Remove specific characters from a string in Python
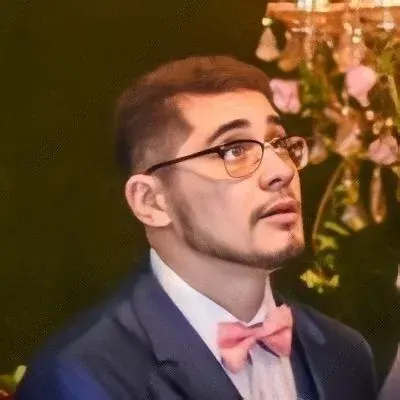
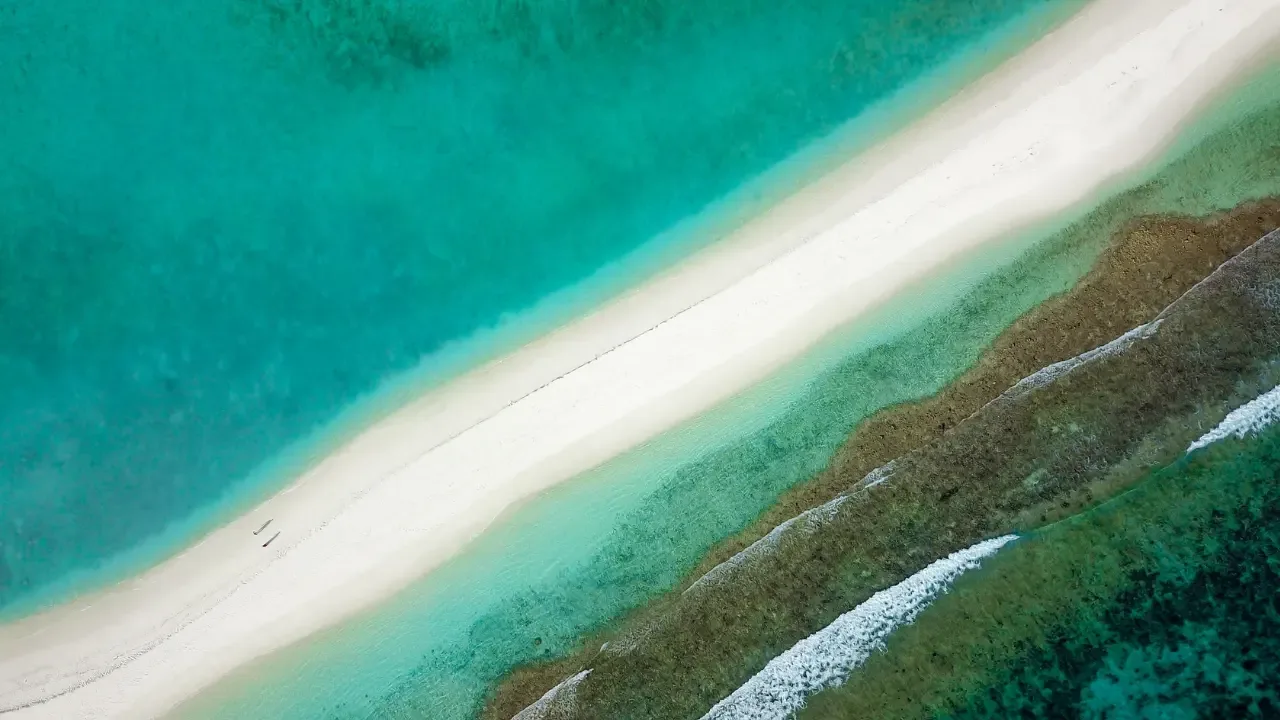
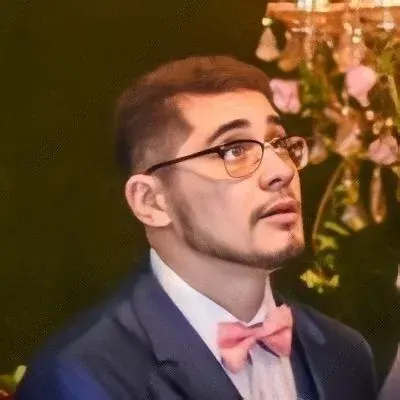
Removing Specific Characters from a String in Python
Are you struggling to remove specific characters from a string in Python? Don't worry, you're not alone! Many developers encounter this problem while working with strings. In this guide, we'll walk you through common issues and provide easy solutions to get rid of those unwanted characters.
The Problem
Let's start by addressing the specific problem mentioned in the context above. The code snippet provided attempts to remove certain characters from a string using the replace()
method. However, it fails to modify the string as expected. So, what's going wrong?
The issue lies in the misunderstanding of how strings work in Python. 🤔 Strings in Python are immutable, which means that you cannot modify them directly. Instead, you need to assign the result of the replace()
method to a new variable or reassign it to the same variable to update the string.
The Solution
To solve this problem, let's modify the code snippet to correctly remove specific characters from the string:
line = line.replace(char, '')
Now, the replace()
method returns a new string with the specified characters removed, and we assign this new string back to the line
variable.
But wait, there's another problem lurking! 😱 The replace()
method is called within a loop, which means it will only check one character at a time. This could lead to unexpected results because the modified string may not contain all the desired replacements.
To avoid this issue, you can iterate over the characters you want to remove and apply the replacements all at once. Here's the modified code:
for char in " ?.!/;:&":
line = line.replace(char, '')
In this code, we iterate over each character in the string of characters we want to remove. For each iteration, we call replace()
to eliminate all occurrences of that character from the line
string.
Further Enhancements
There are several ways to further enhance this solution based on your specific needs. Here are a couple of ideas:
Using Regular Expressions
If you need to remove a more complex pattern of characters from a string, you can leverage the power of regular expressions. The re
module in Python provides powerful tools for pattern matching and substitution. Consider the following example:
import re
line = re.sub(r"[ ?.!/;:&]", "", line)
By using the re.sub()
function, you can search for a regular expression pattern and replace all occurrences with an empty string. This approach provides greater flexibility when dealing with more intricate character removals.
Removing Leading and Trailing Characters
If you only want to remove specific characters at the beginning or end of a string, you can use the strip()
method. This method removes leading or trailing characters that match the provided argument. Check out the following example:
line = line.strip(" ?.!/;:&")
By passing the characters you want to remove as an argument to strip()
, you can easily get rid of them while preserving the rest of the string.
Share Your Ideas
Now that you have learned how to remove specific characters from a string in Python, it's time to put your knowledge into practice! 🚀
Have you encountered any other interesting solutions to this problem? Do you have any questions or suggestions? Share them in the comments below! Let's learn and grow together as a community of Python developers.
Remember, understanding how strings work in Python and leveraging the appropriate methods and techniques will help you successfully remove specific characters from your strings. Happy coding! 😄