Renaming column names in Pandas
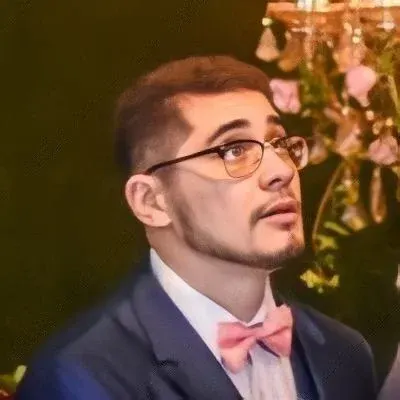
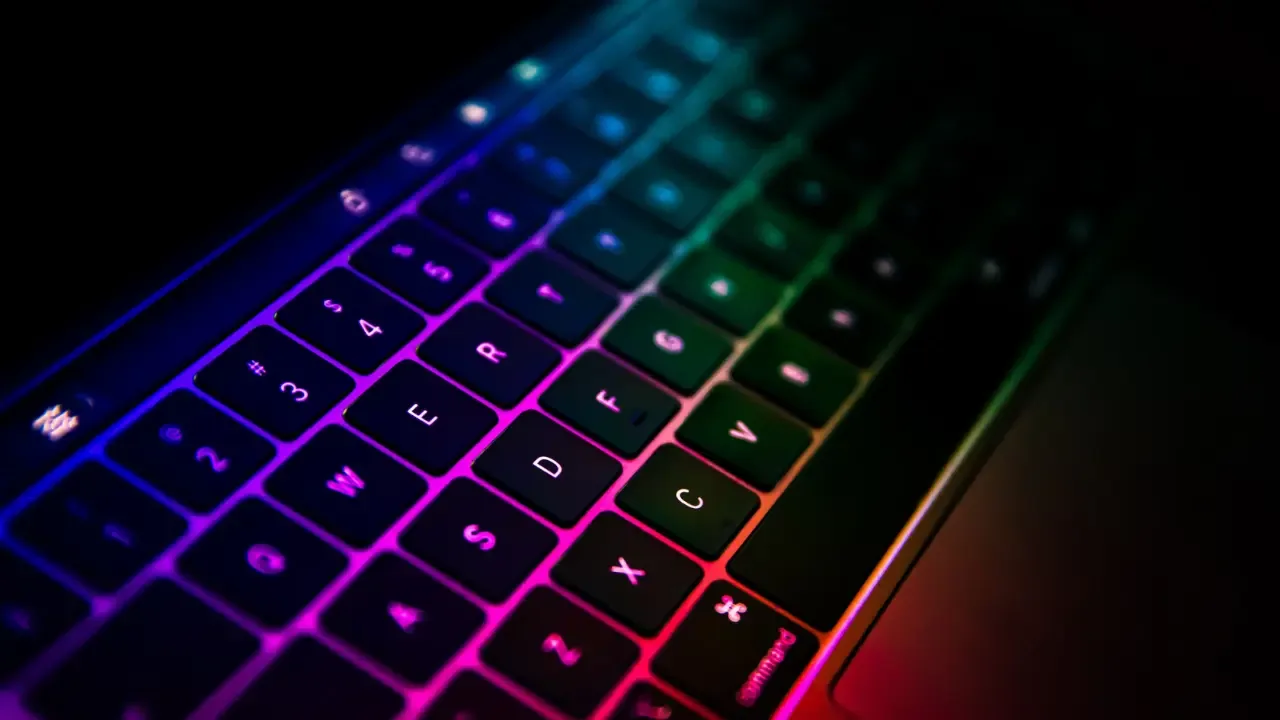
How to Rename Column Names in Pandas πΌ
So you have a Pandas DataFrame and you want to rename the column labels, huh? No worries, it's easier than you might think! In this guide, I'll walk you through a common issue faced by data analysts and provide you with some simple solutions. Let's dive in! πͺ
The Problem π
Say you have a DataFrame with column labels like ['$a', '$b', '$c', '$d', '$e']
, but you want to rename them to ['a', 'b', 'c', 'd', 'e']
. How do you go about doing that in Pandas? Let me show you!
