Remove pandas rows with duplicate indices
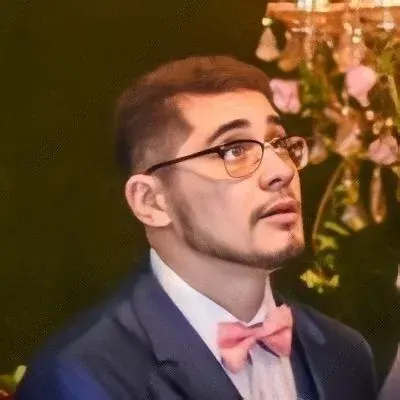
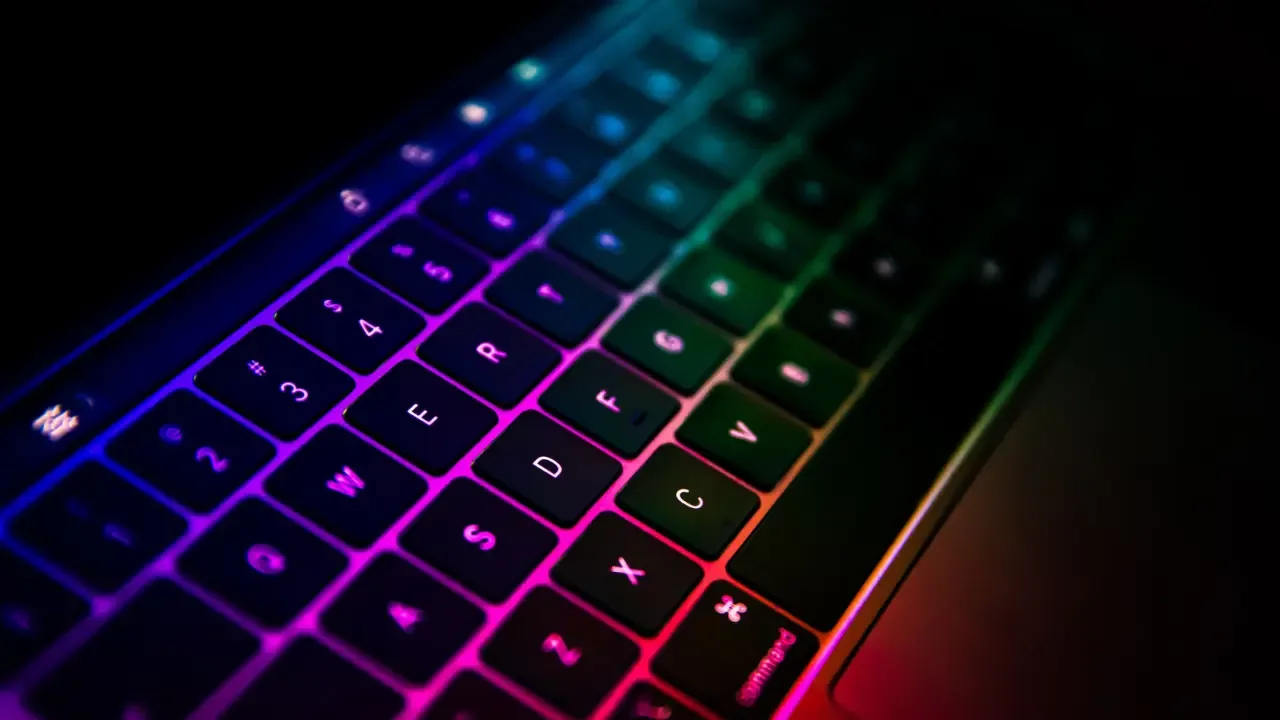
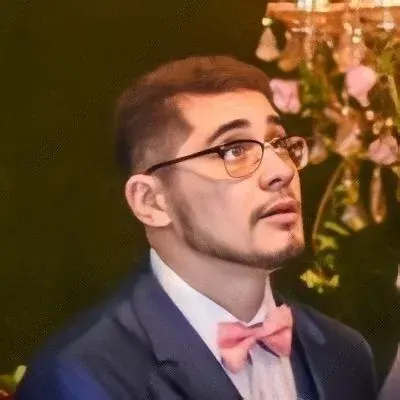
Removing Rows with Duplicate Indices in pandas
👋 Hey there tech enthusiasts! Have you ever found yourself dealing with a pandas DataFrame that has duplicate rows with the same index values? 🤔 It can be quite a puzzling situation, especially if you're not sure how to handle it. But don't worry, because in this blog post, I'm here to guide you through the process of removing those pesky duplicates and getting your DataFrame back in shape! 💪
The Problem: Duplicate Rows with the Same Index
Let's set the stage for our problem. Imagine you're working with a DataFrame that contains weather observations recorded every 5 minutes. However, some entries have duplicate rows appended to the end of the file when errors are corrected. Here's an example of what your DataFrame might look like:
Sta Precip1hr Precip5min Temp DewPnt WindSpd WindDir AtmPress
Date
2001-01-01 00:00:00 KPDX 0 0 4 3 0 0 30.31
2001-01-01 00:05:00 KPDX 0 0 4 3 0 0 30.30
2001-01-01 00:10:00 KPDX 0 0 4 3 4 80 30.30
2001-01-01 00:15:00 KPDX 0 0 3 2 5 90 30.30
2001-01-01 00:20:00 KPDX 0 0 3 2 10 110 30.28
Looking at this example, we can see that the row with the index 2001-01-01 00:00:00
is duplicated at the end of the DataFrame.
The Solution: Removing Duplicate Rows
To remove rows with duplicate indices in pandas, we can follow these simple steps:
Identify the duplicate rows: We need to find the rows in our DataFrame that have duplicate indices 🧐. In pandas, we can do this using the
duplicated
method.Remove the duplicate rows: Once we have identified the duplicate rows, we can use the
.drop_duplicates()
method to remove them from our DataFrame.
To apply these steps to our example, let's assume we have a DataFrame named df3
with duplicate rows:
