Remove empty strings from a list of strings
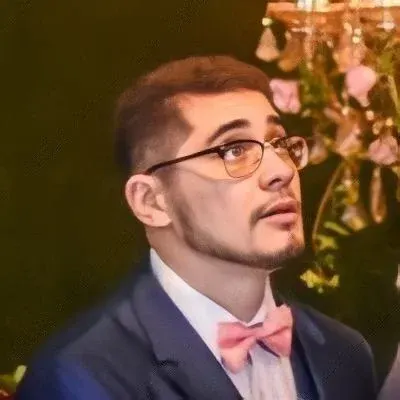

๐งน How to Remove Empty Strings from a List of Strings in Python ๐
Have you ever found yourself with a messy list full of empty strings that you just want to get rid of? Well, fear not! Today we're going to explore how to remove these pesky empty strings from a list of strings in Python. ๐งป
The Problem ๐ซ
Our fellow Pythonista is facing the following problem: they want to remove all empty strings from a list of strings. ๐ค
Here's what their initial idea looks like:
while '' in str_list:
str_list.remove('')
While this approach technically works, it might not be the most elegant or "Pythonic" solution. Thankfully, there are more efficient ways to tackle this issue. ๐ก
The Pythonic Solution ๐
Python provides a variety of tools to make your code more concise and readable. One way to remove empty strings from a list is to use a list comprehension. Here's how it works:
str_list = [s for s in str_list if s]
Let's break it down:
We iterate over each string
s
instr_list
.We keep the string
s
if it evaluates toTrue
(i.e., it's not empty).The resulting strings are collected and stored back into
str_list
.
Voilร ! ๐ Using a list comprehension, we've successfully removed the empty strings from our list in a more elegant and Pythonic manner.
The Final Call-to-Action ๐ฃ
Now that you know how to remove empty strings from a list of strings in Python, it's time to put your newfound knowledge into practice! โจ
Go ahead and try out the Pythonic solution we discussed above. Modify your code and see how it performs. Share your experience in the comments below! ๐ฌ
Remember, sharing is caring! If you found this tip helpful, don't hesitate to hit the "Share" button and spread the Pythonic wisdom with your fellow developers. ๐
Happy coding! ๐โ๏ธ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
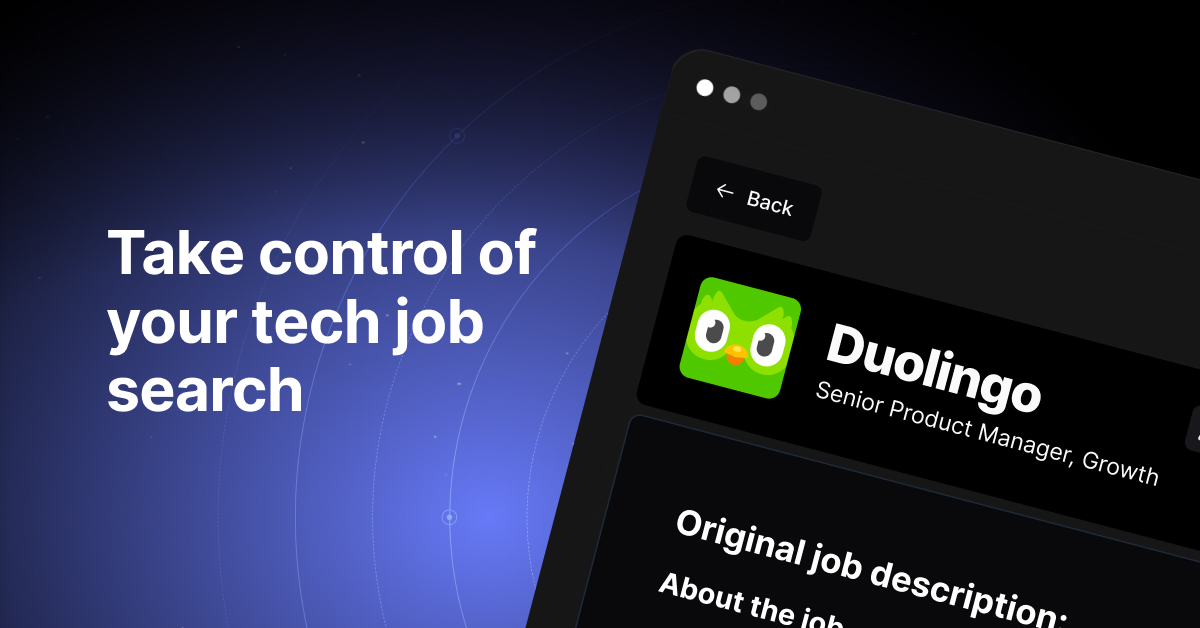