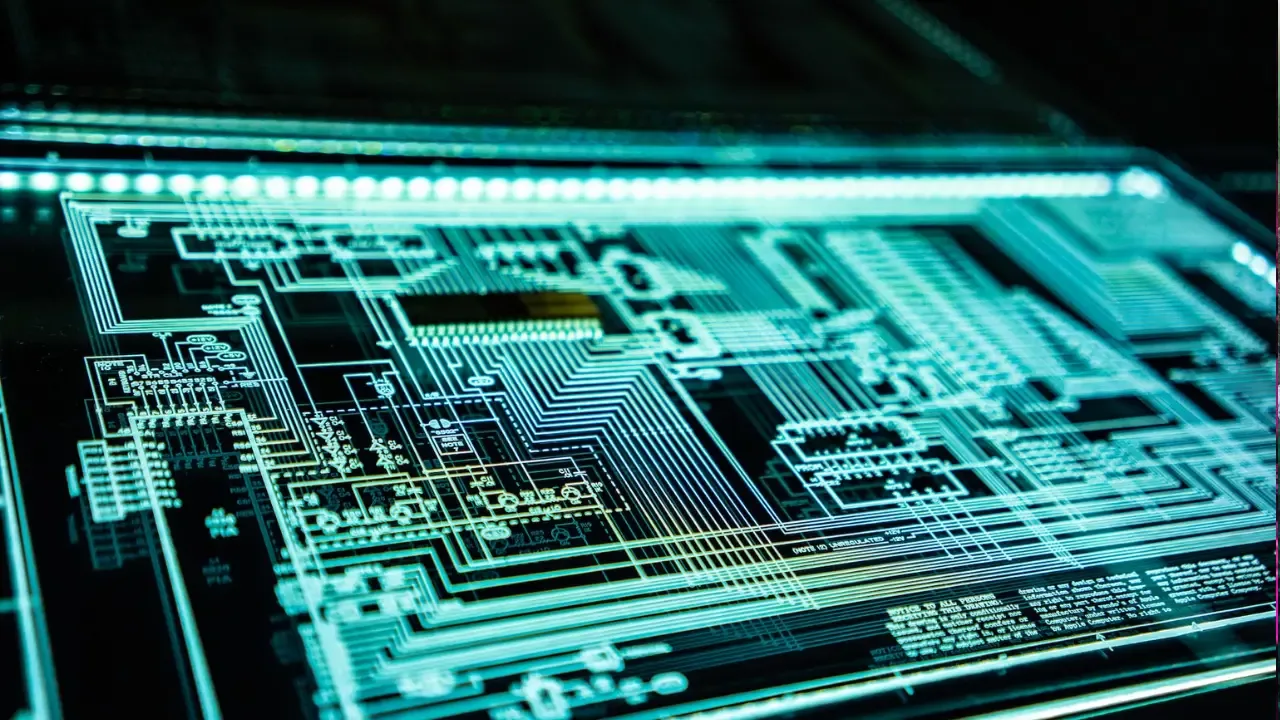
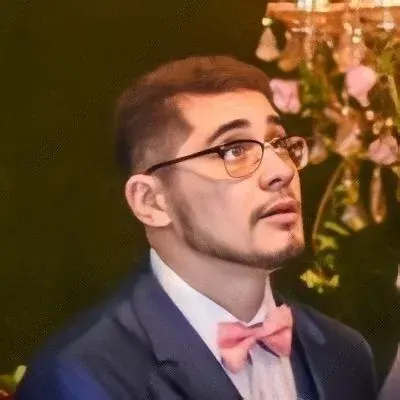
Replacing Blank Values (Whitespace) with NaN in Pandas: A Complete Guide 🐼
Are you facing the challenge of dealing with blank values (whitespace) in your Pandas dataframe? Look no further! In this guide, we will address this common issue and provide you with easy solutions to replace those blank values with NaNs. So let's dive straight into it!
The Problem: Blank Values in Pandas DataFrame
Consider the following Pandas dataframe:
A B C
2000-01-01 -0.532681 foo 0
2000-01-02 1.490752 bar 1
2000-01-03 -1.387326 foo 2
2000-01-04 0.814772 baz
2000-01-05 -0.222552 4
2000-01-06 -1.176781 qux
Here, we have some blank values represented by whitespace in columns B and C. Our goal is to replace those blank values with NaNs. But how can we achieve this in a Pythonic, efficient, and elegant way? Let's explore the solutions!
Solution 1: Looping through Columns
One way to tackle this problem is by looping through each column and applying a boolean replacement based on a column mask generated by a function that performs a regex search for whitespace. However, the code for this solution might look a bit clunky and less efficient:
import re
for column in df.columns:
df[column][df[column].apply(lambda x: True if re.search('^\s*$', str(x)) else False)] = None
Note that this code replaces the target strings with None
, which can be easily handled by Pandas' fillna()
function.
Solution 2: Optimized Iteration
To make the code more efficient, we can optimize it by iterating only through fields that could potentially contain empty strings. We can achieve this by checking the column's data type and filtering accordingly. Here's a modified version of the code using this optimization:
import numpy as np
for column in df.columns:
if df[column].dtype == np.dtype('object'):
df[column][df[column].apply(lambda x: True if re.search('^\s*$', str(x)) else False)] = None
While this optimization improves the performance, it may not be a significant improvement for smaller dataframes. However, it can make a noticeable difference for larger datasets.
Solution 3: Inserting NaN Directly
In the previous solutions, we replaced the blank values with None
. However, if you prefer to have NaN
in your dataframe, we can modify the code to insert NaN
directly. Here's how you can achieve that:
for column in df.columns:
if df[column].dtype == np.dtype('object'):
df[column][df[column].apply(lambda x: True if re.search('^\s*$', str(x)) else False)] = np.nan
This modification allows you to work seamlessly with Pandas' functions like fillna()
without any further conversions.
Conclusion
Dealing with blank values (whitespace) in a Pandas dataframe doesn't have to be a complex or tedious task. By following the solutions presented in this guide, you can easily replace those blank values with NaNs, making your data analysis smoother and more accurate.
Remember to choose the solution that suits your preferences and optimize the code accordingly. Now you're empowered with the knowledge to handle this common issue effortlessly!
If you found this guide helpful and want to learn more about working with pandas or any other tech-related topics, make sure to subscribe to our newsletter for regular updates. Don't forget to share this post with fellow data enthusiasts to spread the knowledge.
Happy coding! 👩💻👨💻