Remap values in pandas column with a dict, preserve NaNs
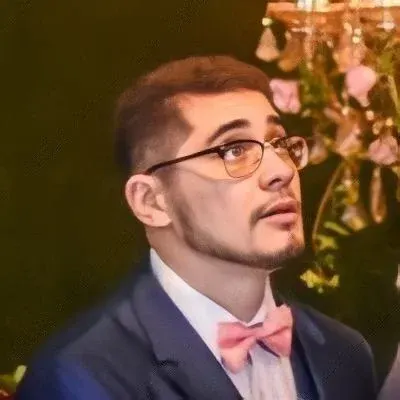

📝🔥 Remap values in pandas column with a dict, preserve NaNs 🔥📝
Have you ever found yourself needing to remap values in a pandas column using a dictionary, while still preserving any NaN values? If so, you've come to the right place! In this blog post, we'll dive into this common issue and provide you with easy and efficient solutions. Don't worry, it's not as difficult as it may seem! 😉
Let's start by understanding the context of the problem. You have a dictionary (di
) containing the mapping you want to apply to a specific column (col1
) in a pandas DataFrame. The DataFrame looks like this:
col1 col2
0 w a
1 1 2
2 2 NaN
The goal is to remap the values in col1
based on the values in di
, while preserving any NaN values:
col1 col2
0 w a
1 A 2
2 B NaN
So, how can we achieve this? 🤔
Solution 1: Using the map()
function
One straightforward way to remap values in a pandas column is by using the map()
function. This function takes a dictionary as an argument and replaces the values in the column based on the keys in the dictionary. Here's how you can do it:
# Create the dictionary
di = {1: "A", 2: "B"}
# Use the map() function to remap the values in col1
df["col1"] = df["col1"].map(di)
# Preserve NaN values
df["col1"] = df["col1"].fillna(df["col1"])
# Print the resulting DataFrame
print(df)
Output:
col1 col2
0 w a
1 A 2
2 B NaN
This solution leverages the power of pandas' map()
function and then fills any NaN values in the remapped column using the fillna()
function.
Solution 2: Using the replace()
function
Another neat approach to achieve the desired result is by using the replace()
function in pandas. This function can be used to replace specific values in a column according to a mapping dictionary. Here's an example:
# Create the dictionary
di = {1: "A", 2: "B"}
# Use the replace() function to remap the values in col1
df["col1"].replace(di, inplace=True)
# Print the resulting DataFrame
print(df)
Output:
col1 col2
0 w a
1 A 2
2 B NaN
In this solution, we directly apply the replace()
function to the col1
column, replacing the values based on the dictionary mapping.
Call-to-action: Share your experience!
Now that you know how to remap values in a pandas column with a dictionary while preserving NaN values, it's time to put your newfound knowledge into action! 🔥 Have you ever encountered any difficulties with this process? Do you have any tips or tricks to share? Let us know in the comments section below! Your insights might help fellow data scientists and pandas enthusiasts.
Keep learning, keep exploring, and keep coding! 😄✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
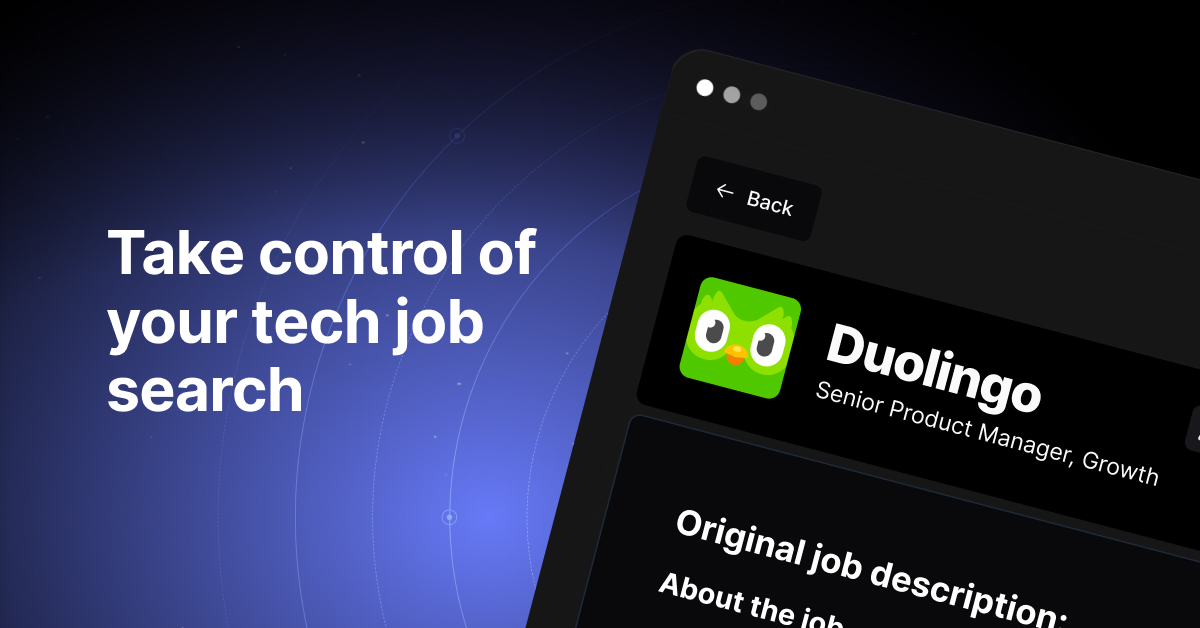