Replacements for switch statement in Python?
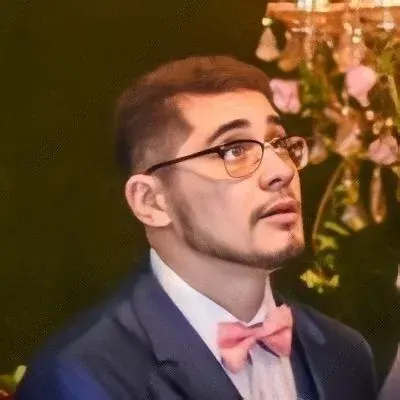
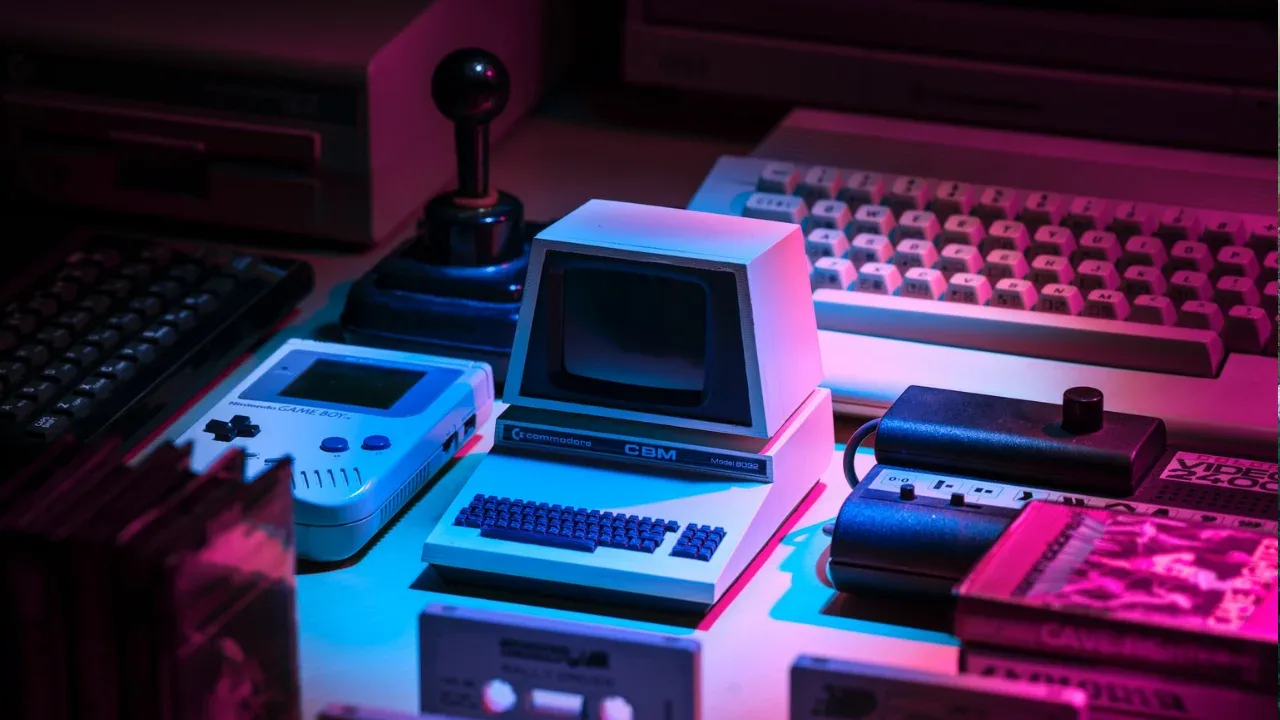
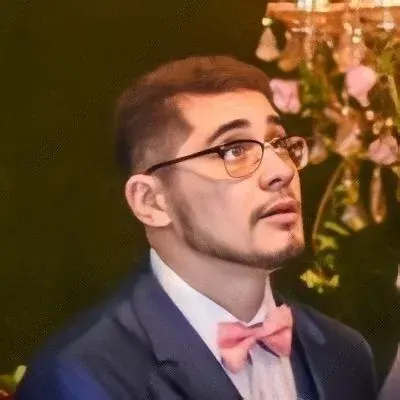
Replacements for switch statement in Python? π‘
So you're writing some Python code and you need to implement branching logic based on the value of an input index. In other languages, you might have used a switch
or case
statement, but Python doesn't have that built-in. Don't worry, though! There are some recommended alternatives that you can use to achieve the same result. Let's dive in! πββοΈ
The problem at hand π€
You want to create a function that returns different fixed values based on the value of an input index. This is a common programming scenario, often encountered when you need to handle different cases for a given input.
Solution 1: If-elif-else chain π§
The first alternative to a switch
statement in Python is to use an if-elif-else
chain. Here's how it works:
def get_fixed_value(index):
if index == 1:
return "First value"
elif index == 2:
return "Second value"
elif index == 3:
return "Third value"
else:
return "Default value"
You can add as many elif
statements as you need to handle different cases. The last else
statement serves as the default case.
Solution 2: Dictionary-based dispatch πΊοΈ
Another approach is to use a dictionary to map the index values to the corresponding fixed values. Here's an example:
def get_fixed_value(index):
values = {
1: "First value",
2: "Second value",
3: "Third value"
}
return values.get(index, "Default value")
In this solution, we create a dictionary values
where the keys are the index values and the values are the fixed values associated with each index. The get()
method allows us to retrieve the value for a given key, and if the key is not found, it returns the default value specified as the second argument.
The choice is yours! π€
Now that you have two possible solutions to replace the switch
statement in Python, you can choose the one that suits your needs the best.
Remember to consider the complexity of your code, the readability, and the maintainability of your solution. Sometimes, the if-elif-else
chain may be more appropriate, while in other cases, the dictionary-based dispatch might be a better fit.
Feel free to experiment with both approaches and see which one resonates with you!
If you have any other interesting solutions or tips regarding this problem, make sure to share them in the comments section below. Let's collaborate and make the Python community even stronger! πͺ
Happy coding! πβ¨