import pandas as pd
import datetime
startdate = datetime.datetime(2001, 1, 1, 0, 0)
enddate = datetime.datetime(2001, 1, 1, 5, 0)
index = pd.date_range(start=startdate, end=enddate, freq='H')
data1 = {'A' : range(6), 'B' : range(6)}
data2 = {'A' : [20, -30, 40], 'B' : [-50, 60, -70]}
df1 = pd.DataFrame(data=data1, index=index)
df2 = pd.DataFrame(data=data2, index=index[:3])
df3 = df2.append(df1)
df3
Running this code will give us the following DataFrame:
A B
2001-01-01 00:00:00 20 -50
2001-01-01 01:00:00 -30 60
2001-01-01 02:00:00 40 -70
2001-01-01 03:00:00 3 3
2001-01-01 04:00:00 4 4
2001-01-01 05:00:00 5 5
2001-01-01 00:00:00 0 0
2001-01-01 01:00:00 1 1
2001-01-01 02:00:00 2 2
Now, let's proceed with removing the duplicate rows using the steps outlined earlier:
df3 = df3.loc[~df3.index.duplicated(keep='first')]
After running this snippet, our DataFrame df3
will no longer contain any duplicate rows with the same indices.
A B
2001-01-01 00:00:00 0 0
2001-01-01 01:00:00 1 1
2001-01-01 02:00:00 2 2
2001-01-01 03:00:00 3 3
2001-01-01 04:00:00 4 4
2001-01-01 05:00:00 5 5
And voilà! We have successfully removed the duplicate rows from our DataFrame, leaving us with a clean and tidy dataset. 🎉
Conclusion and Call-to-Action
Dealing with duplicate rows in pandas can be tricky, especially when it comes to handling duplicate indices. However, by following the steps outlined in this post, you can easily remove those duplicates and get back to analyzing your data.
So why not give it a try? Take a look at your own DataFrame and see if you have any duplicate index values. If you do, now you have the knowledge and tools to deal with them effectively!
👍 If you found this blog post helpful, don't hesitate to share it with your tech-savvy friends or colleagues who might be struggling with the same issue. And remember, stay curious and keep exploring the wonderful world of data analysis! 😄