Remove duplicates by columns A, keeping the row with the highest value in column B
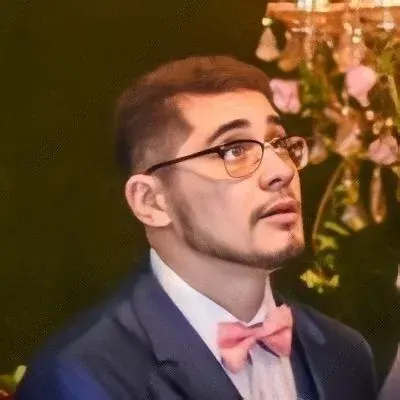
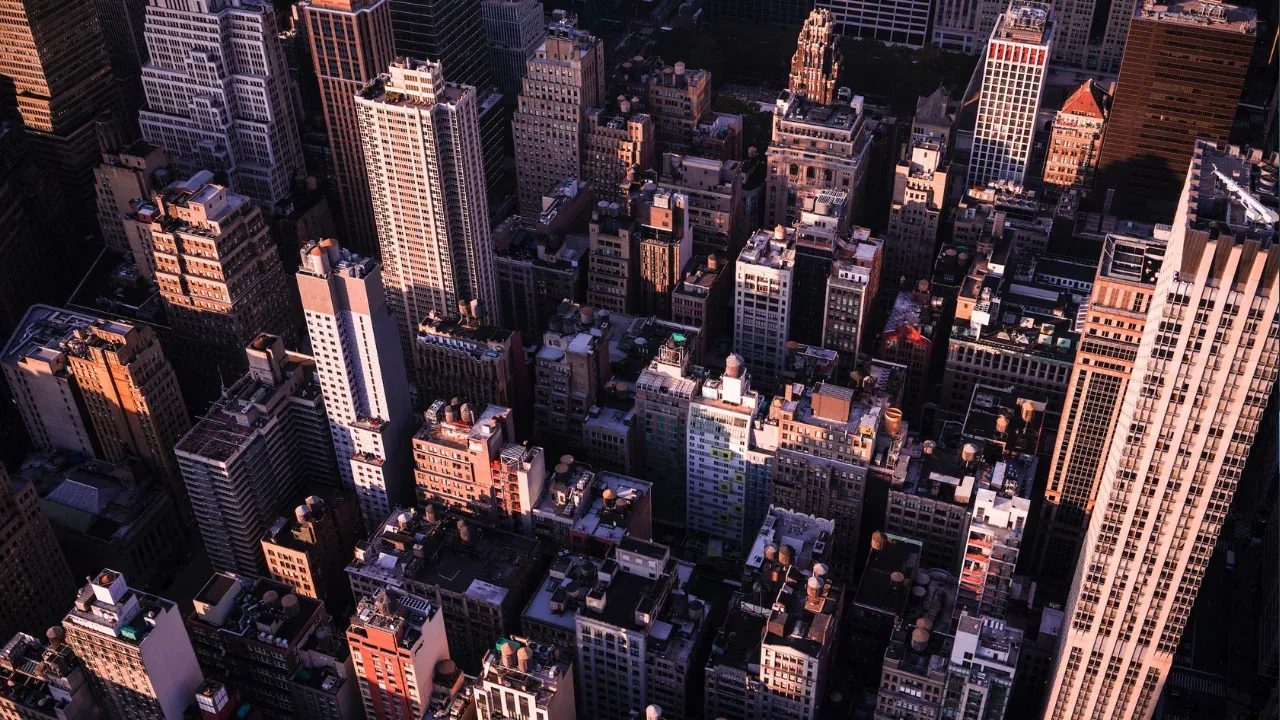
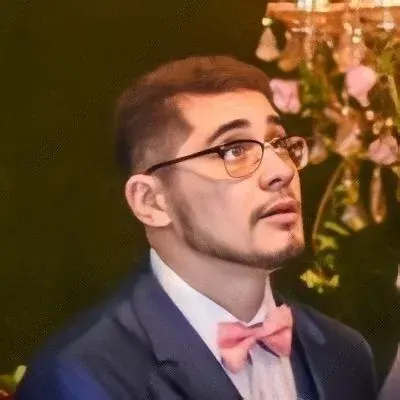
🚀 Easy Guide to Removing Duplicates by Columns A, Keeping the Row with the Highest Value in Column B
So you've found yourself with a dataframe that has duplicate values in column A, but you only want to keep the row with the highest value in column B. Don't worry, I've got you covered! In this guide, I'll walk you through the steps to solve this problem easily and efficiently.
The Problem
Let's start by understanding the problem with an example. Imagine you have the following dataframe:
A B
1 10
1 20
2 30
2 40
3 10
You want to remove the duplicates in column A, while keeping the row with the highest value in column B. So the expected output should be:
A B
1 20
2 40
3 10
The Solution
Step 1: Sorting the DataFrame
To solve this problem, we need to sort the dataframe based on column B in descending order. This will ensure that the row with the highest value in column B appears first for each unique value in column A.
Here's how you can sort the dataframe using pandas:
sorted_df = df.sort_values(by='B', ascending=False)
Step 2: Dropping Duplicates
Now that the dataframe is sorted, we can drop the duplicates in column A while keeping the first occurrence (which will be the row with the highest value in column B).
final_df = sorted_df.drop_duplicates(subset='A')
And there you have it! You now have a new dataframe, final_df
, that only contains the rows where column A is unique, keeping the row with the highest value in column B intact.
💡 Pro Tip
If you want to modify the original dataframe instead of creating a new one, you can use the inplace=True
parameter in the drop_duplicates()
method. This will update the dataframe without creating a copy.
df.sort_values(by='B', ascending=False, inplace=True)
df.drop_duplicates(subset='A', inplace=True)
Conclusion
Removing duplicates by columns A, while keeping the row with the highest value in column B, can be done easily with just a few simple steps. By sorting the dataframe and then dropping duplicates, you'll have a clean and tidy dataframe.
Have any other data wrangling challenges or curious about other pandas tricks? Let me know in the comments below! Happy coding! 🔥
🎉 Get in Touch
I hope this guide was helpful to you! If you have any more questions or need further assistance, feel free to reach out to me on Twitter or leave a comment below. Don't forget to share this guide with your friends and colleagues who might find it useful.
Until next time, keep programming! Happy data exploration! 🚀