Relative imports - ModuleNotFoundError: No module named x
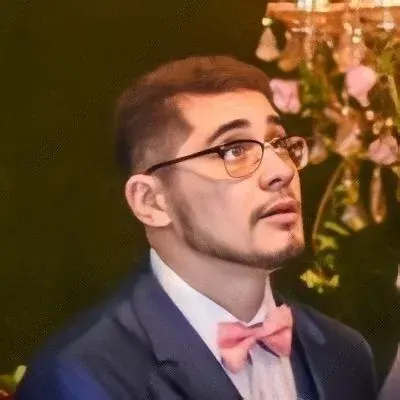
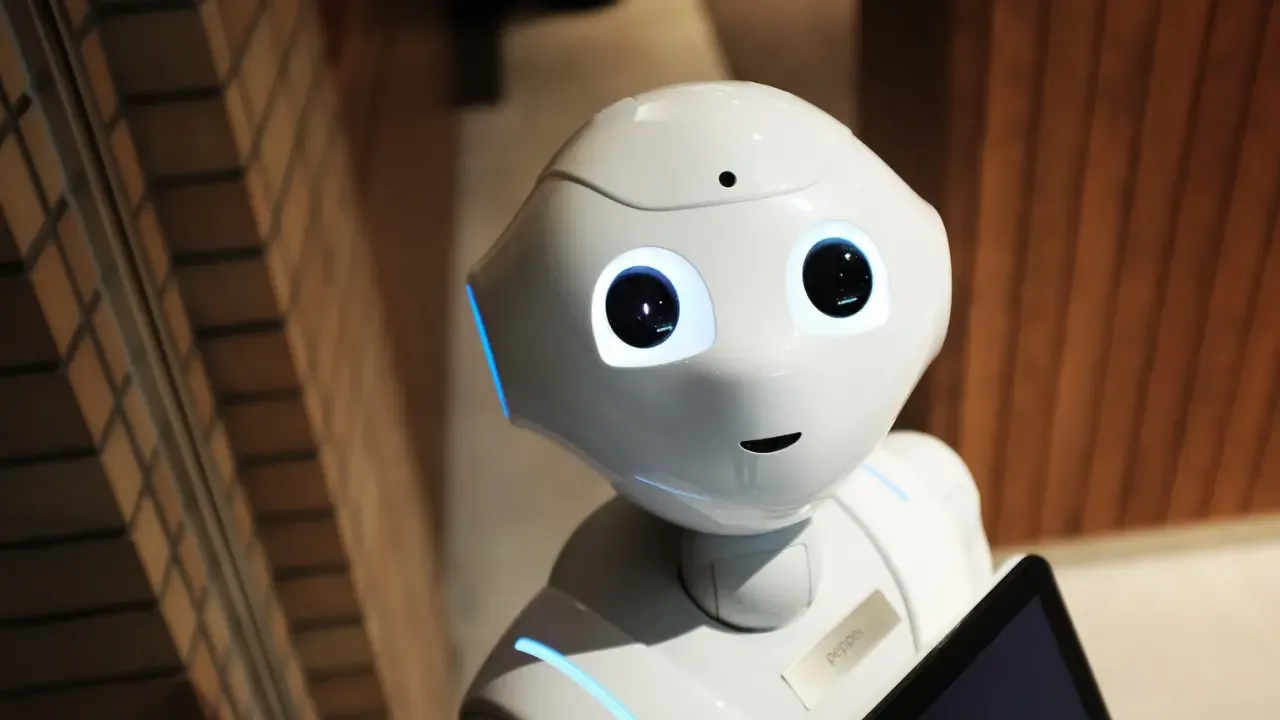
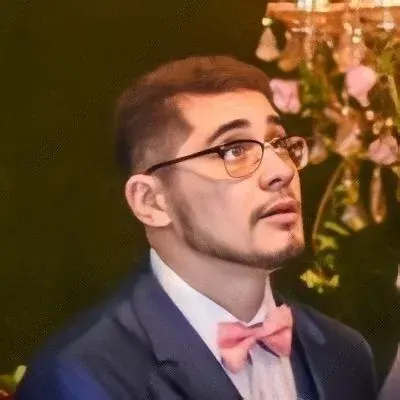
š Title: Mastering Relative Imports in Python 3: Solving the ModuleNotFoundError
š„ Introduction
Python 3 can be a bit tricky to navigate, especially if you're used to Python 2. One common issue that often arises is the dreaded ModuleNotFoundError: No module named x
error. Fear not! In this guide, we'll tackle a specific problem involving relative imports and provide easy solutions to get you back on track. So, let's dive in and conquer this challenge together! šŖ
š Understanding the Problem
The error message "ModuleNotFoundError: No module named 'config'" indicates that the Python interpreter is unable to locate the module you're trying to import. In this case, the module in question is config.py
. So, what's causing this error? Let's examine the context provided:
import config
print(config.debug)
ā ļø Potential Issues
Missing
__init__.py
: Ensure that an__init__.py
file is present in the same directory asconfig.py
andtest.py
. This file is required to treat the directory as a Python package.Incorrect Import Statement: By default, Python uses absolute imports, but attempting to import
config.py
using the statementimport config
will result in theModuleNotFoundError
. We need to use relative imports to resolve this issue.
š ļø Easy Solutions
Solution 1: Use Relative Imports
Modify your import statement in test.py
to use relative imports. Replace import config
with:
from . import config
āļøNote: The leading dot (.
) in the import statement denotes a relative import. It tells Python to look for the module in the same package/directory.
However, you might encounter another error: ImportError: cannot import name 'config'
. Don't worry; we've got a solution for this too!
Solution 2: Modify Your Project Structure
If you're facing the ImportError
mentioned above, it could be due to your project structure. Make sure you have the following structure:
project/
āāā __init__.py
āāā test.py
āāā package/
āāā __init__.py
āāā config.py
Ensure that the __init__.py
files exist in both the project
and package
directories. The package
directory contains the config.py
module.
With this adjusted structure, you can use the original import statement in your test.py
file:
import package.config
print(package.config.debug)
3ļøā£ The Importance of Troubleshooting Even with these easy solutions, you may still encounter issues. It's crucial to troubleshoot effectively to identify the root cause. Always check for:
Typos in your file and directory names
Inconsistent capitalization
Correct Python version
If the problem persists, consider posting a specific question on online forums, such as Stack Overflow, to seek assistance from the community. Sharing your code and the error message will help others understand the problem better.
š Call-to-Action Don't be discouraged by Python 3's learning curve! Embrace challenges, explore solutions, and seek support. Share this guide with others who might be struggling with relative imports, and let's build a helpful community together. š
Now, go forth and conquer those pesky ModuleNotFoundError
errors like a Python pro! šāØš
Do you have any Python error stories or solutions to share? Drop a comment below; let's discuss and learn from each other! š