Solution 1: Using the .rename()
Method π
One way to rename the column labels is by using the .rename()
method provided by Pandas. Here's how you can do it:
import pandas as pd
# Assuming your DataFrame is named df
new_column_names = ['a', 'b', 'c', 'd', 'e']
df.rename(columns=dict(zip(df.columns, new_column_names)), inplace=True)
In the code snippet above, we import the pandas
library and specify the new column labels in new_column_names
. We then use the .rename()
method to rename the columns using a dictionary comprehension (dict(zip(df.columns, new_column_names))
). Finally, we set inplace=True
to modify the DataFrame in place.
Solution 2: Assigning a New List to .columns
π
Another way to rename column labels is by directly assigning a new list to the .columns
property of the DataFrame. Here's how you can do it:
import pandas as pd
# Assuming your DataFrame is named df
new_column_names = ['a', 'b', 'c', 'd', 'e']
df.columns = new_column_names
In this solution, we assign the new list of column labels, new_column_names
, to df.columns
. This will replace the existing column labels with the new ones.
Time to Take Action! π
Now that you've learned two different approaches to rename column names in Pandas, it's time to put that knowledge into action! Choose the method that resonates with you and tackle your column renaming task like a pro! π₯
Got any other Pandas-related questions or need further assistance? Let me know in the comments below! I'm here to help you excel in your data analysis journey! π
Keep exploring, keep learning, and keep coding! Happy pandas-ing! πΌπ»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
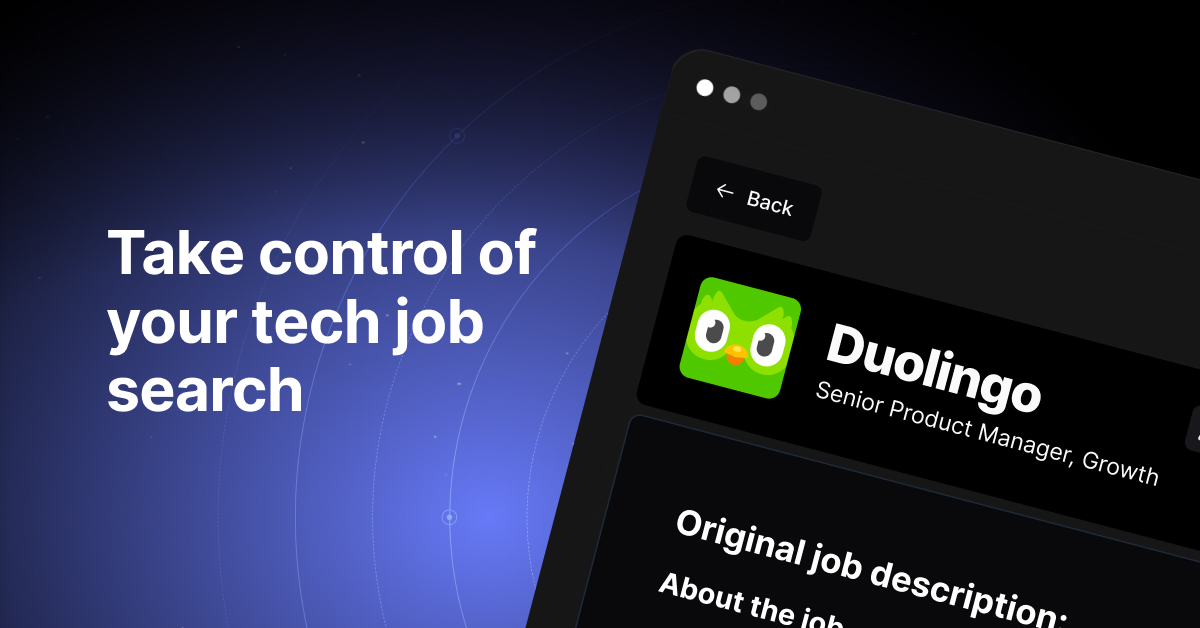