Remove all special characters, punctuation and spaces from string
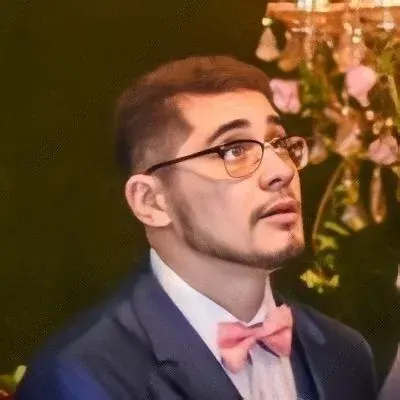
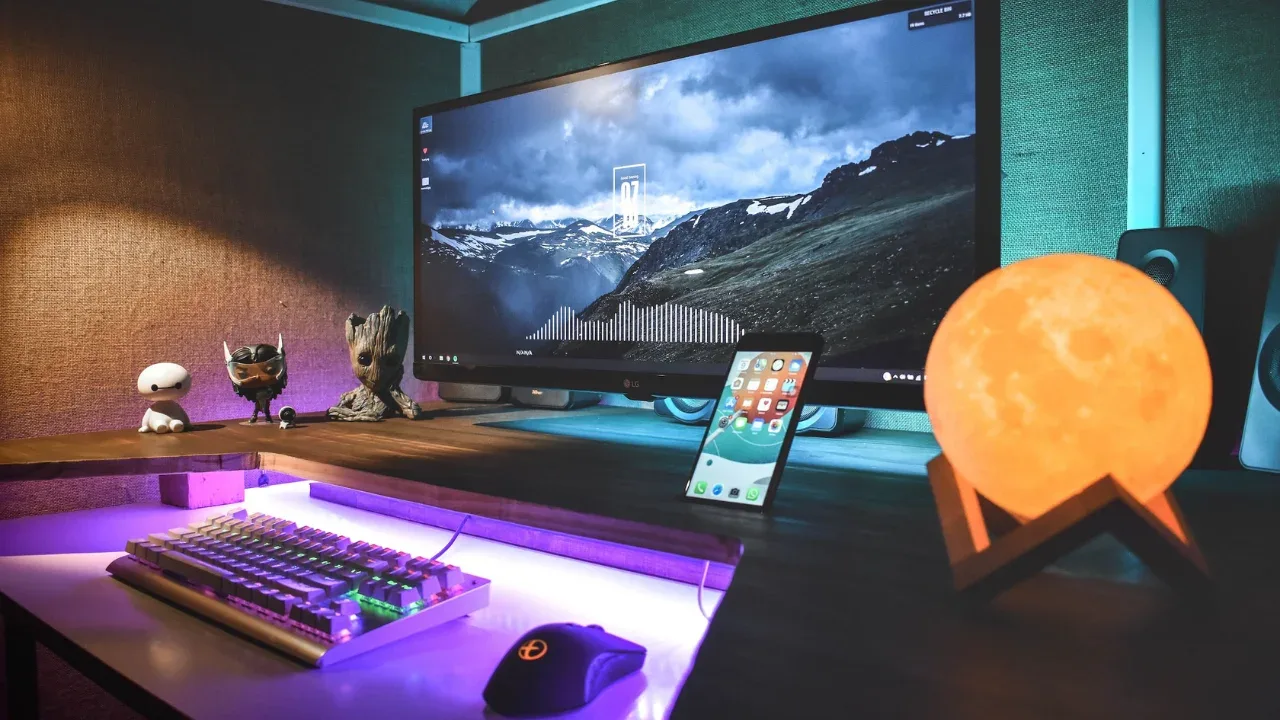
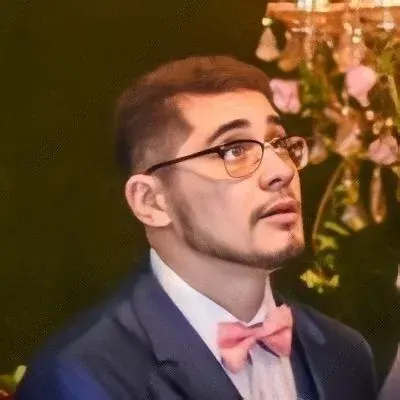
How to Remove Special Characters, Punctuation, and Spaces from a String
Are you tired of dealing with special characters, punctuation, and spaces in your strings? Do you just want the clean, crisp letters and numbers? Well, you're in luck! In this guide, we will show you how to effortlessly remove all those unwanted characters and spaces from your string. Let's dive in! 🚀
The Problem: Special Characters, Punctuation, and Spaces All Over the Place 😫
So, you have a string that's full of messy special characters, punctuation marks, and pesky spaces. You want to get rid of all that clutter and have a string with just the letters and numbers. Trust me, we've all been there. Dealing with this mess can be a real headache, but fear not! We have some simple solutions for you. 💪
Solution 1: Using Regular Expressions (Regex) 💡
Regex is a powerful tool that allows you to perform complex pattern matching and manipulation on strings. In our case, it can help us remove all the unwanted characters and spaces. Here's a simple code snippet in Python to get you started:
import re
def remove_special_chars(string):
cleaned_string = re.sub('[^a-zA-Z0-9]', '', string)
return cleaned_string
string_with_special_chars = "H3ll0! W0rld. Welc0me 😊"
cleaned_string = remove_special_chars(string_with_special_chars)
print(cleaned_string) # Output: "H3ll0W0rldWelc0me"
In the above example, we import the re
module, define a function remove_special_chars
, and use the re.sub
method to replace anything that is not a letter or number with an empty string. Voila! Bye-bye, special characters, punctuation, and spaces! 👋
Solution 2: Using Built-in Functions or Methods 🛠️
If you're not familiar with regular expressions or just prefer a simpler approach, don't worry! Many programming languages have built-in functions or methods that can help you achieve the same result. Here are a few examples:
Python:
def remove_special_chars(string):
cleaned_string = ''.join(e for e in string if e.isalnum())
return cleaned_string
string_with_special_chars = "H3ll0! W0rld. Welc0me 😊"
cleaned_string = remove_special_chars(string_with_special_chars)
print(cleaned_string) # Output: "H3ll0W0rldWelc0me"
JavaScript:
function removeSpecialChars(string) {
var cleanedString = string.replace(/[^a-zA-Z0-9]/g, '');
return cleanedString;
}
var stringWithSpecialChars = "H3ll0! W0rld. Welc0me 😊";
var cleanedString = removeSpecialChars(stringWithSpecialChars);
console.log(cleanedString); // Output: "H3ll0W0rldWelc0me"
In both Python and JavaScript examples, we use the isalnum()
method in Python and the replace()
method in JavaScript to filter out non-alphanumeric characters. It's as simple as that!
The Call-to-Action: Share Your Thoughts! 🙌
Now that you have the power to remove those unwanted characters and spaces from your strings, leave a comment and let us know how these solutions worked for you. Did you find them helpful? Do you have any other tips or tricks to solve this problem? We'd love to hear from you! Don't forget to share this article with your tech-savvy friends who might find it useful. Happy coding! 💻💡
Disclaimer: The examples provided in this article are just a few ways to solve the problem. Make sure to adapt the code to your specific language or use case.