Remove all whitespace in a string
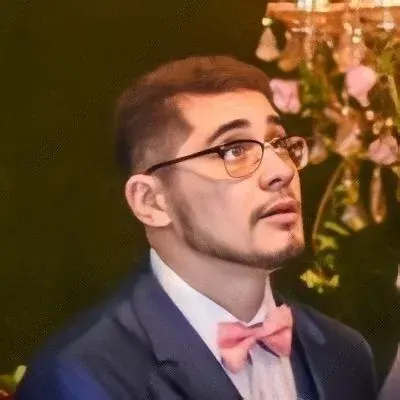
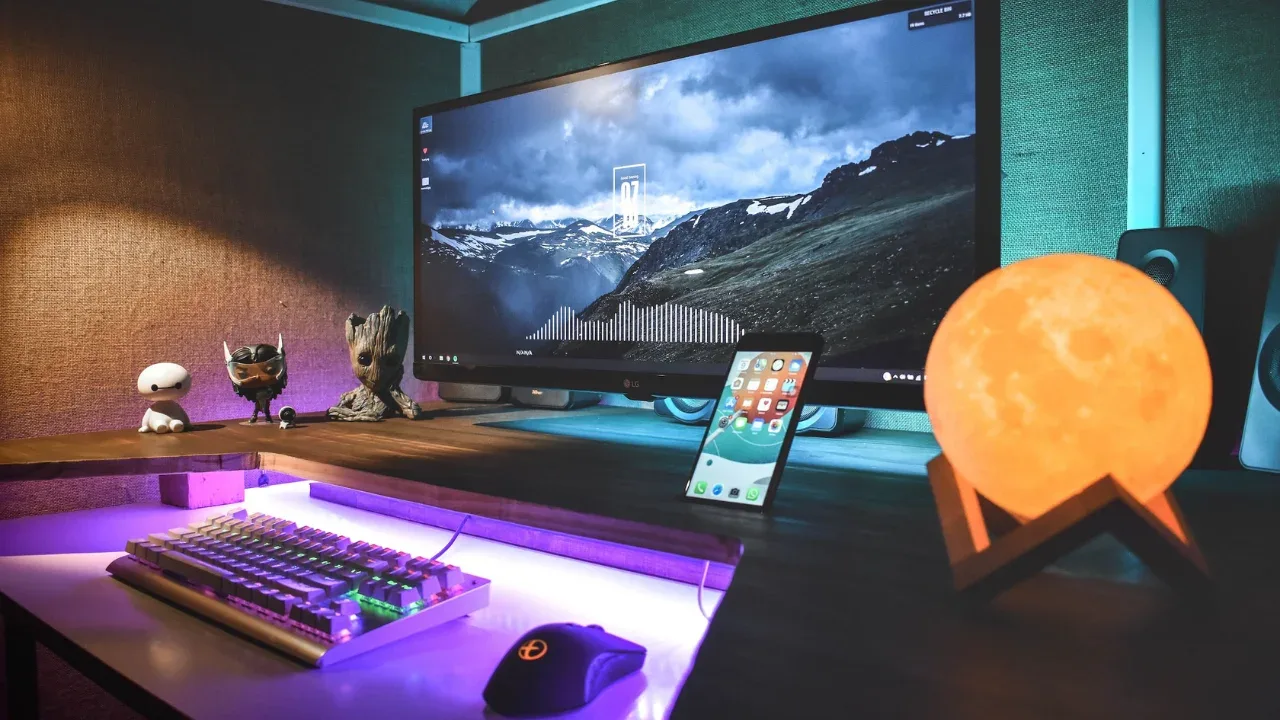
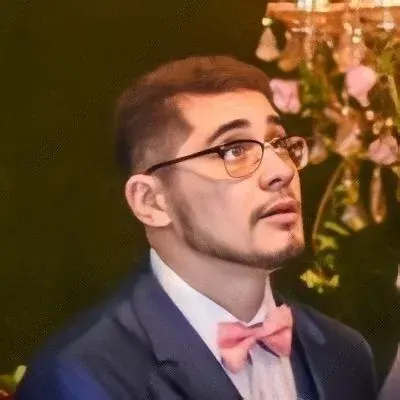
Removing All Whitespace in a String: A Complete Guide ๐งน๐
Are pesky white spaces ruining your string manipulation game? Don't worry, we've got you covered! In this blog post, we'll walk you through the common issues and provide easy-to-implement solutions to remove all whitespace in a string using Python. Let's dive in! ๐ช
The Problem: Struggling to Remove All Whitespace ๐ซ
So, you have a string that's infested with unnecessary whitespace, and you want to get rid of it all. The Python code you tried using strip()
function seemingly didn't do the trick, and now you're wondering if there's a way to eliminate whitespace between words too. We hear you, and we've got the answers!
Solution 1: Replace Whitespace with an Empty String using replace()
๐
To remove all whitespace from a string, you can use the replace()
function in Python. Simply replace all occurrences of whitespace with an empty string. Take a look at the modified code snippet below:
def my_handle(self):
sentence = ' hello apple '
sentence = sentence.replace(" ", "")
By replacing all the spaces with an empty string, you effectively remove all whitespace in the string. Easy as pie! ๐ฐ
Solution 2: Use Regular Expressions with re
Module ๐งต๐
Another powerful method to eliminate whitespace in a string is by utilizing the re
module in Python, which provides regular expression-based operations. Regular expressions allow you to define complex patterns for matching and manipulating strings.
Here's an example of how you can remove all whitespace using regular expressions:
import re
def my_handle(self):
sentence = ' hello apple '
sentence = re.sub(r'\s', '', sentence)
In this case, the regular expression pattern \s
matches any whitespace character (spaces, tabs, newlines, etc.). By replacing it with an empty string, you successfully eradicate all whitespace in the string.
Conclusion and Call to Action ๐ก๐ข
There you have it! Now you know not one but two effective ways to remove all whitespace from a string in Python. Whether you go for the replace()
function or the power of regular expressions with the re
module, whitespace will never stand in your way again!
Test out these solutions on your own strings and see the magic happen. And if you encounter any issues or have more questions, don't hesitate to drop a comment below. We'd love to assist you further! ๐
Lastly, if you found this blog post helpful, why not share it with your fellow coding enthusiasts? Spread the knowledge and help others conquer whitespace-related woes too. Happy coding! ๐ฉโ๐ป๐จโ๐ป
Please note that the code snippets in this blog post may require additional modifications to fit your specific use case.